Node.js is a platform that is used to compile or execute JavaScript code over the web server by the utilization of several defaults or external modules like “http” or “express”. The most popular and recommended to perform server operations like sending or receiving requests, the “express” module is used. The user can send specific messages or the entire file and folder using these modules and may encounter several sorts of errors that are going to be handled.
This guide illustrates the possible fixes for the node.js below-mentioned error by covering the below sections:
What is “TypeError: path must be absolute or specify root to res.sendFile” Error in Node.js?
The mentioned error occurs when the path of a selected file that is required to be sent over the server as a response is not valid or unreachable. This error restricts the user from checking the selected file path and its accessibility before sending it to the server.
For instance, visit the below node.js program in which the requested file is not available at the provided location. This unreachable provided path leads to the generation of a “path must be absolute or specify root to res.sendFile” error:
const expressObj = require('express');
const linuxhintApp = expressObj();
const portNumber = 8080;
linuxhintApp.get('/', (req, res) => {
res.sendFile('./wrongDirectory/frontEnd.html');
});
linuxhintApp.listen(portNumber, () => {
console.log(`Linuxhint app is listening on "http://localhost:${portNumber}/"\n`);
});
In the above code:
- First, import the “express” module and create its application named “linuxhintApp” by calling its default constructor. The port number for a localhost server is also stored in a random named “portNumber” variable.
- Next, the “get()” method is attached to send a get response over the local server having the route handler of “/”. This handler means that the request is being sent to the main page which is “http://localhost:8080/” in our case.
- Assign the required “callback” function with the “get()” method having the parameters of “req” and “res”.
- Inside the function, provide the file to be sent as a parameter for the “sendFile()” method invoked by the callback “res” parameter.
- After that, invoke the “listen()” method and pass the “portNumber” variable as its parameter to run the provided “linuxhintApp” over the provided localhost port number.
The output generated for the above code shows the occurrence of the mentioned error due to the provided wrong path of a file:
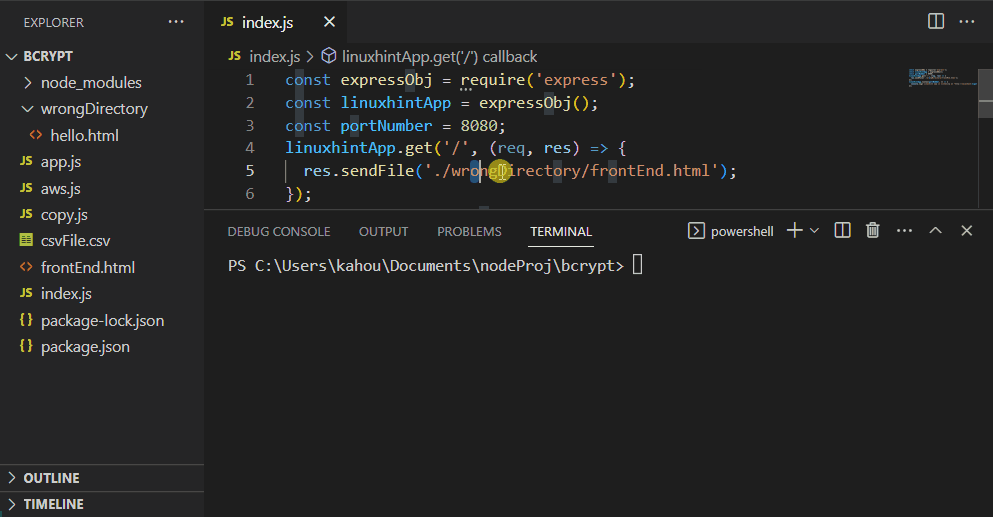
Fix Node.js “TypeError: path must be absolute or specify root to res.sendFile”?
To resolve the mentioned error, the user must provide the absolute path of the selected path or retrieve the file path using the provided path finder properties. There are various quick fixes as well to resolve the specified “path must be absolute or specify root to res.sendFile” error which are being demonstrated below.
Fix 1: Use of “__dirname” Variable to Solve Error In Both ES6 and ES5 Version
The “__dirname” variable retrieves the absolute path for the currently executing file. For instance, the absolute path for the currently executed file “index.js” is going to be retrieved. The retrieval of a path using the specified variable reduces the chance of human error in providing an invalid or unreachable path.
Since there are two available modules for developers in node.js, the “ES5” and “ES6”. The following code represents the prevention of a specified error using the “__dirname” in the ES6 module:
import expressObj from 'express';
import pathObj from 'path';
import {fileURLToPath} from 'url';
const selectedFile = fileURLToPath(import.meta.url);
const directoryPath = pathObj.dirname(selectedFile);
const linuxhintApp = expressObj();
const portNumber = 8080;
linuxhintApp.get('/', (req, res) => {
res.sendFile(directoryPath + '/frontEnd.html');
});
console.log("\nAdress of Send File: ", directoryPath);
linuxhintApp.listen(portNumber, () => {
console.log(`Linuxhint app is listening on "http://localhost:${portNumber}/"\n`);
});
Explanation of the above code block is as follows:
- First, the ES6 “import” statement is used to import the “express” and “path” modules and store their objects in random “expressObj” and “pathObj” variables. The default method named “fileURLToPath()” is also imported from the “url” module.
- Next, pass the “import.meta.url” property into the “fileURLToPath()” method to retrieve the absolute path for the file from where the script is running.
- Store this path in the custom-built variable “selectedFile” which is then passed as the parameter for the “dirname()” method.
- This method returns the currently working directory path which is done by the “__dirname” variable in the ES5 module. The output or generated path is stored in the “directoryPath” variable.
- Then, the express application named “linuxhintApp” is created and the localhost port number is stored in the “portNumber” variable.
- Now, set up the “get()” method having the route handler of “/” which sends a file by invoking the “sendFile()” method. The path of that file is concatenated with the “file name” and “directoryPath” variables.
- After that, the generated valid address for the selected file is displayed on the console for verification purposes.
- Finally, the “listen()” method is used to set the server on the provided “portNumber”.
The generated output shows that the file has been served without generating the “path must be absolute or specify root to res.sendFile” error:
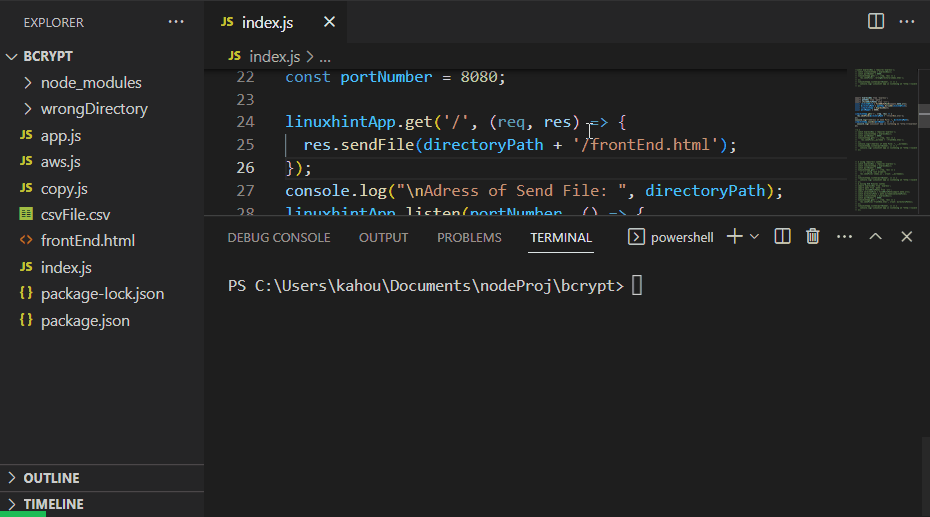
Representation of the above code to prevent the mentioned error in an ES5 CommonJs version is written below:
const expressObj = require('express');
const linuxhintApp = expressObj();
const portNumber = 8080;
linuxhintApp.get('/', (req, res) => {
res.sendFile(__dirname + '/frontEnd.html');
});
console.log("\nAdress of Send File: ", __dirname);
linuxhintApp.listen(portNumber, () => {
console.log(`Linuxhint app is listening on "http://localhost:${portNumber}/"\n`);
});
Explanation of the above code is as follows:
- First, the “express” module is imported, and using this module, the express application named “linuxhintApp” is created. The port number for the localhost server is also stored in a “portNumber” named variable.
- Next, the “get()” method is invoked having the root handler of “/” and inside its callback function the “sendFile()” method is used. Inside its parenthesis, the absolute path is created for a selected file by concatenating it with the “__dirname” variable.
- After that, the path of the current directory stored in “__dirname” is printed for verification purposes. In addition, the application “linuxhintApp” is served over the local server with a specified port number.
The generated output shows that the mentioned “path must be absolute or specify root to res.sendFile” error has been resolved:
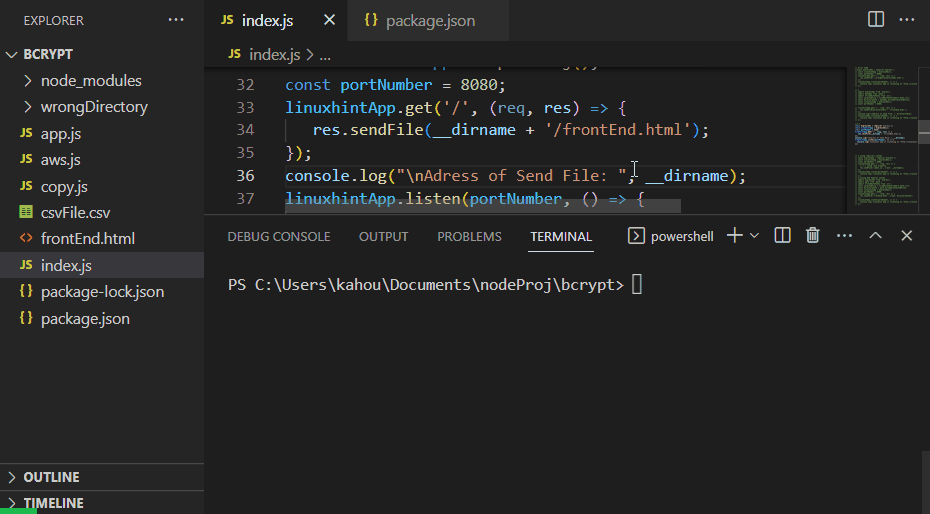
Note: Visit the linked error, just in case you encounter the “SyntaxError: Cannot use import statement outside a module” node.js error while executing the above code. This error is generated due to a different modular version.
Fix 2: Use of “root” Property In Both ES6 and ES5 Modular Version
The “root” property in node.js is used to identify the root directory path for relative file names. In our case, it retrieves the currently executing directory’s absolute path concatenated with the selected file “frontEnd.html” path. To resolve the mentioned “TypeError: path must be absolute or specify root to res.sendFile” error. Visit the below line of code in an ES6 modular version:
import expressObj from 'express';
import pathObj from 'path';
import {fileURLToPath} from 'url';
const selectedFile = fileURLToPath(import.meta.url);
const directoryPath = pathObj.dirname(selectedFile);
const linuxhintApp = expressObj();
const portNumber = 8080;
linuxhintApp.get('/', (req, res) => {
res.sendFile('frontEnd.html', {root: directoryPath});
});
linuxhintApp.listen(portNumber, () => {
console.log(`Linuxhint app is listening on "http://localhost:${portNumber}/"\n`);
});
The above-shown code is the same as we have already described in the above section “ES6” module version part. Only the “directoryPath” variable is now passed as the value for the “root” property as an optional parameter for the “sendFile()” method.
The generated output shows the file has been served over the network without generating the mentioned error:
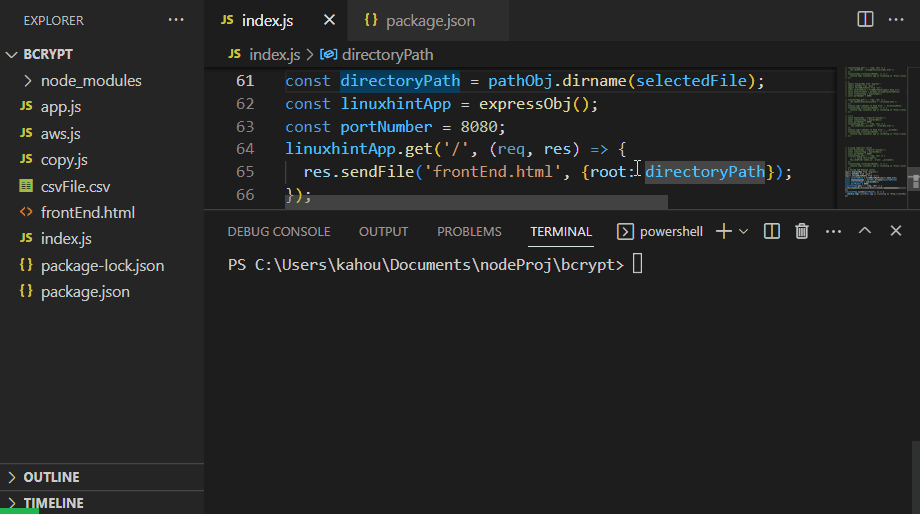
The representation of the above-stated code in an ES5 CommonJs version will be:
const expressObj = require('express');
const linuxhintApp = expressObj();
const portNumber = 8080;
linuxhintApp.get('/', (req, res) => {
res.sendFile('frontEnd.html', {root: __dirname});
});
linuxhintApp.listen(portNumber, () => {
console.log(`Linuxhint app is listening on "http://localhost:${portNumber}/"\n`);
});
In the above code block:
- The “__dirname” is passed as the value for a “root” property which is available as an optional parameter for the “sendFile()” method.
- This allotment of the absolute path to a selected file reduces the chances of the occurrence specified error.
- The remaining code is the same as described in the above section “ES5” modular part.
The generated output confirms the error is now resolved and the selected file is served over the server as needed:
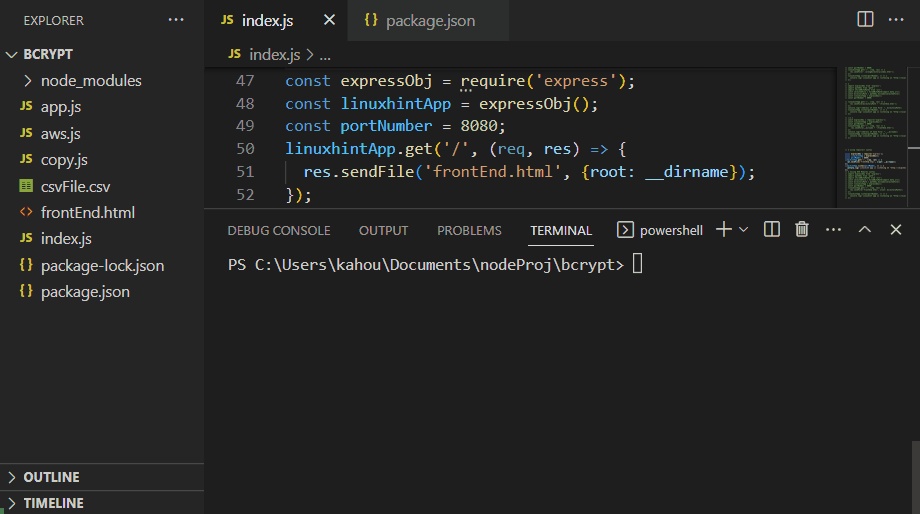
Fix 3: Use of “join()” Method to Solve Error In Both ES6 and ES5 Version
The “join()” method provided by the “path” module joins or merges the provided two parts of the URL or path into a single one. It can be known as a concatenation operation for provided paths or URLs. For instance, the absolute path of the current directory is going to be joined with the file name which is going to be served over the local server.
The code provided in the above section for the ES6 modular version to integrate the “join()” method gets modified like this:
import expressObj from 'express';
import pathObj from 'path';
import {fileURLToPath} from 'url';
const selectedFile = fileURLToPath(import.meta.url);
const directoryPath = pathObj.dirname(selectedFile);
const linuxhintApp = expressObj();
const portNumber = 8080;
linuxhintApp.get('/', (req, res) => {
res.sendFile(pathObj.join(directoryPath, 'frontEnd.html'));
});
linuxhintApp.listen(portNumber, () => {
console.log(`Linuxhint app is listening on "http://localhost:${portNumber}/"\n`);
});
In the above code:
- The “join()” method contains an absolute directory path and the “file” name that needs to be served as the parameters.
- The result of this “join()” method is then passed as an argument for the “sendFile()” method.
- To get the authentic absolute file path of a file that needs to be served over the server.
Output after the execution of the provided code shows that the file has been served without any error:
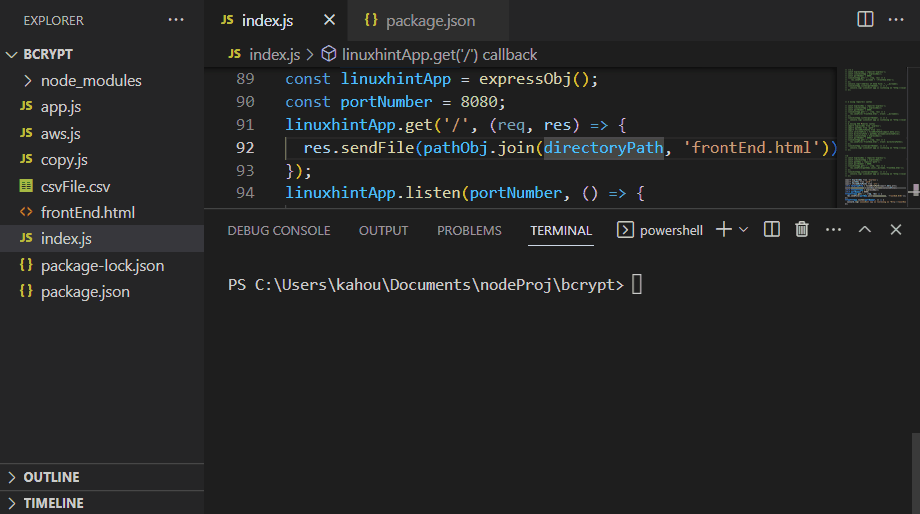
The representation of utilizing the “join()” method in the ES5 CommonJs version to resolve the mentioned error is shown below:
const expressObj = require('express');
const linuxhintApp = expressObj();
const pathObj = require('path');
const portNumber = 8080;
linuxhintApp.get('/', (req, res) => {
res.sendFile(pathObj.join(__dirname,'frontEnd.html'));
});
linuxhintApp.listen(portNumber, () => {
console.log(`Linuxhint app is listening on "http://localhost:${portNumber}/"\n`);
});
In the above code:
- The “join()” method having the “__dirname” and the selected file to be served as the first and second parameters is passed to the “sendFile()” method.
- To create a custom absolute path for selected files, reducing the chances of invalid or faulty paths.
- The remaining code is the same as described in the above section of the ES5 CommonJs part.
The generated output for the above code shows that the selected file has been served fixing the mentioned error:
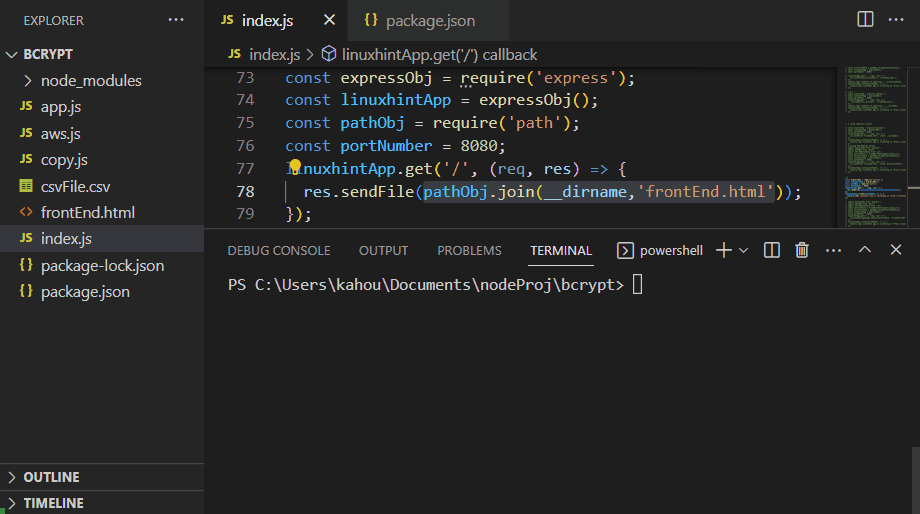
That’s all about fixing the node.js mentioned error.
Conclusion
To fix the node.js “path must be absolute or specify root to res.sendFile” error use the “join()” method provided by the “path” module, the “root” property or “__dirname” variable to find the absolute path for the current directory. These approaches are used perfectly in both ES6 and ES5 JavaScript versions. As an alternative to the “__dirname” variable, the “import.meta.url” property is passed to the “fileURLToPath()” to retrieve the absolute directory path in ES6. This guide has illustrated the fixes to resolve the mentioned error.