We know that the strings are human-readable data types i.e. these data types can easily be understood by humans but these can not be understood by the computer. The machine first converts them into byte objects to store them on the disk. This encoding may be of many types. In this article, we will discuss strings-to-byte conversion in Python 3. Let’s get started.
How to Convert Strings to Bytes in Python 3?
In order to make the string/text readable to the machine, we convert them into the byte format. There are several methods by which we can convert a string into bytes. Let’s discuss them one by one.
Method 1: Using bytes(String , Encoding)
We can encode the string into bytes using this byte function. There are several conversion or encoding techniques that are being used but the most significant ones are the ASCII and UTF-8.
Let’s see an example of encoding using this method.
Example
Consider the code below which will demonstrate the conversion into a byte with both encoding techniques:
val = "This is String-to-Byte conversion"
# encoding in 'UTF-8'
conv1 = bytes(val, 'utf-8')
# encoding in 'ASCII'
conv2 = bytes(val, 'ascii')
print(conv1,'\n') # This will print the original string named as val
for byte in conv1:
print(byte, end=' ') # This will print the byte code in UTF-8 technique
print("\n")
for byte in conv2:
print(byte, end=' ') # This will print the byte code in ASCII technique
The the above code we have encoded the string into both the encoding techniques using the byte function. The outcome for the provided code will be:
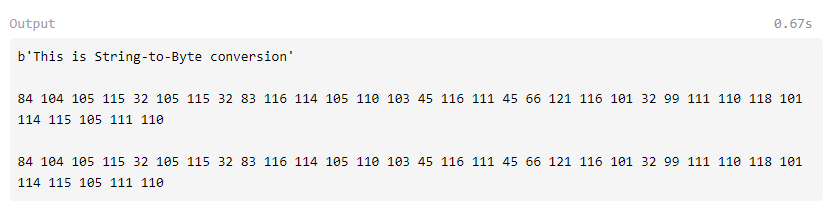
Let’s move towards an alternate method.
Method 2: Using encode(enc)
This method is the most recommended method among all the methods. The encode() function takes in the type of technique we want our string to be encoded in. The code for conversion is given below:
val = "This is String-to-Byte conversion"
# encoding with encode() function
conv1 = val.encode('utf-8')
conv2 = val.encode('ascii')
print(conv1,'\n')
# printing the utf-8 code
for byte in conv1:
print(byte, end=' ')
print("\n")
# printing the ascii code
for byte in conv2:
print(byte, end=' ')
The above code will cover the given string named as val into the bytes using the encode() function using both the encoding techniques like this:
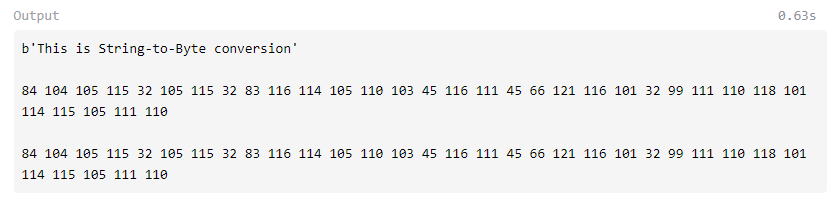
This was so simple.
Method 3: Using binascii.unhexlify() method
In this method, we use the binascii.unhexlify() method. For this method, we will first have to import the binascii module. Next, we will have to initialize the string having the hexadecimal representation of bytes. We will then be using the unhexlify() method to convert that string to bytes. We can code this description like this:
import binascii
val = "This is String-to-Byte conversion"
# encoding with encode() function
conv1 = binascii.unhexlify(val)
print(conv1,'\n')
for byte in conv1:
print(byte, end=' ')
The above code will convert the string into the byte format. The time complexity of binascii.unhexlify() method is O(n) where n denotes the input string’s length. While the remaining operations have the complexity of O(1). Therefore, the overall time complexity of this code is O(n).
Conclusion
We can convert a string to a byte in order to make the text more machine-readable. There are three methods to convert a string into byte; by using the byte(), by making use of the encode() function, and by using binascii.unhexlify() method. In this post, we have comprehended all these methods along with their practical examples.