In Python, we can print the colored text in a Python terminal. Majorly, there are 3 ways to change the text color in Python. This article consists of all these 3 methods to print the colored text onto the Python terminal. Let’s see how it is done.
How to Print Colored Text to the Terminal/Console in Python?
There are 3 different commonly used methods by which we can print the colored text in the Python terminal. These methods are:
- Method 1: Using Termcolor Module
- Method 2: Using ANSI code
- Method 3: Using Colorama Module
Let’s discuss them one by one.
Method 1: Using Termcolor Module
The termcolor module is a Python module for printing color text on the Python terminal. We can see the example to better understand its functionality.
Example: Printing Colored Text on Python Terminal Using Termcolor Module
Here is a simple example of the termcolor module color printing. The code is given below:
from termcolor import colored
print(colored('Colored Text', 'blue'))
In the above output:
- First, we have to import “colored” from the “termcolor” module.
- The colored() method contains two parameters. The first parameter is the text you want to print and the second parameter is the color of text you want.
The above code will print the text “Colored Text” in blue color on the Python terminal. The output of the above program is:
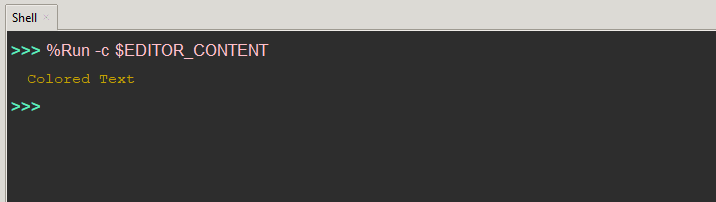
We will now move toward the second method to print the colored text to the Python terminal.
Method 2: Using ANSI Code
The most commonly used method to print the colored text to the Python terminal is by utilizing the ANSI escape sequence/code directly. The syntax of this method can be demonstrated using an example.
Example: Printing Colored Text on Python Terminal Using ANSI Code
A simple example of printing the colored text on a Python terminal using ANSI code is. The basic syntax looks like this:
print('\033[96m' + 'Colored Text' + '\033[00m')
We will now break down the structure of the above code statement we are looking at:
- The “\033” calls a function. “\x1b” is also used for the same purpose.
- The “m” is the function name. Whereas m means the Select Graphics Rendition function also known as SGR function.
- The “Colored text” corresponds to the text that is to be shown in color.
- The part “\033[96m” gives the foreground color which is cyan color and the part “\033[00m” gives the background color which depicts it as plain.
- We can discover more about the ANSI escape sequence codes by referring to the ANSI escape code.
The code will result in the text “Colored Text” printed on the terminal in Cyan color. It will look like this:
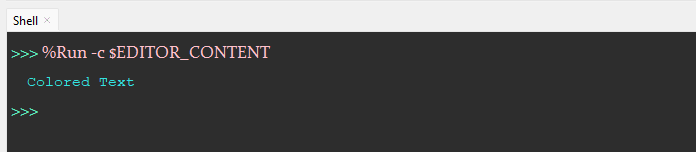
Method 3: Using Colorama Module
The Colorama module is the cross-platform module for printing the colored and styled text on the terminal. Let’s consider an example.
Example: printing green colored text with background and style on Python terminal.
We can execute the following code to print the colored text like this:
from colorama import Fore, Back, Style
print(Fore.GREEN + 'GREEN')
print(Back.RED + 'GREEN with RED background')
print(Style.DIM + 'dim text')
print(Style.RESET_ALL)
print('original text')
In the above syntax:
- First, we will import the colors and the styles we want to apply in the text from the Colorama.
- In the very next statement, we simply printed the green-colored text.
- The next line depicts that we have added a red background to it.
- In the next line, we have added a style and lastly, we have printed the original text that is printed in the default color and style.
The output of the above code looks like this:
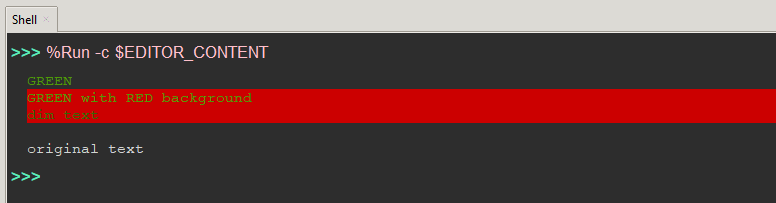
Conclusion
We can print the colored text on the Python terminal. There are three easy/convenient approaches to do this. The first way is by using the “termcolor” module. The second way is by using the ANS code and the last method is by using the Colorama module in Python. All these methods are effective in printing the color text on the terminal but the most commonly used is by using ANSI escape sequence. In this blog, we have comprehended all these methods with the help of examples and syntax.