The “Map” is a collection type that stores the data in key-value pair format and the key assigned to each element value needs to be unique. The elements get added, deleted, accessed, or removed according to its assigned “Key”. The Map is created using “HashMap” and they both offer the same functionality of storing data in key-value pairs. However, the work or rules they both follow are very different from each other. This difference between “HashMap” with “Map” is mentioned as:
HashMap | Map |
---|---|
HashMap does not follow any order for its elements. | Strictly follow the insertion order for their elements. |
It is quicker than Map when it comes to performing operations over the elements. | Due to insertion order, the Map is comparatively slower. |
HashMap can be used to implement the traditional Map interface. | Map by itself is an interface, which is implemented using “HashMap”, “TreeMap”, and many others. |
The user can store more than one null key-value pair in HashMap. | It can not hold any “null” value or key. |
HashMap can contain Duplicate Values as well. | The duplication of values is not allowed, each element must be unique. |
This guide explains the approaches to display the HashMap in Java.
How to Print HashMap in Java?
Java offers multiple methods to select and print the elements of the HashMap. These methods are mentioned and described below with proper implementation:
- Method 1: Print HashMap Using “System.out.println()” Method
- Method 2: Print HashMap Using “entrySet()” Method and “forEach” Loop
- Method 3: Printing User Defined HashMap Using “keySet()” Method
- Method 4: Printing HashMap Using “Arrays.asList()” Method
- Method 5: Printing HashMap Using “values()” Method
- Method 6: Printing HashMap Using “BiConsumer” Interface
- Method 7: Printing HashMap Using “singletonList()” Method
Let’s dive into the mentioned method to print HashMap. Let’s first create a dummy or targeted “HashMap” that is going to be retrieved, the code for the creation is shown below.
Method 1: Print HashMap Using “System.out.println()” Method
The “System.out.println()” method is the most basic and straightforward method to display any “collection” type or “text” over the console. If there is duplication then the duplicate will be printed only at once. In our case scenario, the Set is passed as an argument in the “System.out.println()” method to display its elements:
import java.util.*;
public class csvReading {
public static void main(String[] args) {
Map<Double, String> demoMap = new HashMap<>();
demoMap.put(1.4, "Jackson");
demoMap.put(2.2, "Alen");
demoMap.put(3.5, "Jane");
System.out.println("\nThe Map Contains Following Elements: \n\n" + demoMap);
}
}
The explanation of stated code is as follows:
- First, all methods or classes of the “java.util” package are imported using the “import” keyword.
- Next, the “HashMap” with the name “demoMap” having the Key and Value pair of “Double” and “String” data type is declared.
- After that, the values in the “Key-value” pair format are inserted in the “demoMap” using the “put()” method.
- Finally, pass the “demoMap” as an argument in the “System.out.println()” method to print the elements on the console.
The output shows that HashMap elements have printed on the console:
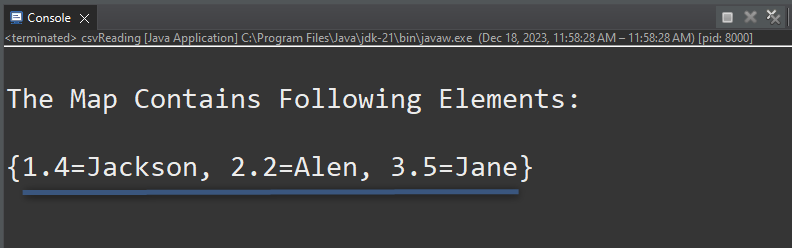
Method 2: Print HashMap Using “entrySet()” Method and “forEach” Loop
The “entrySet()” method is used with the Map to print the internal mapping that occurred between the Map “Keys” and “Values”. This allows the user to see the internal workings and gather information about the values corresponding to each key:
import java.util.*;
public class csvReading {
public static void main(String[] args) {
Map<Double, String> demoMap = new HashMap<>();
demoMap.put(1.4, "Jackson");
demoMap.put(2.2, "Alen");
demoMap.put(3.5, null);
System.out.println("\nThe Map Contains Following Elements: \n");
//Using the Combination of entrySet Method and forEach loop
demoMap.entrySet().forEach(System.out::println);
}
}
The description of above code is written as:
- First, the map named “demoMap” is created and random values are inserted in it, using the “put()” method.
- Next, the “entrySet()” method is applied over the “demoMap” to retrieve the internal mapping of key-value pairs.
- After that, the “forEach()” method is utilized to display each key value map entry over the console.log.
The output confirms that internal mapping has been displayed on the console:
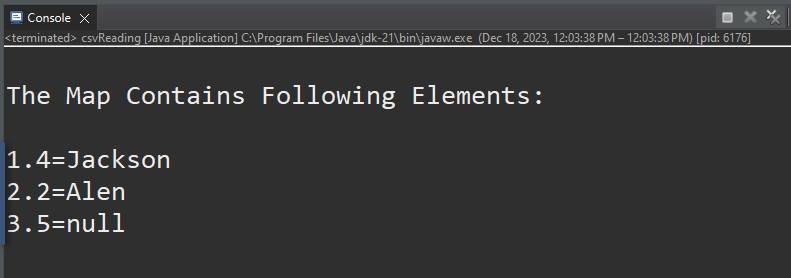
Method 3: Printing User Defined HashMap Using “keySet()” Method
The “keySet()” method selects the items stored in Map by accessing their corresponding “Keys”. The retrieved keys are then passed in the enhanced “for” loop to display each Map item on the console. In the below example, the HashMap is created according to the user-defined data at run time. Then, the entered data in HashMap is retrieved using the “keySet()” method:
import java.util.*;
public class csvReading {
public static void main(String[] args) {
Map<Double, String> demoMap = new HashMap<>();
//Setting And Displaying The Map Length
System.out.println("\nEnter Map Length: ");
Scanner userInput = new Scanner(System.in);
int mapSize = userInput.nextInt();
System.out.println("\nThe Entered Map Length is: \t: " + mapSize);
//Getting and Setting Key Value Pairs for Map.
for(double i = 0; i<mapSize; i++)
{
System.out.println("\nEnter 'Key' for Map in double: ");
double mapKey = userInput.nextDouble();
System.out.println("\nEnter Map 'Value' in String: ");
String mapValue = userInput.next();
demoMap.put(mapKey, mapValue);
}
//Displaying the Map Values
System.out.println("\nThe Map Contains Following Key value pairs: \n");
for (Double mapKey: demoMap.keySet()){
System.out.println(mapKey +" = "+demoMap.get(mapKey));
}
}
}
In the above code snippet:
- First, declare a “Map” named “demoMap” with the datatype of “Double” and “String” for the Key and Value pair respectively.
- Next, create a new instance of the “Scanner” class named “userInput” which gets a single integer type value from the user. The retrieved value is stored in the “mapSize” named variable.
- Then, use the “for” loop that starts from “0” till the length of a “mapSize” variable value.
- After that, retrieve the Map “Key” and “Value” data from the user using the “userInput” instance. The data is then stored in “mapKey” and “mapValue” integer and double-type variables.
- To insert the user-entered data in the Map, pass these variables as arguments in the “put()” method.
- Finally, apply the “keySet()” method over the “demoMap” and pass it into the enhanced “for” loop. This will select and retrieve the information for all Map keys and then display them on the console.
The output for the above code shows the retrieval of Map data entered by the user at runtime:
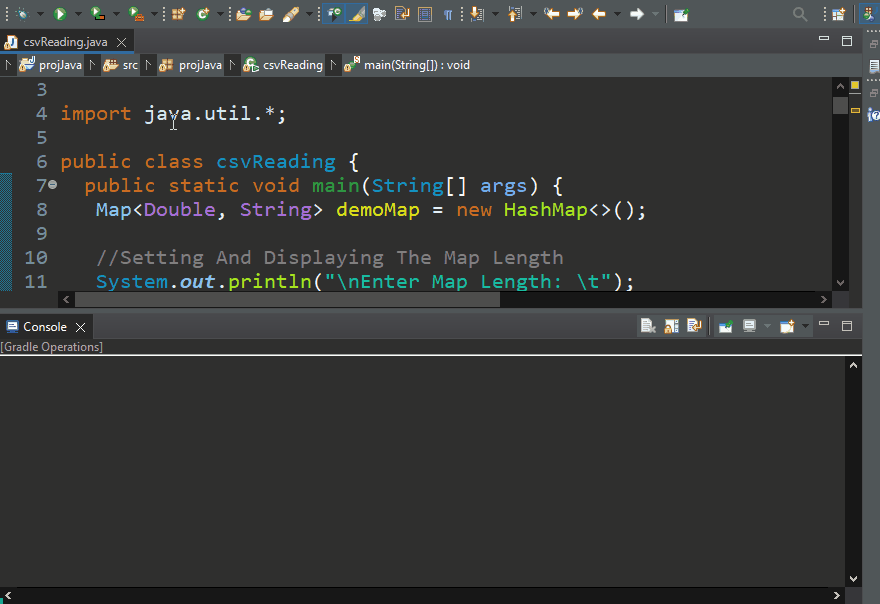
Method 4: Printing HashMap Using “Arrays.asList()” Method
The “Arrays.asList()” method creates a new List from the provided collection which is “Map” in our case. The generated List is then displayed on the console to print the internal mapping, as shown below:
import java.util.*;
public class csvReading {
public static void main(String[] args) {
Map<Double, String> demoMap = new HashMap<>();
demoMap.put(1.4, "Jackson");
demoMap.put(2.2, "Alen");
demoMap.put(3.5, null);
System.out.println("\nThe Map Contains Following Elements: \n");
//Displaying the Map Values
System.out.println("\nThe Map Contains Following Key value pairs: \n");
System.out.println(Arrays.asList(demoMap));
}
}
In the above code snippet:
- First, declare a HashMap named “demoMap” and initialize it with the random values.
- Next, pass this “demoMap” as a parameter for the “Arrays.asList()” method. The returned List is then printed on a console using the “System.out.println()” method.
The output shows the printing of HashMap on the console window:
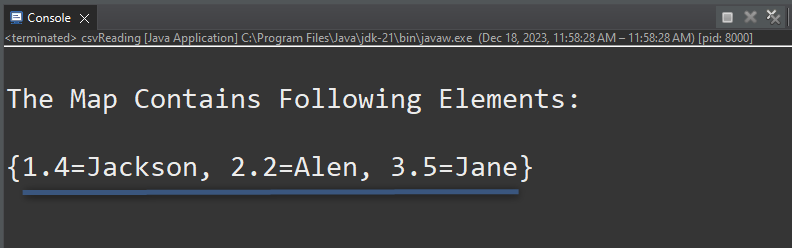
Method 5: Printing HashMap Using “values()” Method
To print only values of HashMap on the console window, the “values()” method is used. This method is used with the enhanced “for” loop to iterate over every key-value pair of HashMap and prints only the values. For instance, the HashMap is first created according to the user-inserted data and then the HashMap values are displayed on the console:
import java.util.*;
public class csvReading {
public static void main(String[] args) {
Map<Double, String> demoMap = new HashMap<>();
//Setting And Displaying The Map Length
System.out.println("\nEnter Map Length: ");
Scanner userInput = new Scanner(System.in);
int mapSize = userInput.nextInt();
System.out.println("\nThe Entered Map Length is: " + mapSize);
//Getting and Setting Key Value Pairs for Map.
for(double i = 0; i<mapSize; i++)
{
System.out.println("\nEnter 'Key' for Map in double: ");
double mapKey = userInput.nextDouble();
System.out.println("\nEnter Map 'Value' in String: ");
String mapValue = userInput.next();
demoMap.put(mapKey, mapValue);
}
//Printing the HashMap in Java
System.out.println("\nThe HashMap Contains Following Key value pairs: ");
for(String mapItems : demoMap.values()){
System.out.println( mapItems );
}
}
}
The above code works like this:
- First, the “Map” named “demoMap” is declared and initialized with the user-defined Keys and Values at runtime as described in the above sections.
- Next, the enhanced for loop is utilized to iterate over the List values provided by the “values()” method.
- In each iteration, the new value for a single HashMap item is selected and displayed on the console window.
The generated output shows that the provided values for HashMap have been printed on the console:
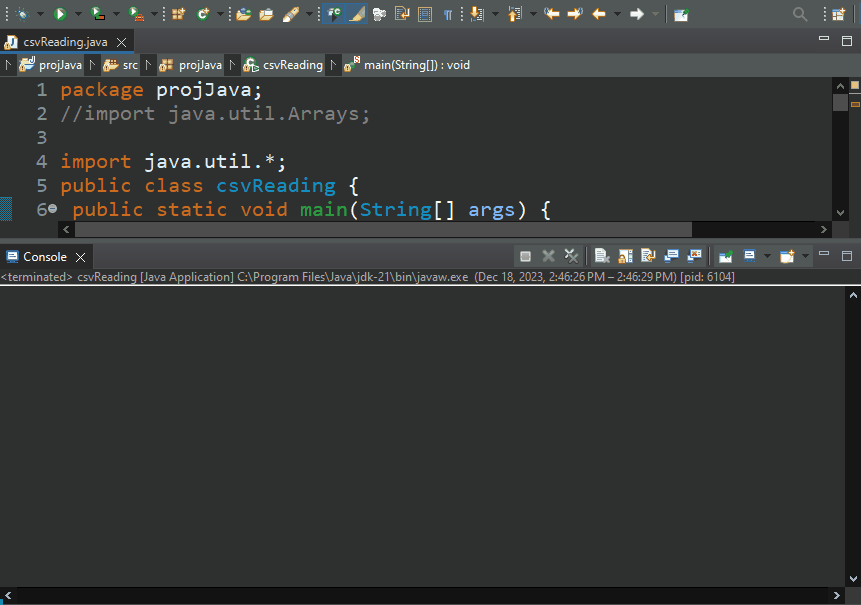
Method 6: Printing HashMap Using “BiConsumer” Interface
The “BiConsumer” interface uses the “lambda” function to perform the specified operation. In our case, this function accepts two arguments of Key and Value that select each item of a Map and then print their values on the console. If the Map key is set to “null” then this method will not display its corresponding value. However, if the value of any Key is set to “null” then its value will be displayed on the console:
import java.util.*;
import java.util.function.BiConsumer;
public class csvReading {
public static void main(String[] args) {
Map<Double, String> demoMap = new HashMap<>();
demoMap.put(1.4, "Jackson");
demoMap.put(2.2, "Alen");
demoMap.put(3.5, null);
demoMap.put(null, "Jane");
BiConsumer<Double, String> printBiconsumer = (key, val) ->
{
System.out.println("The HashMap Key: '" + key + "' has the Value of '" + val + "'");
};
demoMap.forEach(printBiconsumer);
}
}
In the above code block:
- First, the class “csvReading” is created that contains a Map named “demoMap”. Then, random values are inserted in the Map using the “put()” method.
- Next, create a “BiConsumer” interface named “printBiconsumer” and pass two arguments of “key” and “val”.
- Inside this lambda function, print both “key” and “val” arguments on the console window.
- After that, invoke the “forEach()” method and pass this “printBiconsumer” as a parameter. To perform the “printBiconsumer” operation over all elements of the provided “demoMap”.
The output for a provided code shows that the Map is printed on the console:
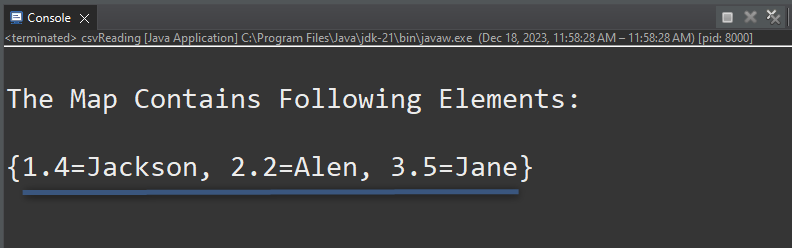
Method 7: Printing HashMap Using “singletonList()” Method
The “Collections” class offers a method named “singletonList()” to return a mutable list consisting only of the specified object provided by another collection. This returned list is then easily printed on the console:
import java.util.*;
class csvReading {
public static void main(String[] args) {
Map<Double, String> demoMap = new HashMap<>();
demoMap.put(12.4, "Moscow");
demoMap.put(23.2, "Qatar");
demoMap.put(53.5, null);
demoMap.put(null, "Italy");
//Displaying
System.out.println("\nThe Provided HashMap Contains Following Key Value Pairs: \n");
System.out.println(Collections.singletonList(demoMap));
}
}
In the above code block:
- First, import the “java.util” package and declare a HashMap named “demoMap”. Also, initialize this HashMap using the “put()” method.
- Next, invoke the “singletonList()” method of the “Collections” class and pass the “demoMap” as their argument.
- The returned result is then passed into the “System.out.println()” method.
The generated output is shown below:
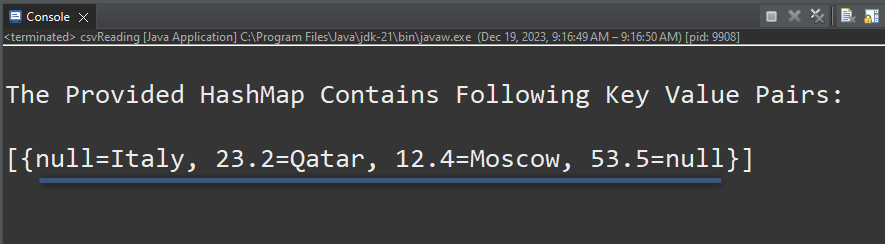
That’s all about the printing of HashMap in Java.
Conclusion
To print a HashMap in Java, the user can utilize the “System.out.println()”, “entrySet()”, “forEach()”, “keySet()”, “Arrays.asList()”, “values()”, “BiConsumer”, or “singletonList()” Methods. Each provided method has its own time complexity and flexibility so you must pick one according to your requirements. The printing of HashMap is vital because it stores the data in key value pairs and the user can store or retrieve data from it with very less time complexity. This guide has explained the printing of Hashmap in Java.