A programmer has to go through the concepts of object-oriented programming during their programming journey. Constructors are an essential part of object-oriented programming. A constructor enables to create and initialize the objects of a class. In Python, the class constructor is the __init__() function which internally constructs the object and initializes it.
In this article, we will discuss the Python class constructor __init__() function, its working, and examples.
Python Class Constructor – Python __init__() Function
A class can be considered a set of things to which certain properties or attributes can be given. An instance of the class is an object performing the same functionalities as its class. __init__() is a reserved method and acts as a class constructor in Python.
Syntax
class ClassName:
def __init__(self, parameter1, parameter2):
self.parameter1 = parameter1
self.parameter2 = parameter2
- ClasName is the name of the class
- def __init__(self, parameter1, parameter2) is defined as the constructor method by which the object properties are initialized using the given parameters
- The instance being created is referred to as self
How does the Python __init__() Function work?
The __init__() method is the same as a C++ constructor in OOP. The function is called whenever an object has to be made from a given class. The method helps to initialize the properties of the object. It is used inside the classes. The Python __init__() function works in the following steps:
- Create a class
- Create an object
- Access the properties of the object
Example to Demonstrate the Working of Python __init__
Every class has a function named __init__() that is automatically executed when the class is defined.
Let’s look at the following example to see how the __init__() function works:
class Personality:
def __init__(self, name, type):
self.name = name
self.type = type
p1 = Personality("Neha", "ENFJ")
print(p1.name)
print(p1.type)
In the above code,
- A class Personality is defined
- The class constructor __init__() gets the parameters: name and type.
- The attributes are stored using self so they can be used later
- An instance of Personality class p1 is created, giving the information of the name (Neha) and type (ENFJ) of the person.
- The code prints out the name of the person using print(p1.name) and the personality type using print(p1.type)
Output:
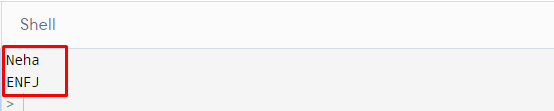
__init__() Function and Inheritance
Inheritance is the process by which a derived class can get (inherit) the properties and attributes of the base class. Let us see the following example that uses the __init__() function along with the inheritance:
class PersonalityType(object):
def __init__(self, type):
self.type = type
class ENFJ(PersonalityType):
def __init__(self, name):
super().__init__("ENFJ")
self.name = name
def personality(self):
print(f"{self.name} personality type is {self.type}")
neha = ENFJ("Neha")
neha.personality()
In the above code,
- The base class PersonalityType is defined with the parameter object.
- The class uses the __init__() constructor taking the parameter type which tells about the information about the personality type.
- A subclass ENFJ is defined that inherits from its parent class PersonalityType
- super().__init__(“ENFJ”) calls the class constructor to get the personality as ENFJ.
- self.name and self.type gives the information about name and the personality type respectively
- An instance neha of the class ENFJ is created with the parameter “Neha”
- The personality() function is called on the instance neha printing out “Neha personality type is ENFJ”
So in this code, the base class constructor is called. It is not absolutely necessary to call the base class constructor initially. The order to call the __init__ method can be changed by calling the base class constructor after the child class constructor has been defined.
Output:

In this article, we learned about the Python class constructor __init__() function, how it works, and how it supports the concept of inheritance.
CONCLUSION
__init__() is the class constructor that helps with instance creation and initialization in Python programming language. Constructors initialize the state of the object. The values are initialized when the class’s object is created. Conclusively, the __init__() method does the object’s creation and initialization making it an essential component in Python programming.