PyTorch is a machine-learning library that offers various modules to work with images. The “torchvision.transforms” module from the PyTorch package has a collection of classes and functions to perform various transformations on images including cropping, resizing, rotating, flipping, scaling, etc. Sometimes, users may want to flip the specific image. They can use the “RandomHorizontalFlip()” to flip any image at a random angle with the specified probability.
This blog will illustrate the method to flip an image in PyTorch.
How to Flip an Image Horizontally in PyTorch?
To flip a particular image horizontally in PyTorch, check out the given-provided steps:
- Upload/add an image to Google Colab
- Install necessary libraries
- Read uploaded input image
- Define a transform through the “RandomHorizontalFlip(p=1)” method
- Apply the transform on the image
- Display horizontally flipped image
Step 1: Upload/Add an Image to Google Colab
First, users are required to open Google Colab and upload a specific image from the computer to it. Tap on the following icons to do so:
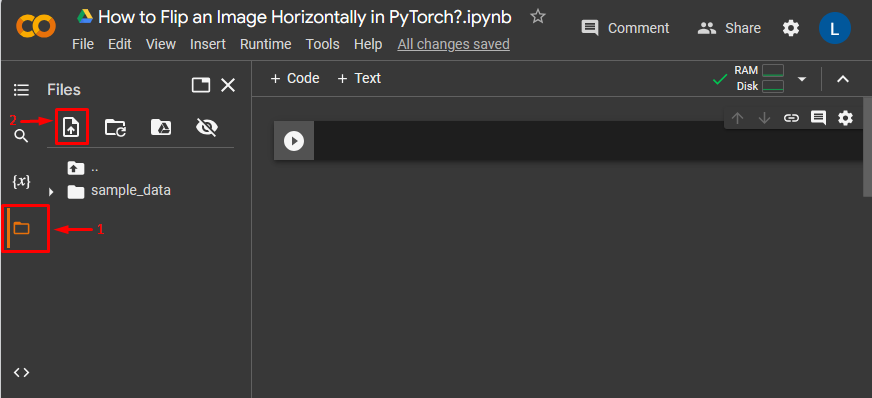
This has uploaded the image to Google Colab as seen below:
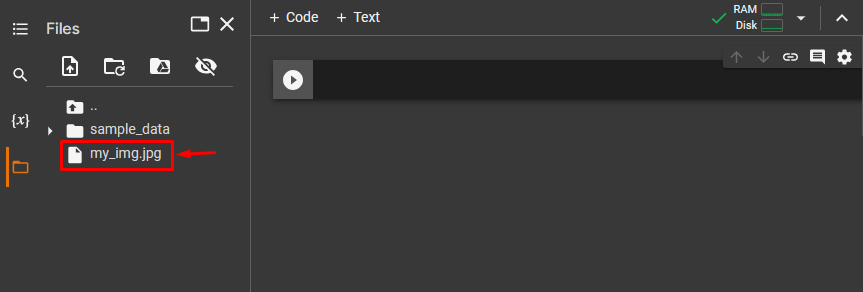
Below is the image that we have uploaded/added to flip horizontally:
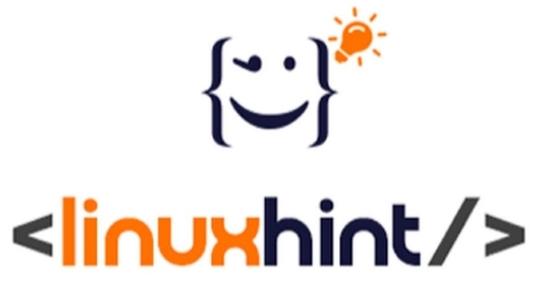
Step 2: Install Necessary Libraries
Next, install the following libraries that are essential for performing operations on images:
import torch, torchvision.transforms as T
from PIL import Image
In the above snippet:
- “import torch” imports PyTorch package.
- “import torchvision.transforms as T” imports the “transforms” module as a “T” to preprocess image data.
- “from PIL import Image” is utilized for opening and saving various image file formats:

Step 3: Read the Uplaoded Input Image
After that, use the “Image.open()” function to read the uploaded image i.e. “my_img.jpg” from the computer:
input_img = Image.open('my_img.jpg')

Step 4: Define a Transform
Now, use the “RandomHorizontalFlip(p=1)” method to define a transform to flip the provided input image horizontally:
transform = T.RandomHorizontalFlip(p=1)

Step 5: Apply the Transform on Input Image
Then, apply the defined transform on the uploaded input image:
flipped_img = transform(input_img)

Step 6: Display Horizontally Flipped Image
Finally, print the horizontally flipped image:
flipped_img
In the below output, it can be observed that the input image has been successfully flipped horizontally:
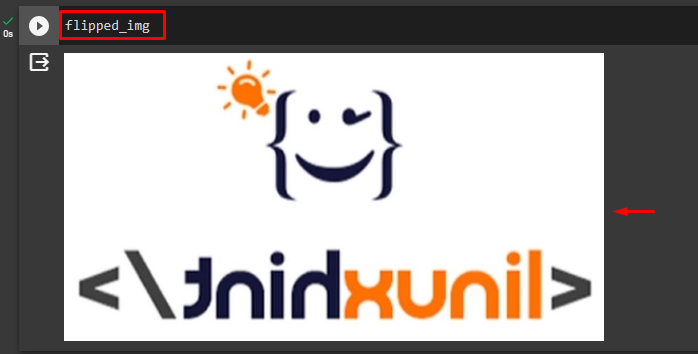
Comparison
The below table shows the comparison between the original image and the horizontally flipped image:
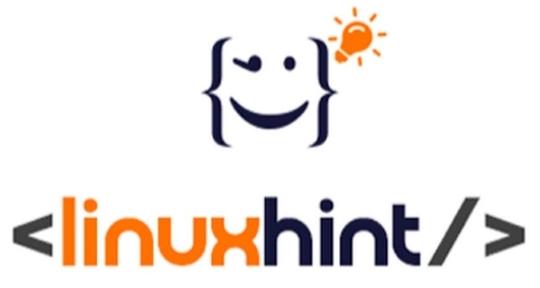
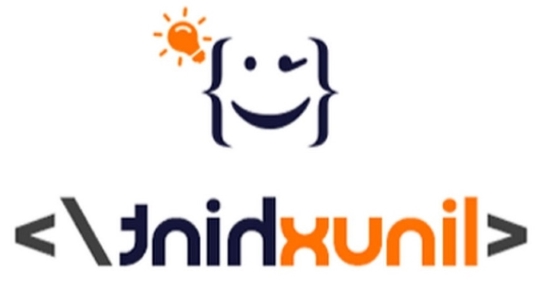
We have explained the efficient method of flipping an image horizontally in PyTorch.
Note: Click on the provided link to access our Google Colab Notebook.
Conclusion
To flip a particular image horizontally in PyTorch, first, upload/add an image to Google Colab. Next, install the required libraries and read the uploaded input image. Then, use the “RandomHorizontalFlip(p=1)” method to define and apply the transform to the input image. Finally, display the horizontally flipped image. This blog has illustrated the method to flip an image in PyTorch.