The norm of a matrix or vector is a computation of its length/magnitude. In PyTorch, a vector is simply a 1D tensor that has only one dimension whereas a matrix is a 2D tensor that has two dimensions i.e. rows and columns. Sometimes, users may want to calculate the norm of a vector or a tensor for various reasons, such as normalization. PyTorch provides a “torch.linalg.norm()” method to find/calculate the norm of the desired vector or matrix. Moreover, it only allows the inputs of float, cfloat, double, etc.
This article will illustrate the following content:
- How to Calculate/Find the Norm of a Vector in PyTorch?
- How to Calculate/Find the Norm of a Matrix in PyTorch?
How to Calculate/Find the Norm of a Vector in PyTorch?
To calculate the norm of the vector in PyTorch, follow the below-mentioned steps:
- Import PyTorch library
- Create a vector/1D tensor
- Calculate the norm of the vector
- Print the calculated norm of the vector
Step 1: Import PyTorch Library
First, install the “torch” library to compute the vector norm:
import torch

Step 2: Define a Vector/1D Tensor
Then, define a vector/1D tensor and display its elements. Here, we are defining the following “vector” tensor from a list using the “torch.tensor()” function:
vector = torch.tensor([7., -4., 2., 0., 9., -1., -5.])
print(vector)
This has created a vector as seen below:

Step 3: Calculate/Find the Norm of the Vector
Now, use the “torch.linalg.norm()” method and pass the above-created vector as an argument to calculate its norm:
norm_vec = torch.linalg.norm(vector)

Step 4: Print Calculated Norm
Finally, display the calculated norm of the vector:
print(norm_vec)
The below output shows the calculated norm of the vector:

How to Calculate/Find the Norm of a Matrix in PyTorch?
To calculate the norm of the matrix in PyTorch, look at the given-provided steps:
- Import PyTorch library
- Create a matrix/2D tensor
- Calculate the norm of the matrix
- Print the calculated norm of the matrix
Step 1: Import PyTorch Library
First, install the “torch” library to compute the matrix norm:
import torch

Step 2: Create a Matrix/2D Tensor
Then, use the “torch.tensor()” function to create a matrix/2D tensor and display its elements. Here, we are making the following “matrix” 2D tensor:
matrix = torch.tensor([[2., 4., -6.],
[-1., 3., 5.],
[7., -8., 9.]])
print(matrix)
This has created a matrix as seen below:
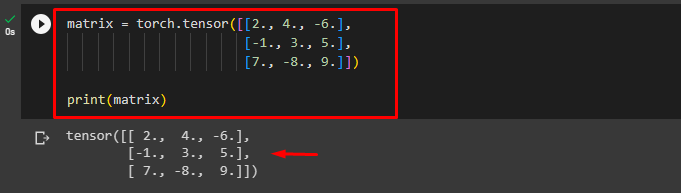
Step 3: Calculate/Find the Norm of the Matrix
Now, find the norm of the above-created matrix using the “torch.linalg.norm()” method and pass the matrix as an argument:
norm_mat = torch.linalg.norm(matrix)

Step 4: Print Calculated Norm
Lastly, display the calculated norm of the matrix:
print(norm_mat)
The below output displays the calculated norm of the matrix:

Moreover, users can also calculate the norm of the matrix along different dimensions. To do so, follow the next provided steps.
Step 5: Calculate/Find the Norm of the Matrix Column-wise
To calculate the norm of the matrix column-wise, specify the “dim=0” as seen in the below code:
norm0 = torch.linalg.norm(matrix, dim = 0)
print(norm0)
In the below output, the norm of the matrix column-wise can be seen below:

Step 6: Calculate/Find the Norm of the Matrix Row-wise
To calculate the norm of the matrix row-wise, specify the “dim=1” as seen in below code:
norm1 = torch.linalg.norm(matrix, dim = 1)
print(norm1)
It can be observed that the norm of the matrix row-wise has been calculated successfully:

We have efficiently explained the method to calculate the norm of the vector and matrix in PyTorch.
Note: The link to our Google Colab Notebook is accessible right here.
Conclusion
To calculate the norm of the vector or a matrix in PyTorch, install the “torch” library. Then, define the desired vector/1D tensor or matrix/2D tensor and view its elements. Next, use the “torch.linalg.norm()” method and pass the vector or tensor as an argument to calculate its norm. Finally, display the calculated norm of the vector or tensor. This article has illustrated the method to calculate the norm of the vector and matrix in PyTorch.