In PyTorch, the “torchvision.transforms” module has a collection of classes and functions to apply different transformations on desired images including rotating, cropping, resizing, and many more. It provides the “RandomResizedCrop()” method to crop a random area of a particular image and resize it to a specific size. Users are required to define the specific crop size i.e. image’s height and width. By using this method, the randomly cropped image of the defined size will be generated.
This article will demonstrate the method to crop a random area of an image and resize it to a specific size in PyTorch.
How to Crop a Random Area of Image and Resize it in PyTorch?
To crop a random area of an image and resize it to a specific size in PyTorch, try out the below-provided steps:
- Upload/add an image to Google Colab
- Install necessary libraries
- Read uploaded input image
- Define a transform for cropping a random area of the image and resizing it
- Apply transform on uploaded image
- Print randomly cropped and resized image
Step 1: Upload/Add an Image to Google Colab
First, add the desired image to the notebook by tapping on the below-highlighted icons:
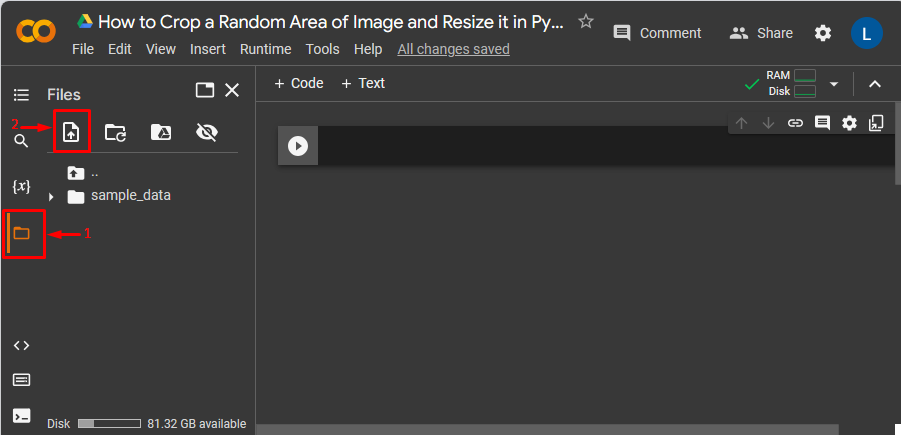
This has added the image to Google Colab:
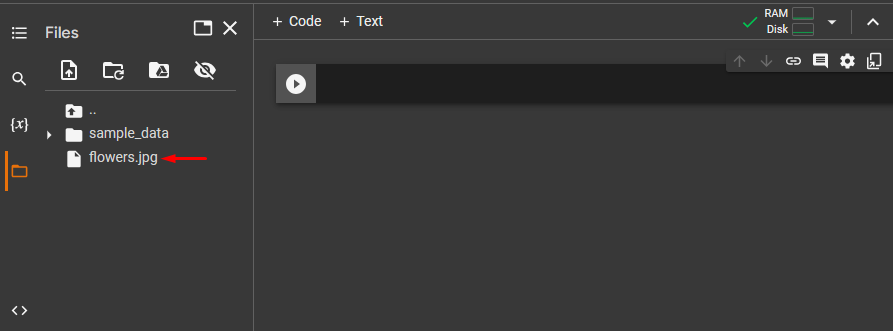
Below is the image that we have added to crop a random area of it and resize it:
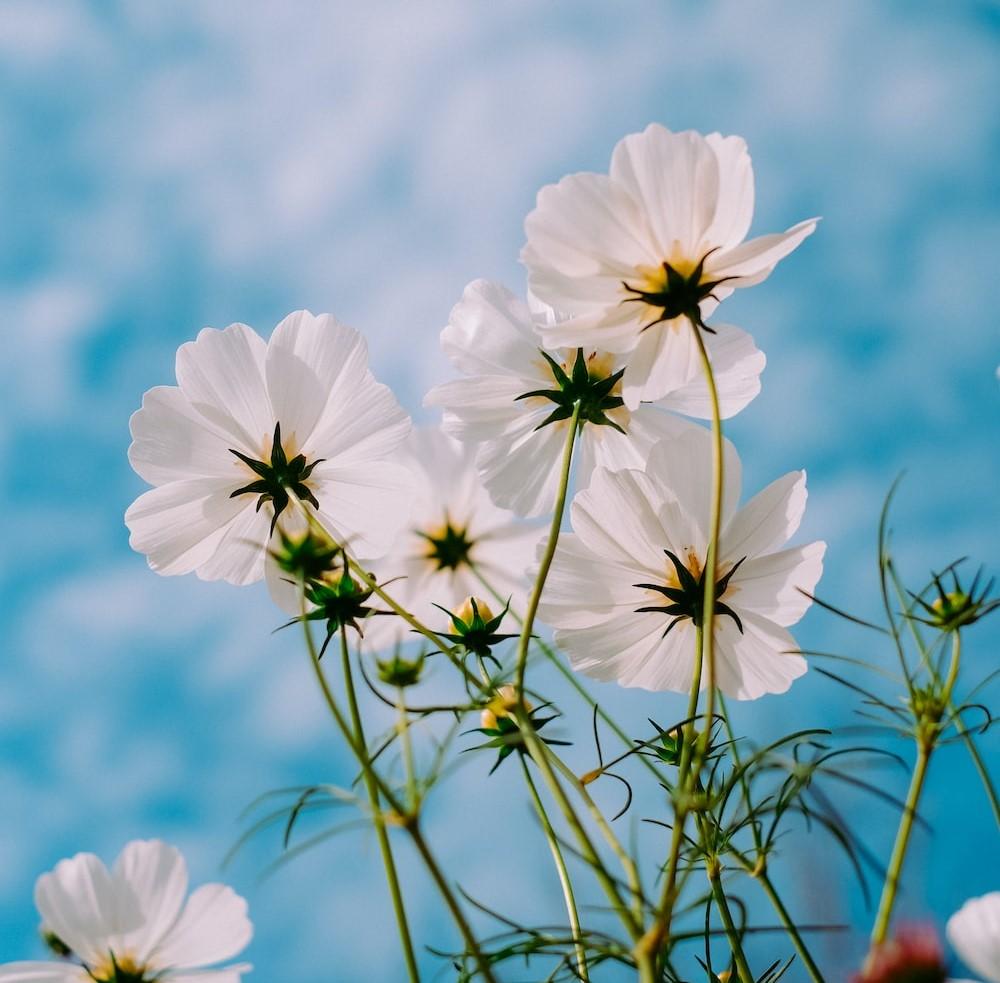
Step 2: Install Necessary Libraries
Next, install the following libraries that are necessary for applying operations on images:
import torch, torchvision.transforms as transforms
from PIL import Image
In the above snippet:
- “import torch” imports PyTorch package.
- “import torchvision.transforms as transforms” imports the “transforms” module as a “transforms” for preprocessing image data.
- “from PIL import Image” imports “Image” from “PIL” for opening and saving various image file formats:

Step 3: Read the Uploaded Input Image
Then, utilize the “Image.open()” function to read the uploaded image i.e. “flowers.jpg” from the computer:
input_img = Image.open('flowers.jpg')

Step 4: Define a Transform
After that, define a transform through the “RandomResizedCrop()” method to crop the random area of the above input image and resize it to a specific size. For the random square crop, we need to define one value and two values can be defined for the random rectangle crop. Here, we have specified two values of height and width i.e. “200, 300” for the random rectangle crop:
transform = transforms.RandomResizedCrop(size=(200, 300))

Step 5: Apply Transform on the Uploaded Image
Now, apply defined transform on the uploaded input image to crop its random area and resize it:
cropped_img = transform(input_img)

Step 6: Display Cropped and Resized Image
Finally, print the cropped and resized image:
cropped_img
The below output shows the randomly cropped and resized image:
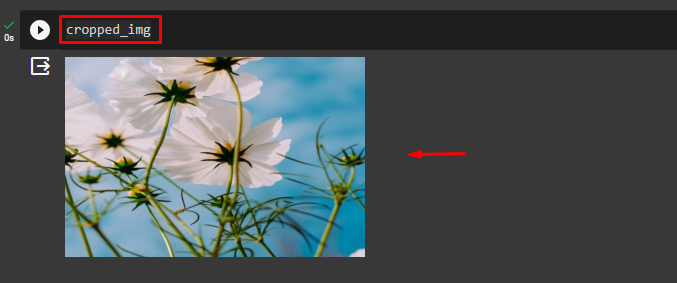
Likewise, users can define one value in the “RandomResizedCrop()” method to crop the random area of the image in a square shape. Moreover, the “scale” and “ratio” parameters can also be utilized to specify the upper and lower bounds for the random area and aspect ratio respectively:
transform = transforms.RandomResizedCrop(size=(200), scale=(0.2, 0.7), ratio=(0.5, 1.2))

This has cropped the random area of the image in a square shape:
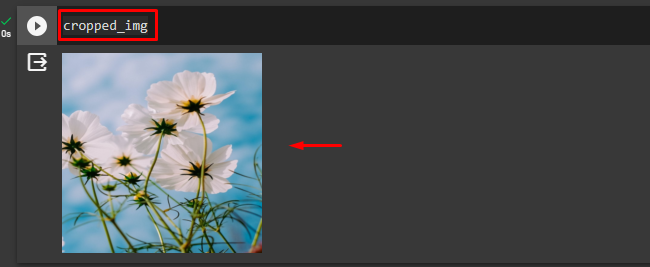
Comparison
In the below table, the comparison between the original image and randomly cropped and resized images can be observed:
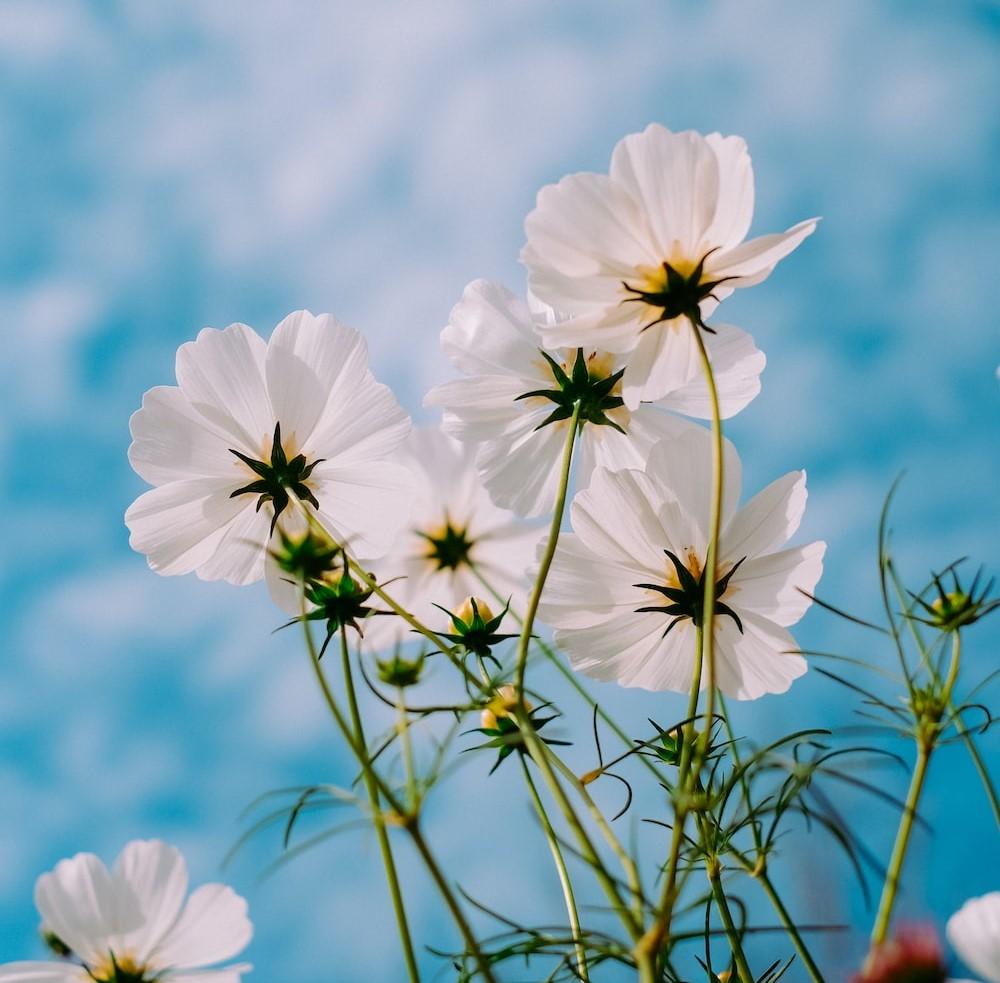
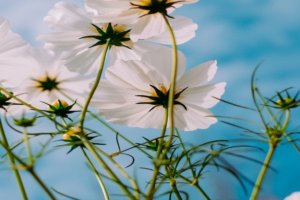
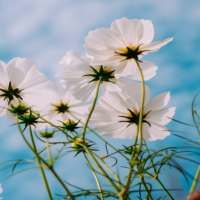
We have explained the method of cropping a random area of an image and resizing it in PyTorch.
Note: Click on the provided link to access our Google Colab Notebook.
Conclusion
To crop a random area of an image and resize it to a specific size in PyTorch, first, add the desired image to Google Colab. Then, install the required libraries and read the uploaded input image. After that, utilize the “RandomResizedCrop()” method to crop the random area of the input image and resize it. Finally, display the cropped and resized image. This article has demonstrated the method to crop a random area of an image and resize it to a specific size in PyTorch.