Floating point numbers can be unnecessarily long sometimes. In such a case, we need to round down the number to some specified decimal numbers. Several built-in functions are offered/supported by Python that can be utilized for different purposes. In this post, we will see how can we round a number to 2 decimals in Python. Let’s get started with the article.
How to Round Numbers to 2 Decimals in Python
To round a number to 2 decimals in Python there are 4 simple methods that we can use. These 4 methods are:
- Method 1: Using the round() function
- Method 2: Using format() function
- Method 3: Using the ceil() function
- Method 4: Using Decimal Module
Let’s discuss these functions one by one along with their practical examples.
Method 1: Using the round() function
The round() function is a built-in function that rounds off a number up to a defined number of decimal/fractional points. The generic syntax used for this function is:
round(Num, Decimal_pts)
The function takes in 2 parameters:
- The first one is a number that needs to be rounded off.
- The second one is the decimal points or number of decimal places up to which we want to round off the number.
The function will retrieve the rounded-off number up to the prescribed number of decimal places/points.
Let’s understand the function with the help of examples.
Example
We will write the following code to see what the round() function does:
print("The number is rounded as :" ,round(5))
# round function with floating point
print("The number is rounded as :" ,round(5.24525,2))
print("The number is rounded as :" ,round(90.5678,2))
print("The number is rounded as :" ,round(-17.853,2))
The above code contains the code statements for the integer and the floating point case. The output for the above code is:

We can see, that all the numbers given to the round() function have been rounded off up to the specified decimal point which is 2.
This is how the round() function in Python works.
Method 2: Using format() function
The second method is by making use of the format() function. This function also takes in the number and decimal point up to which we want to round off our number as the parameter. Let’s see an example of this function.
Example
Consider the following code to understand the functioning of the format() function:
print("The number 123.4567 upto 2 decimal places is:", format( 123.4567,".2f"))
The output for the function gives the number rounded off up to 2 decimal places like this:

We can also write the above query in str.format() way like this:
print("The number 123.4567 upto 2 decimal places is:{:0.2f}".format( 123.4567))
The output for the query will be the same as above:
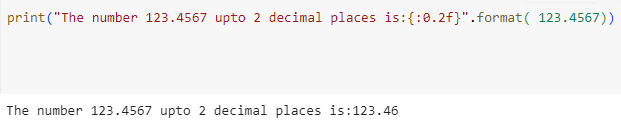
Let’s move toward the next way of rounding off numbers up to 2 decimal points.
Method 3: Using ceil() function
The Python math module provides the ceil() function to round up a number. We can also utilize this function to round a number to 2 decimal positions like this:
import math
number = 12.34567
print(math.ceil(number*100)/100)
In this code, we have simply declared a number and first multiplied the number by 100 to shift the decimal point by 2 decimal places and then divided it by 100 to compensate for it. The output of the code is:
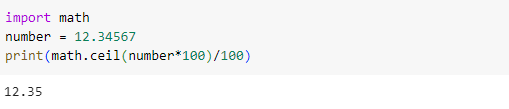
Let’s move towards the last method.
Method 4: Using Decimal Module
The decimal module in Python can also round a floating point number to 2 decimal places. This module needs to be imported into the program to make it work. The “decimal.Decimal(floating pt number)” by default gives the decimal value having 50 digits. The other “value.quantize(decimal.Decimal(‘0.00’))” gives the digits rounded up to 2 decimal places. The syntax for this method is:
import decimal
num = 123.456789
decimalValue = decimal.Decimal(num)
round_off = decimalValue.quantize(decimal.Decimal('0.00'))
print("The original number is: ", num)
print("The number after Rounding off upto 2 decimal places is:", round_off)
The output is as follows:
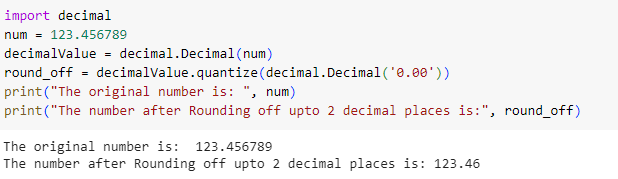
So these were the 4 methods to round numbers to 2 decimals in Python.
Conclusion
Sometimes we need to round a floating point number or maybe an integer up to 2 decimal places. There are 4 methods for doing this; the first method is by using the round() function, the second is by using the format() function, the third is by using the ceil() function, and the last one is by using the decimal module. In this post, we have learned about all these methods with examples.