The String in Java can be generated either by the user or the System. The Strings hold some values that are either provided by some assigned method or can be entered on a random basis. These random value-holding Strings can be alphabetic or alphanumeric according to the needs. The user can either generate these random String manually for once or can use default methods to generate different random String on each execution.
This guide explains the procedure to generate a random String in Java.
How to Generate a Random String in Java?
Multiple approaches can be used to generate a random alphabetic or alphanumeric type String in Java. These approaches are mentioned below:
- Method 1: Generate a Random String Using Random Class in Java
- Method 2: Generate a Random String Via Java 8 API Stream
- Method 3: Generate a Random String Using Regular Expression
- Method 4: Generate a Random String Using Apache Commons
- Method 5: Generate a Random String Using “Math.random()” Method
Method 1: Generate a Random String Using Random Class in Java
The usage of the “Random” class for the generation of random String is the most optimized approach. This class is part of the “java.util” package and it provides many methods to generate random bounded or unbounded Strings. Take a look at the below examples to create a random bounded and unbounded String in Java.
Example 1: Generation of Random Unbounded String
The “unbounded” Strings contain a mixture of alphabetic, numerical, and symbol characters. Its random generation of this String is shown below:
import java.util.Random;
class javabeat
{
public static void main(String args[])
{
byte[] strArr = new byte[5];
new Random().nextBytes(strArr);
String genRanStr = new String(strArr);
System.out.println("The Generated Random Un-Bounded String is:" + genRanStr);
}
}
Explanation of the above code is as follows:
- First, the required “java.util.Random” package is imported and a class named “javabeat” is created.
- Inside the class, the “main()” function is defined.
- Next, the array having the type of “byte” is created with the length of “5” and the name of “strArr” is assigned to the created array.
- Then, invoke the “Random” class constructor and apply the “nextBytes()” methods having the “strArr” as a parameter. This will generate an unbounded random String and store that String in the created “strArr” array.
- After that, store the byte “strArr” array value in the “genRanStr” named String which is then displayed on the console.
The output shows that the random unbounded String has been generated:
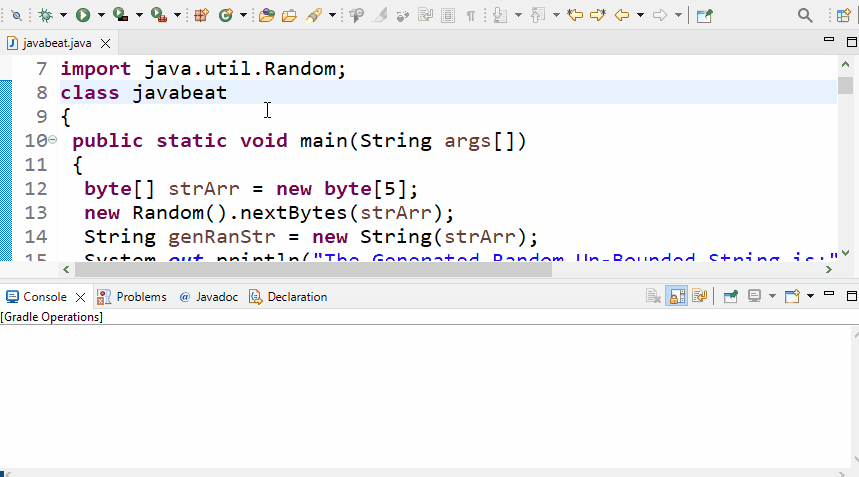
Example 2: Generation of Random Bounded String
In this example, the “Random” class is used to generate random Strings within the provided bound or range. This will restrict the “Random” class to generate unique random Strings while remaining inside the range. For instance, the range of all alphabetic characters in uppercase is set in below below-mentioned code:
import java.util.Random;
class javabeat
{
public static void main(String args[])
{
int startRange = 65;
int endRange = 90;
int randomStrLen = 15;
Random randomizer = new Random();
StringBuilder bufferSpace = new StringBuilder(randomStrLen);
for (int k = 0; k < randomStrLen; k++) {
int stringRangeChecker = startRange + (int)(randomizer.nextFloat() * (endRange - startRange + 1));
bufferSpace.append((char) stringRangeChecker);
}
String generatedString = bufferSpace.toString();
System.out.println("The Generated Random Bounded String is:" + generatedString);
}
}
The explanation of the above code snippet is written below:
- First, import the required “java.util.Random” package and create a new class named “javabeat”.
- Next, declare two integer type variables named “startRange” and “endRange”. Then, assign them the starting and ending characters in ASCII code format. In our case, the ASCII code for starting(A) and ending(Z) uppercase characters are “65” and “90”.
- In addition, declare and initialize an “int” type variable “randomStrLen” that contains the length of a random String that needs to be generated.
- Now, create a new object of the “Random” class named “randomizer”. Pass this object as a parameter for the “StringBuilder” constructor and create a “bufferSpace” object.
- After that, utilize the “for” loop that iterates till the value of a “randomStrLen” variable. It generates a random String while remaining inside the provided range.
- In addition, the generated “int” type String is converted into “char” using type casting and appends to the “bufferSpace” String builder object.
- Finally, this “bufferSpace” is converted into a String via the “toString()” method, and the result is then displayed on the console.
The output confirms the generation of bounded random String:
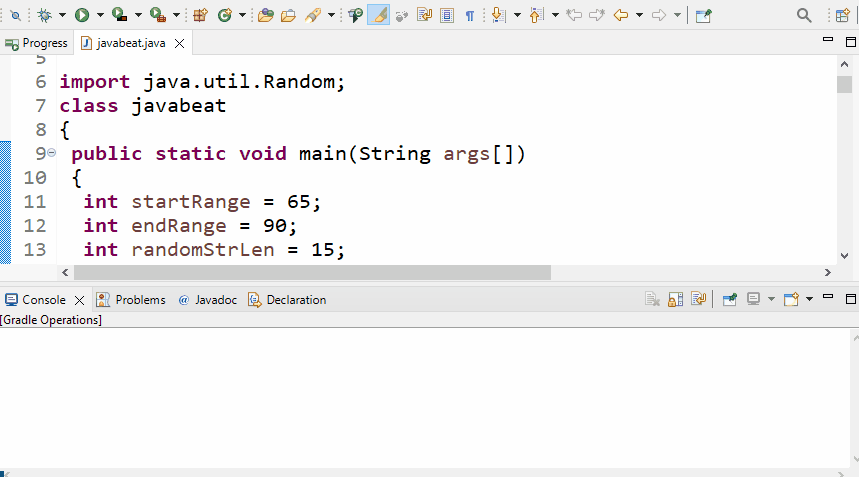
Method 2: Generate a Random String Via Java 8 API Stream
The Java 8 API stream offers three methods to generate the random Strings according to the type of String stream data. These methods are “ints()”, “longs()”, and “doubles()” and they are applied over the “intStream”, “LongStream”, and “DoubleStream” respectively.
Let’s have a couple of examples to generate random Strings using the Java 8 API Stream methods.
Example 1: Generation of Alphanumeric String Via Java 8
In this case, the Java 8 API Stream provided method “ints()” is used to generate a simple random String. Then, the “filter()” method is attached to select only numeric and alphabetic characters from the provided range. This leads to the generation of an alphanumeric random String:
import java.util.Random;
class javabeat
{
static String randomGenerator(int startRange, int endRange, int randomStrLen) {
Random randomObj = new Random();
String endString = randomObj.ints(startRange, endRange + 1)
.filter(codepoint -> (codepoint <= 57 || codepoint >= 65) && (codepoint <= 90 || codepoint >= 97))
.limit(randomStrLen)
.collect(StringBuilder::new, StringBuilder::appendCodePoint, StringBuilder::append)
.toString();
return endString;
}
public static void main(String args[])
{
int startRange = 48;
int endRange = 122;
int randomStrLen = 12;
String result = randomGenerator(startRange, endRange, randomStrLen);
System.out.println("\nThe Generated Random Alphanumeric String is:" + result);
}
}
In the above code block:
- First, import the “java.util.Random” package and create a custom class named “javabeat”. Also, define a custom function “randomGenerator()” that accepts three “int” type parameters.
- Next, create an object “randomObj” of the “Random” class and pass the starting and ending range points to the “ints()” method.
- These points are the received parameters and they store the range of characters that can be inserted in the random String.
- Attach the “filter()” method to select only specific parts of characters from the provided range.
- In addition, apply the “limit()” method to restrict the number of generated characters for String. The “collect()” method is also used to append the characters in a single String.
- In the end, store the generated String in the “endString” variable and return it from the function using the “return” keyword.
- After that, define a “main()” method which contains the values of starting and ending range and the String length. These values are stored in variables which are passed as an argument for the “randomGenerator()” function.
- Display the returned random generated String by this method on the console.
The output confirms that the alphanumeric random String has been generated:
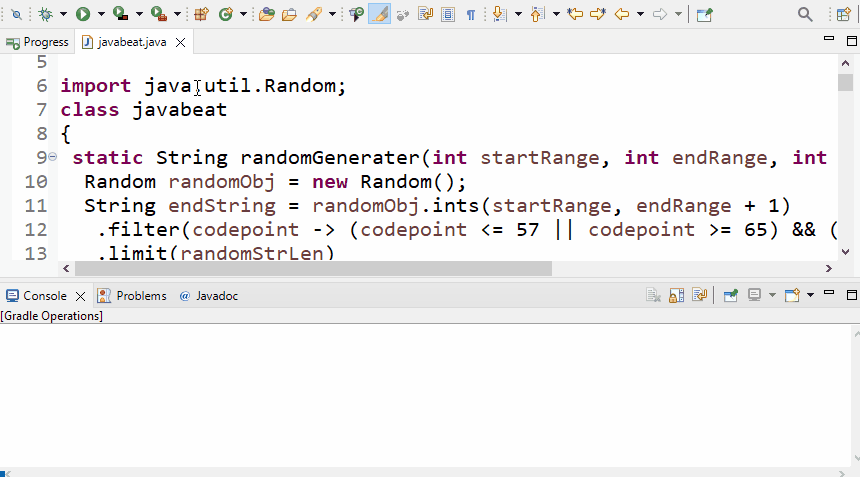
Example 2: Generation of Alphabetic String Via Java 8
In this example, the “Random.ints()” Java 8 API Stream method is used to generate a unique alphabetic random String. This method uses the ASCII codepoints to select the alphabetic characters and then use them to generate the required String:
import java.util.Random;
class javabeat
{
//Creating custom function
static void randomGenerator(int startRange, int endRange, int randomStrLen) {
Random randomizer = new Random();
String generatedString = randomizer.ints(startRange, endRange + 1)
.limit(randomStrLen)
.collect(StringBuilder::new, StringBuilder::appendCodePoint, StringBuilder::append)
.toString();
System.out.println("\nThe Generated Random Alphabetic String is:" + generatedString);
}
//Defining the main() method
public static void main(String args[])
{
int startRange = 65;
int endRange = 90;
int randomStrLen = 15;
randomGenerator(startRange, endRange, randomStrLen);
}
}
The above code works like this:
- First, import the “java.util.Random” package and create a class of “javabeat” which contains a “main()” method.
- Next, create a custom function “randomGenerator” that accepts three integer-type parameters. These parameters store the starting and ending range of characters and the length of a random String.
- Inside the function, create an object “randomizer” for the “Random” class. Using this object invoke its “ints()” method and pass the starting and ending range as its parameter.
- The “limit()” method is also attached to restrict the size of a generated String to the specified limit length.
- Moreover, define a “StringBuilder” object and insert the random String characters init. After converting the provided integer codepoints into their corresponding encoding representation.
- In addition, convert stored literals into a String using the “toString()” method and store it in the “generatedString” variable.
- In the end, define a “main()” method that contains three “int” type variables storing values for initial, ending, and length for random String.
- These variables are then passed as an argument in the custom-created “randomGenerator()” function.
The output confirms that a unique alphabetic random String has been generated after each execution cycle:
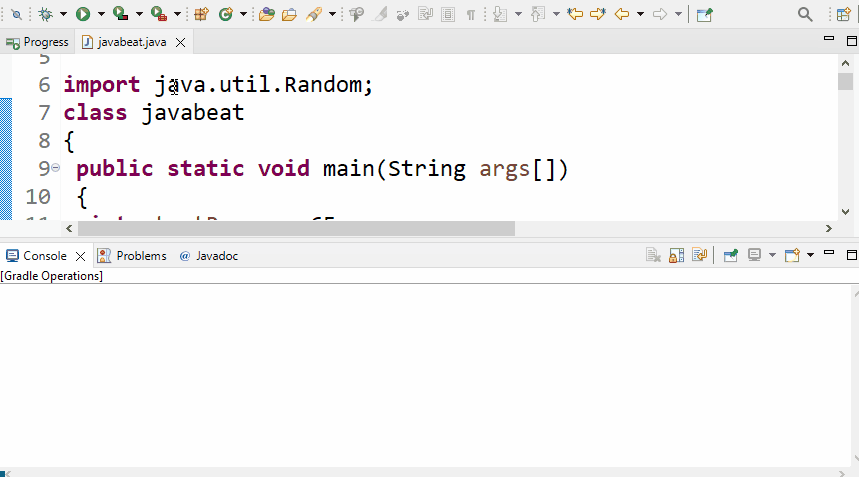
Method 3: Generate a Random String Using Regular Expression
The custom regular expression can be used with the “Random” class to generate a random String of specific characters. The regular expression method gives more freedom to choose the type of characters users want to enter in their random String:
import java.util.Random;
import java.nio.charset.Charset;
class javabeat {
static String randomizer(int strSize)
{
byte[] tempArray = new byte[255];
new Random().nextBytes(tempArray);
String entireRanStr = new String(tempArray, Charset.forName("UTF-8"));
StringBuffer bufferSpace = new StringBuffer();
String ranString = entireRanStr.replaceAll("[^A-Za-z0-9]", "");
for (int k = 0; k < ranString.length(); k++) {
if (Character.isLetter(ranString.charAt(k)) && (strSize > 0) || Character.isDigit(ranString.charAt(k)) && (strSize > 0))
{
bufferSpace.append(ranString.charAt(k));
strSize--;
}
}
return bufferSpace.toString();
}
public static void main(String[] args)
{
int strSize = 12;
System.out.println(randomizer(strSize));
}
}
In the above code snippet:
- First, import the “Random” and “nio.charset.Charset” java packages using the “import” keyword. Also, define a “randomizer()” method that accepts the single parameter of “strSize” inside the custom class named “javabeat”.
- Next, declare a byte array “tempArray” with a size of “255”. Insert random elements by applying the “nextBytes()” method with the “Random” class.
- Convert the byte array into a String named “entireRanStr” having the charset of “UTF-8”. Also, create an instance named “bufferSpace” for the “StringBuffer” class.
- Then, apply the “replaceAll()” method over the “entireRanStr” String to remove all special characters and store the resultant result in the “RanString” variable.
- After that, apply the “for” loop that iterates till the length of “ranString”. This loop contains an “if” statement that appends the characters of “ranString”. After checking if that selected character of String is a “Letter” or “digit” while confirming that the value of “strSize” is greater than “0”.
- Once the condition gets “true”, the selected character gets appended to the “bufferSpace” object, and the value of “strSize” gets reduced by a factor of “1”.
- Outside the “for” loop, return the “bufferSpace” after passing through the “toString()” method.
- Finally, initialize a “main()” method that contains the length of a String stored in the “strSize” variable.
- This variable is then passed as the parameter for the “strSize” function and the returned value is displayed on the console.
The output confirms the generation of an alphanumeric String using the “Regular Expression” approach:
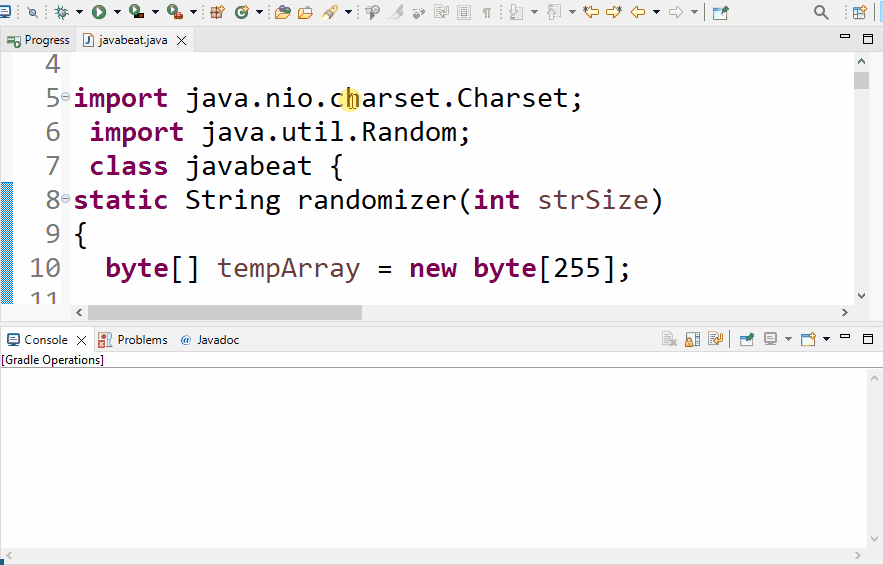
Method 4: Generate a Random String Using Apache Commons
The “Apache Commons” acts as a library host by providing all sorts of methods for various purposes. The provided methods are more optimized, easy to understand, and contain meaningful names. Moreover, the user can either use the provided methods or even modify the working of methods by assigning custom values to the parameters.
For a better understanding and practical demonstration have a look at the below example.
Prerequisite
If the “Apache Commons” library is already installed and added to the current Java project, then move directly to the below example section. In case, the “Apache Commons” is not installed then follow the mentioned steps.
Step 1: Download the Apache Commons
Traverse to the “Apache Commons Documentation” and scroll down to the “Source” section. From there hit the “tar” setup version for the “apache-commons-lang” to download the required tar file:
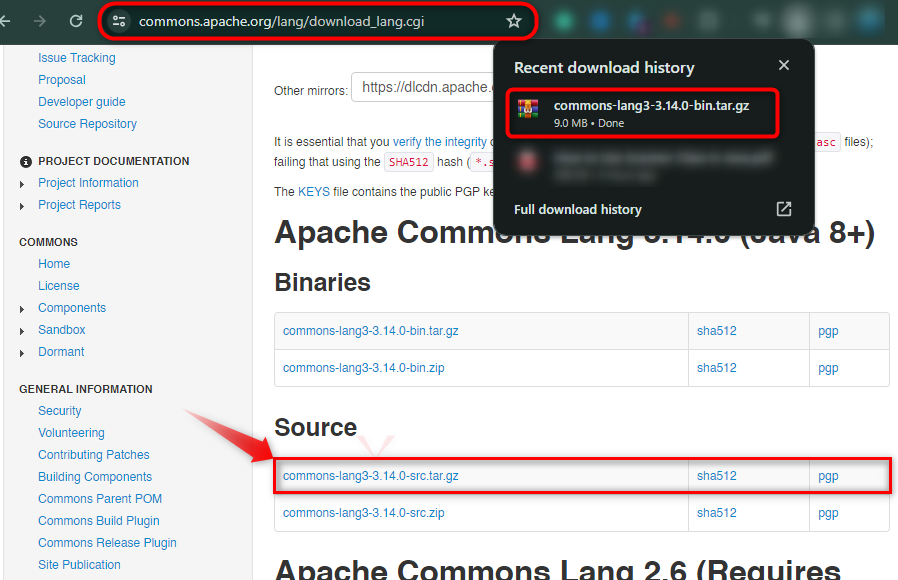
Step 2: Modify the “jar” File Location
Now, extract the downloaded commons-lang “tar” file. From the extracted files select and copy the single “commons-lang3-<versionName>.jar” file:
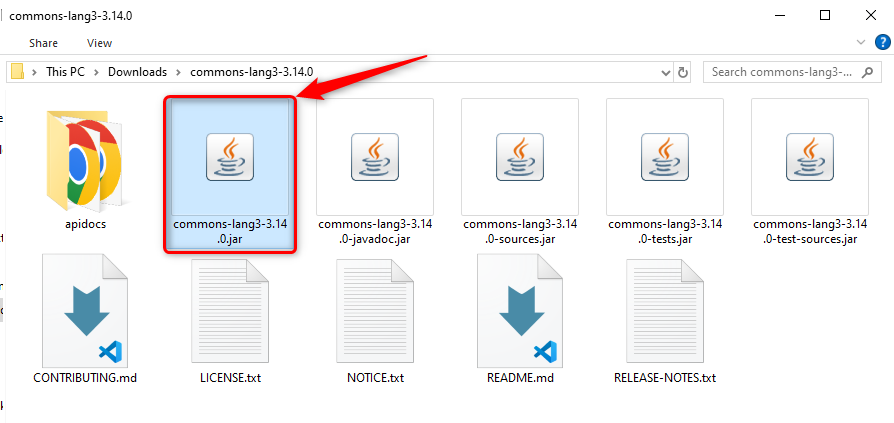
Place the copied file into the “lib” folder of desired Java project as shown below:
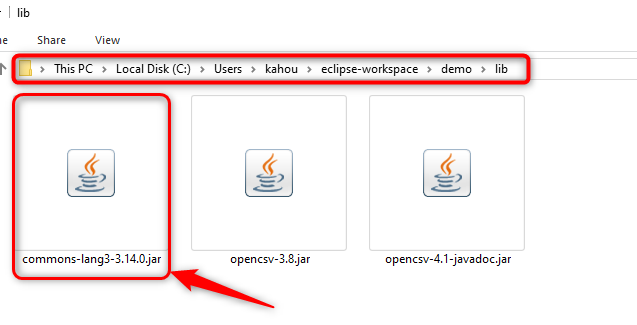
After placement, your project folder structure appears like this in eclipse IDE:
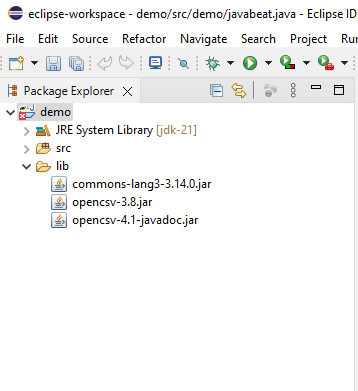
Step 2: Setting up Build Path for Apache Commons Lang
Select the current Java project folder and press the “Alt” and “ENTER” keys at once to open the project “Properties” dialog box. The same action can also be performed by “Right Clicking” the mouse over the project folder and hitting the “Properties” option from the appeared list:
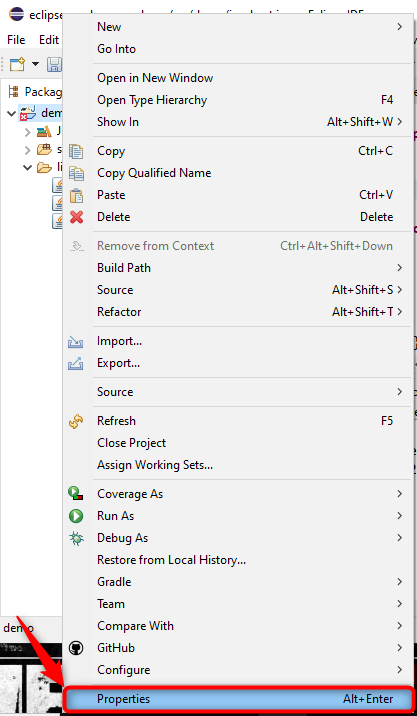
Now, select the “Java Build Path” option from the appeared dialog box and move to the “Libraries” tab. Then, select the “Classpath” and hit the “Add JARs” button to include custom JAR files in the opened project:
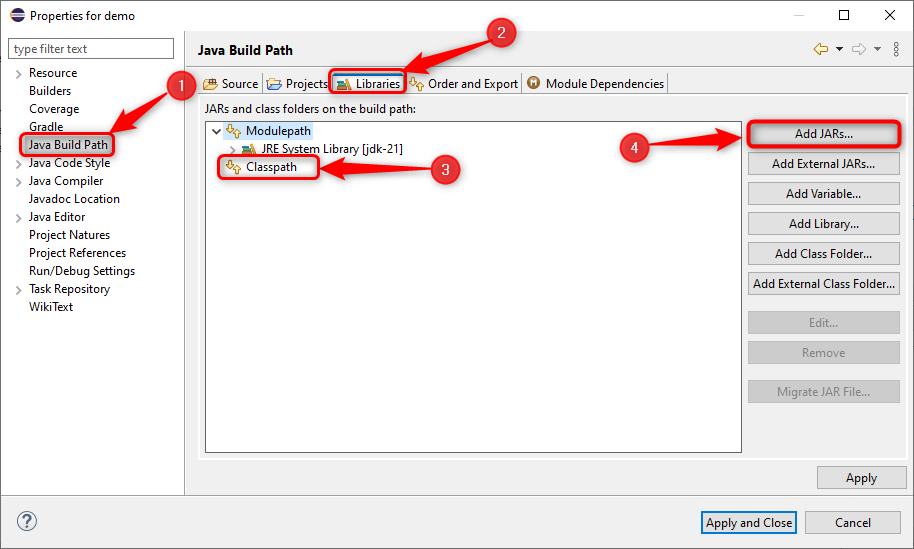
After hitting the mentioned button, the dialog box of “JAR Selection” appears. From there, select the downloaded “commons-lang3-<versionName>.jar” file and hit the “OK” button:
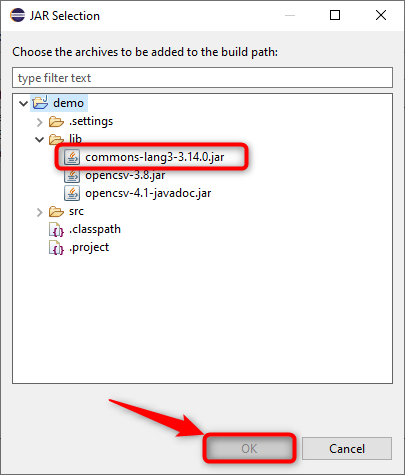
The selected file appears like this on the “Java Build Path” dialog box. Finally, press the “Apply and Close” button to save the performed modification and you’re good to go:
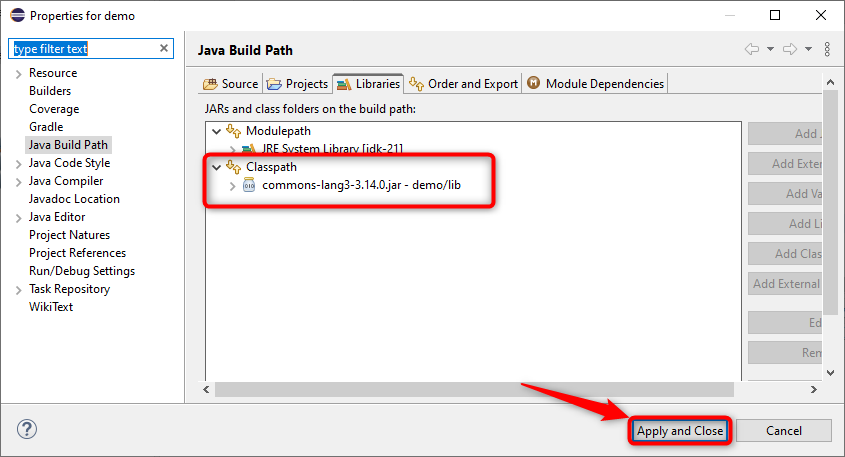
Example: Use of Apache Commons Lang to Generate Alphabetic, Alphanumeric, and Bounded Random String:
In this example, the methods provided by the “Apache Commons Lang” library are used to generate random Strings. The Random Strings that are going to be generated are “Alphabetic”, “Alphanumeric”, and “Bounded”.
The methods for generating “Alphabetic” and “Alphanumeric” random strings are “randomAlphabetic()” and “randomAlphanumeric()”. For custom type Strings that contain only “Alphabet” or “Numbers” or their combination, the “random()” method is used along with its parameters. For instance, have a look at below- lines of code:
import org.apache.commons.lang3.RandomStringUtils;
class javabeat {
public static void main(String args[])
{
//Generating Random Alphabetic String
String alphaRanStr = RandomStringUtils.randomAlphabetic(8);
System.out.println("\nThe generated Alphabetic String is: '" + alphaRanStr + "'.");
//Generating Random Alphanumeric String
String alphanumRanStr = RandomStringUtils.randomAlphanumeric(8);
System.out.println("\nThe generated Alphanumeric String is: '" + alphanumRanStr + "'.");
//Generating Random Bounded String
int len = 8;
boolean useOfChar = false;
boolean useOfNum = true;
String boundedStr = RandomStringUtils.random(len, useOfChar, useOfNum);
System.out.println("\nThe generated Bounded String is: '" + boundedStr + "'.");
}
}
The working of above code is described below:
- First, use the “import” keyword to import the “RandomStringUtils” package from the “apache.commons.lang3” library.
- Create a class “javabeat” which contains a “main()” method.
- Now, generate a random “Alphabetic” String by invoking the “RandomStringUtils.randomAlphabetic()” method. The length of a String that is required to be generated is passed as a parameter to the mentioned method.
- Store and display the generated result in an “alphaRanStr” variable and over the console respectively.
- For a random “Alphanumeric” String, invoke the “RandomStringUtils.randomAlphanumeric()” method. Pass the String length as a parameter and display the generated String on the console window.
- After that, declare an “int” type variable “len” and initialize it with the length of a required random String. Declare two “boolean” type variables as well namely “useOfChar” and “useOfNum”. Assign them the values of “false” and “true” respectively or you can assign them values according to your requirements.
- Finally, pass all three variables as an argument in the “RandomStringUtils.random()” method.
- The resultant String is stored in the “boundedStr” variable and displayed on the console window.
The generated output shows the random String of a specified type:
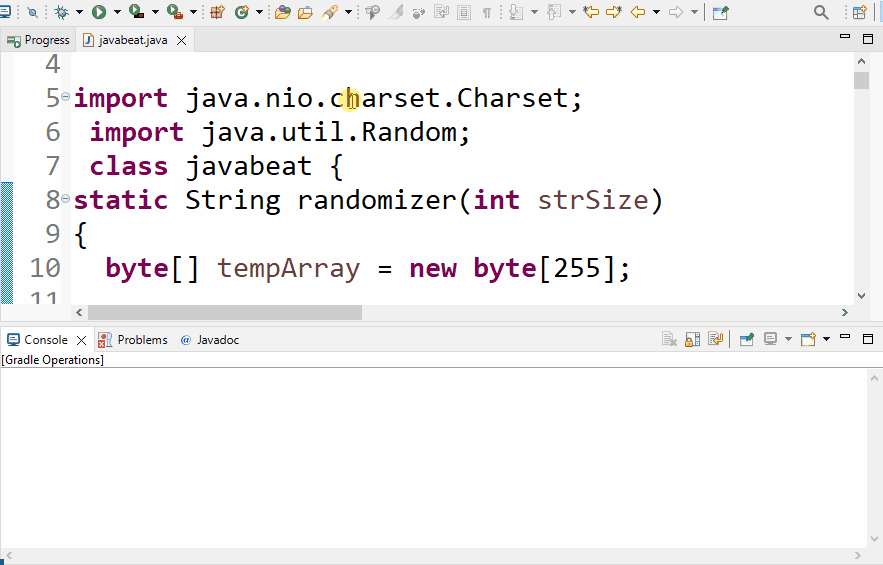
Method 5: Generate a Random String Using “Math.random()” Method
The “Math.random()” method is one of the most popular methods for the generation of random numbers. This method deals with only numbers or can be altered to select the indexing of an array or String indexing. This approach of the “Math.random()” method is used to select characters randomly for the generation of a random String:
class javabeat {
static String RandGenerator(int size)
{
String allowedChars = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvxyz";
// creating a StringBuffer size of AlphaNumericStr
StringBuilder bufferSpace = new StringBuilder(size);
int x;
for (x = 0; x < size; x++) {
int ch = (int)(allowedChars.length() * Math.random());
//adding Random character one by one at the end of s
bufferSpace.append(allowedChars.charAt(ch));
}
return bufferSpace.toString();
}
public static void main(String[] args) {
int len = 10;
System.out.println("The generated random string of " + len + " characters is: " + RandGenerator(len));
}
The working of the above code is explained as follows:
- First, create a custom function named “randGenerator()” that accepts a single “int” type parameter having the name of “size”.
- Next, create a String “allowedChars” and assign all characters that can be added to the generated random String.
- After that, create an instance “bufferSpace” of the “StringBuilder” class having the length of a provided parametric “size” variable.
- Then, utilize the “for” loop that iterates till the “size” value. It selects the characters index randomly via the “Math.random()” method and typecasts the selected one into the “int” type.
- The “charAt()” method selects the “allowedChars” String characters via a randomly selected index parameter. The selected characters are then added into the “bufferSpace” using the “append()” method.
- Return this “bufferSpace” instance that stores the randomly generated String.
- Finally, define a “main()” method that invokes the “RandGenerator()” method and pass the length as an argument.
The output confirms the generation of a random String in Java:
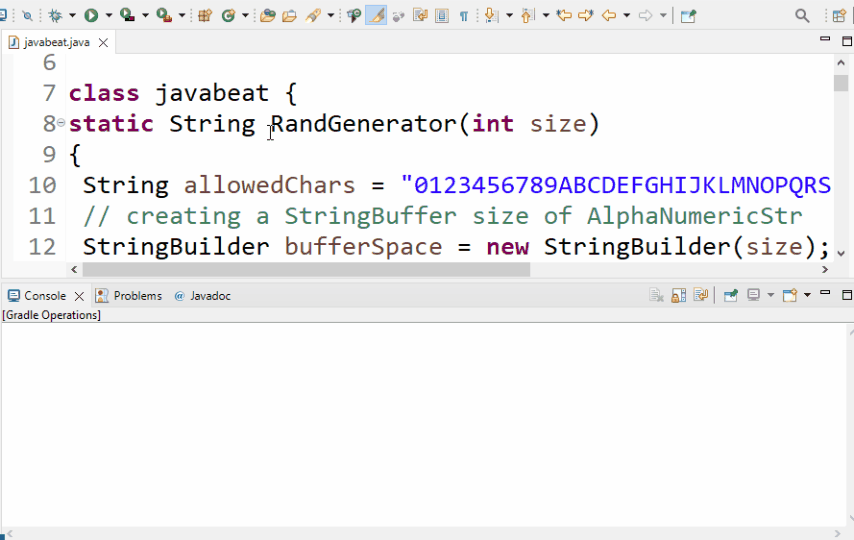
That’s all about the generation of a random String in Java.
Conclusion
To generate a random String in Java, using the “Random” class, Java 8 API stream method of “ints()”, “longs()”, or “doubles()”. The custom build “Regular Expression” can also be used with the combination logical operators and “if” statements. The “Apache Commons” library is also used with its predefined methods of “randomAlphabetic()”, “random()” and “randomAlphanumeric()”. Moreover, the “Math.random()” is also altered to generate the custom string by working with the allowed characters String or array index. This guide has explained the working of the generation of a random String in Java.