“Squaring” a number in Java helps in solving mathematical calculations. These calculations can be long multiplication, calculating area, square root, etc. It is represented by “a²” where “2” is the exponent/power for squaring the number “a”. This approach (i.e., squaring) is useful in situations where there is a need to get rid of negative values in the code that results in code complexity.
What is the Square of a Number in Java?
Upon multiplying a number by itself, the returned product is called the square of a number. For instance, the square of a number “x” is calculated as “x * x”.
How to Square a Number in Java?
A number can be squared by multiplying the number by itself or using the “Math.pow()” method.
Approach 1: Square a Number in Java by Multiplying it by Itself
In this method, a number can be squared by multiplying it by itself.
Example 1: Squaring an Initialized Number
In this example, an initialized double number will be squared:
package jbArticles;
public class Square {
public static void main(String args[]) {
double a = 3;
double b = a * a;
System.out.println("Given Number -> "+a); System.out.println("Square of Number -> "+b);
}
}
Code Explanation
- Initialize the given double number to be squared.
- In the next step, square the given value and store the resultant value in another variable “b”.
- Lastly, return the given and the squared numbers, respectively.
Output
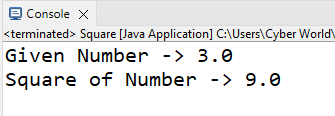
This output implies that the square of the given number is returned appropriately.
Example 2: Squaring a User Input Number
In this specific example, a user-input custom number will be squared using the “Scanner” class:
package jbArticles;
import java.util.Scanner;
public class Square {
public static void main(String args[]) {
Scanner ob = new Scanner(System.in);
System.out.println("Enter the number: ");
double a = ob.nextDouble();
double b = a * a;
System.out.println("Given Number -> "+a);
System.out.println("Square of Number -> "+b);
ob.close();
}
}
Code Explanation
- Import the specified library to use the “Scanner” class and enable user input.
- In “main”, create a Scanner object. The “System.in” parameter enables the user input.
- The “nextDouble()” ensures that the user-entered value is double.
- After that, square the user-input number by itself and return the given as well as the squared number.
Output
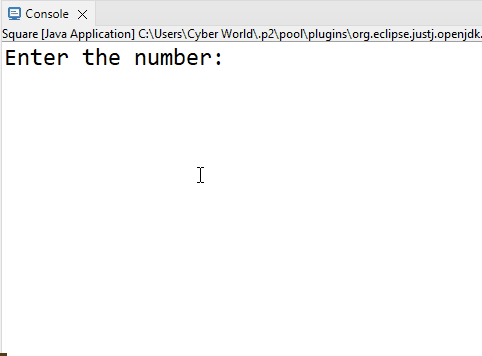
Approach 2: Square a Number in Java Using the “Math.pow()” Method
The “Math.pow()” method calculates a number raised to the power of some other number. This method can also be used to square a number by specifying the power of the base as “2”.
Syntax
public static double pow(double base_value, double exponent_value)
Return Value: xy
Following is the demonstration:
package jbArticles;
public class Square {
public static void main(String args[]) {
System.out.println("Square of Number -> "+Math.pow(4, 2));
}
}
Code Explanation
In this code, use the “Math.pow()” method to square the base number “4” (as a first argument) by specifying its power as “2” (as a second argument).
Output
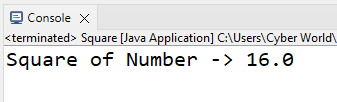
How to Square a Negative Number in Java?
Squaring a negative number also returns the output as a positive number since the negative sign is multiplied twice. The below example calculates the square of a negative number instead:
package jbArticles;
public class Square {
public static void main(String args[]) {
System.out.println("Square of Number -> "+Math.pow(-4, 2));
}
}
Code Explanation
Here, the negative number i.e., “-4” is specified as the “Math.pow()” method first argument and it is squared accordingly.
Output
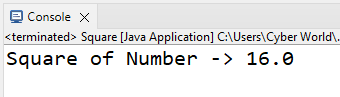
Bonus Tip: Finding Square Root of a Value in Java
The square root of a number “x” is a value that retrieves the original number when multiplied by itself. For instance, the square root of a number “x” is “?x” e.g. “?81 -> 9”. This process is completely opposite to squaring a number.
Below is the code demonstration:
package jbArticles;
public class Square {
public static void main(String args[]) {
double a = 64;
System.out.println(Math.sqrt(a));
double b = -64;
System.out.println(Math.sqrt(b));
double d = 1.0/0;
System.out.println(Math.sqrt(d));
}
}
Code Explanation
Initialize the specified double positive, negative, and infinite values, respectively, and calculate their square roots using the “Math.sqrt()” method.
Output
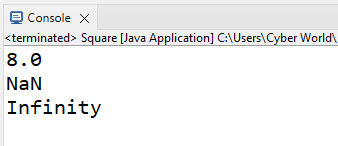
Since the square root of a negative number is “NaN” and the square root of positive infinity is positive infinity, the corresponding square roots are returned accordingly.
Conclusion
The square of a number is calculated by multiplying the number with itself or using the “Math.pow()” method. The “Math.pow()” method takes the exponent value as “2” to calculate the square of the specified base as its arguments.
The square of a negative number, however, returns the same output as that of a positive number since squaring the negative sign also results in a positive value.