Python is an object-oriented programming language that supports many data structures for storing elements such as lists, arrays, tuples, etc. Among these data structures, the two most widely used data structures are lists and arrays and they support indexing, slicing, and iterating.
This write-up will demonstrate how lists and arrays in Python differ from one another.
Understanding Python Array With Examples
A mutable data structure (which can be changed) is known as an array. It doesn’t come built-in as it needs to be imported using the array or numpy module. It stores the same type of data, such as numerical data. For instance
import array as arr
array1 = arr.array('i', [3,2,1])
print(type(array1))
for x in array1:
print(x, end=" ")
In the above code:
- The array module is imported.
- An array is initialized which consists of integers.
- The type of array is printed using the print() method.
- The array is iterated through using a for loop.
- The print method is used to display the array
The output of the above code is shown below:
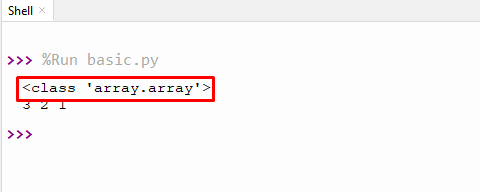
An error will be raised if we attempt to add different data types to the array. Let’s modify the above code a little bit to understand this concept better.
import array as arr
array1 = arr.array('i', [3,2,0.01])
print(type(array1))
for x in array1:
print(x, end=" ")
In the above code:
Everything is kept the same as in the previous example except for the addition of a float number in the array.
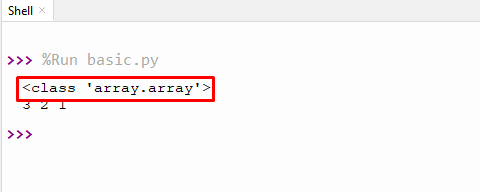
It can be seen that the program shows an error because it was expecting an integer number and got a float number.
Understanding Python List With Examples
A list is also referred to as a mutable data structure in Python. It comes built-in in Python and can store data of multiple types, which can be categorical as well as numerical. For instance,
List = ['John', 23, True, 0.01]
print(type(List))
print(List)
In the above code:
- A list is initialized consisting of multiple data types such as string, integer, boolean, and float number.
- The type of list is printed using the type() method.
- The list is displayed using the print method
The output of the above code is as follows:
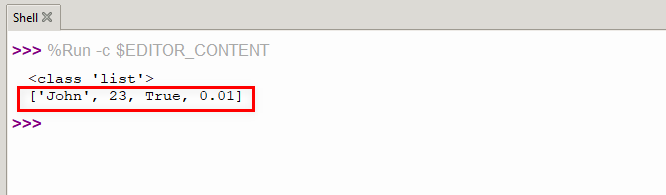
Key Differences Between Arrays and Lists In Python
The table below highlights some of the major differences between arrays and lists in Python:
Lists | Arrays |
---|---|
It is an in-built data structure in Python. | Not in-built. It needs to be imported using an array or numpy module. |
A list can include elements with the same data type as well as elements with distinct data types. | An array comprises/consists of elements of the same data type. |
Mathematical/arithmetic operations cannot be handled by a list | An array can handle mathematical operations easily. |
The list provides more flexibility of data. | An array is not as much flexible as a list |
The list consumes more RAM(Random Access Memory) as compared to Arrays. | An array consumes less RAM. |
Lists are enclosed using square brackets. | Arrays are enclosed using the .arr function. |
The list is favorable to a shorter sequence of data. | An array is favorable to a larger sequence of data. |
A list can be accessed without loops using iloc and loc methods. | An array is commonly accessed using loops. |
Conclusion
Arrays and lists are referred to as mutable data structures in Python. Unlike arrays, a list is a built-in data structure in Python. An array can store data of the same type, such as numerical data whereas a list can store multiple types of data such as strings, integers, boolean, and float numbers. This write-up briefly discussed the difference between arrays and lists.