PyTorch is the open-source library for building Artificial Intelligence models using Machine Learning (ML) or Deep Learning (DL) algorithms. Tensors are the data structures used in PyTorch to store, manage, index, and extract data for training these models. Its libraries are easily accessible in the Python programming language and are highly popular in different fields like Data Science, Analysis, Artificial Intelligence, etc.
Quick Outline
This guide explains the following sections:
- How to Convert a PyTorch Tensor to a List
- Prerequisites
- Method 1: Using tolist() Function With ones Tensor
- Method 2: Using tolist() Function With Customized Tensor
- Method 3: Using numpy() Function
- Method 4: Using For Loop
- Conclusion
How to Convert a PyTorch Tensor to a List
Tensor is more specifically used to organize and analyze data to optimize the performance of the ML or DL models. Sometimes, the user needs to get the data in different formats as the tensors are mostly used in multi-dimensional formats. PyTorch enables the user to convert the tensors into lists using simple methods such as tolist(), numpy(), etc.
To learn the process of converting a PyTorch tensor to a list in the Python programming language, go through the listed steps, and get the code from here:
Prerequisites
Some tasks are required to be completed before converting the PyTorch tensors to a list which are mentioned as follows:
- Access Python Notebook
- Install Modules
- Import Libraries
Access Python Notebook
To write the code for the process in Python, we need to create a new notebook by clicking on its button from the Google Colaboratory official web page:
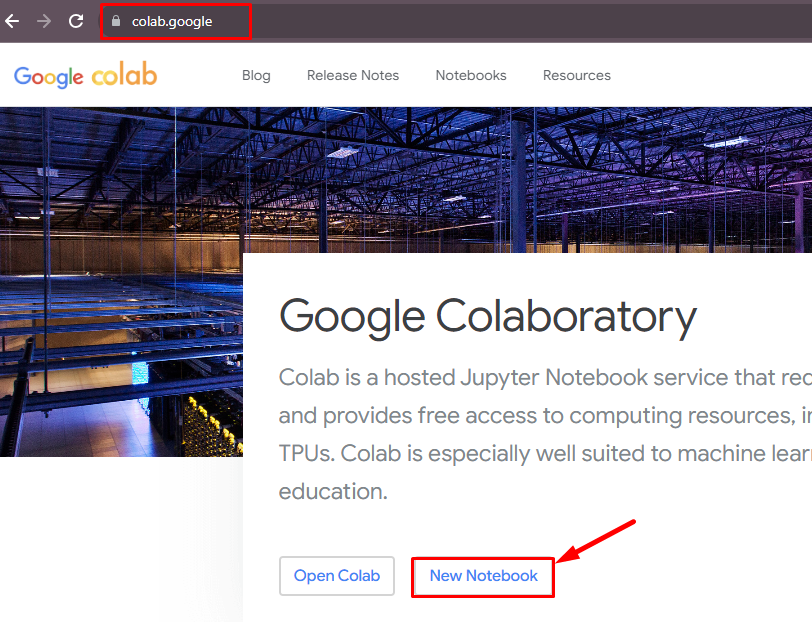
Install Modules
Install the numpy module using the following code to get its libraries to convert PyTorch tensors:
pip install numpy
Install the torch module as well to use the functions offered by the framework:
pip install torch
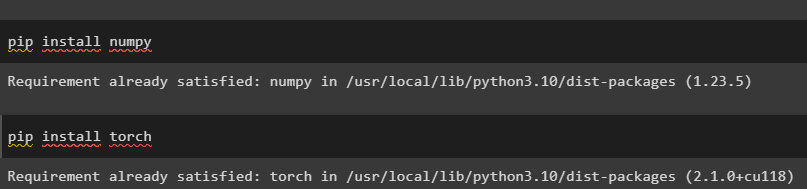
Import Libraries
After that, import the torch library to create tensors and convert them to the lists:
import torch
To display the installed version of the torch, execute the following code in the Python notebook:
print(torch.__version__)
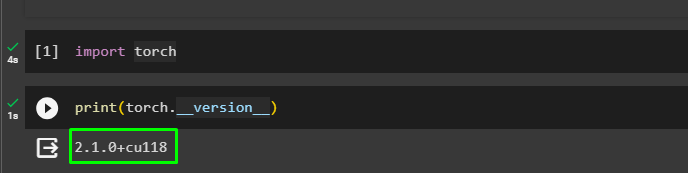
Import the numpy library as this guide will explain the process of using its method for converting the tensors to lists:
import numpy

Method 1: Using tolist() Function With ones Tensor
The simplest method offered by PyTorch is the tolist() method for converting the tensors to lists. Create a tensor using the torch.ones() method and it will create the tensor with all ones stored in it in the structure set in its argument:
py_tensor = torch.ones(2, 3, 4)
Display the data stored in the tensor by calling the print() method:
print(py_tensor)
Get the type of the data stored in the py_tensor variable:
type(py_tensor)
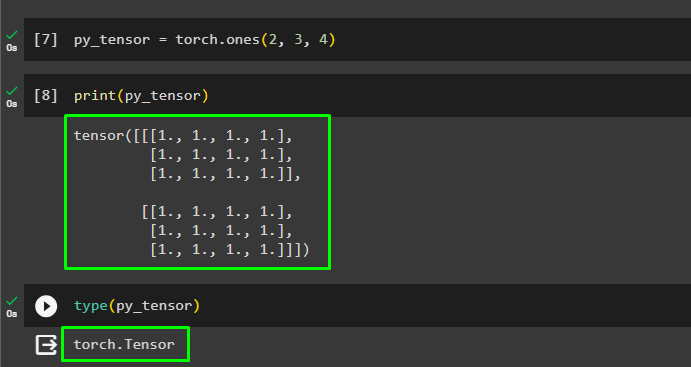
Now, convert the tensor to a list using the tolist() method with the py_tensor variable and store it in the “pylist” variable:
pylist = py_tensor.tolist()
Display the values stored in the pylist variable:
print(pylist)
Getting the data type of the variable using the type() method with the name of the variable:
type(pylist)
The following screenshot displays that the tensor has been converted to the list successfully:
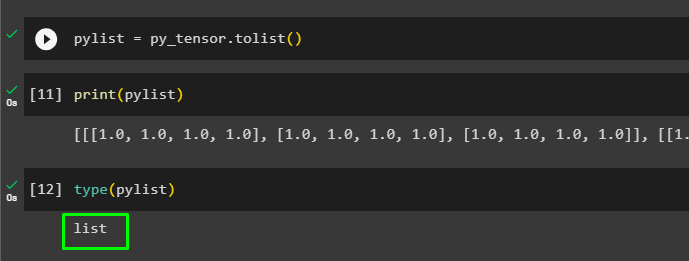
Method 2: Using tolist() Function With Customized Tensor
Previously we used the built-in methods for creating the tensor and this section creates the customized with the values of the user’s choice. Use the torch.tensor() method to create the tensor in PyTorch using the following code:
tensor_py = torch.tensor(
[
[
[9.1, 5.2, 2.3],
[6.4, 8.5, 1.6],
[4.7, 3.8, 6.9]
],
[
[5.1, 6.2, 7.3],
[3.4, 2.5, 1.6],
[1.7, 9.8, 5.9]
]
]
)
Confirm that the list is converted to the tensor using the type() method:
type(tensor_py)
The tensor has been created successfully and stored in the tensor_py variable:
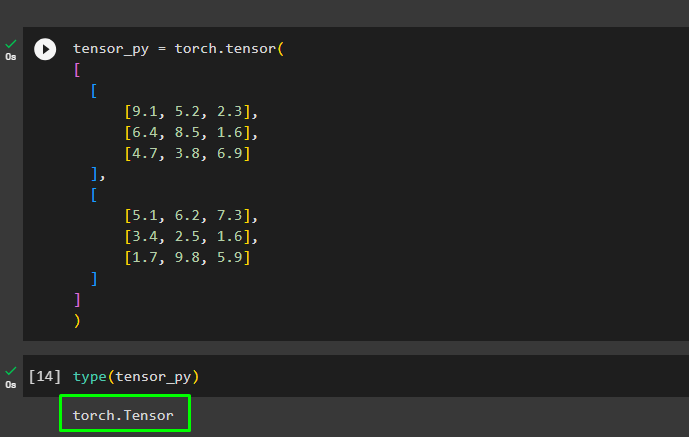
Use the tolist() method to convert the tensor to the list and store it in the list_py variable:
list_py = tensor_py.tolist()
Display the values stored in the list_py variable:
print(list_py)
Get the data type to confirm that the conversion is successful:
type(list_py)
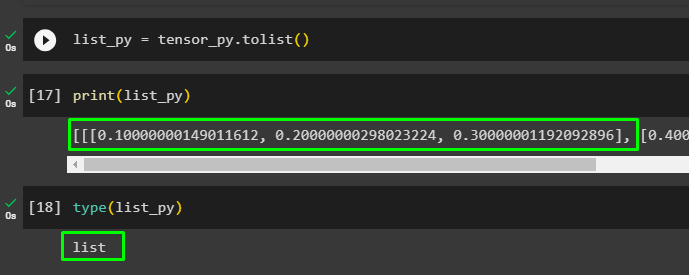
Get the exact value by giving the location as the dimensions of the list:
list_py[0][1][2]
Get the data type of the specific value stored in the list:
type(list_py[0][1][2])
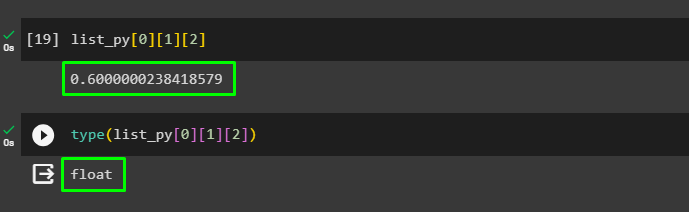
Method 3: Using numpy() Function
The third approach to convert the tensor to the list is using the numpy() method with the tolist() method:
type(tensor_py)
Use the numpy() method for converting the tensor to the list and then print the list as well:
list = tensor_py.numpy().tolist()
print(list)
Print the type of the list after the conversion to confirm the success of the process:
print(type(list))
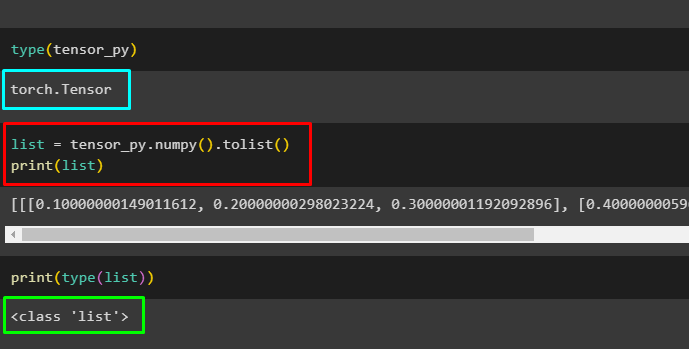
Method 4: Using For Loop
Another method to convert the tensor to the lists is using the for loop in the Python language. The loop gets the values from the tensor and stores them one by one in the form of a list. The first step here is to create a tensor using the following code:
tensor = torch.tensor([1,2,3,4,5])
Display the data type of the data stored in the tensor variable:
type(tensor)
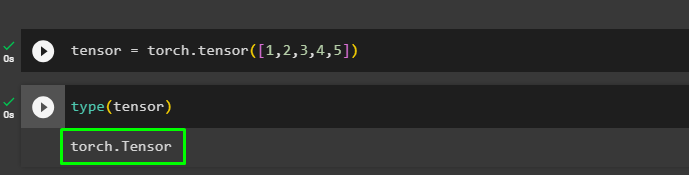
Use the following code to store the tensor in the form of the lists and print the values after its conversion:
new_list = []
for t in tensor:
new_list.append(t.item())
print(new_list)
Display the data type of the new_list variable:
print(type(new_list))
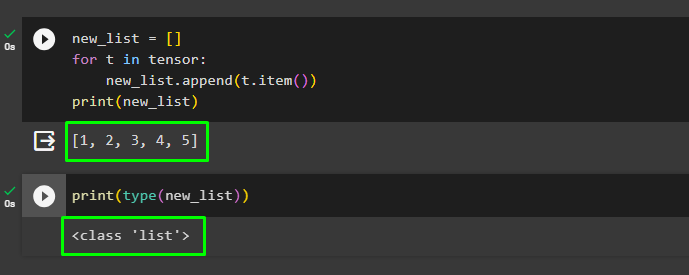
That’s all about the process of converting the PyTorch tensor to a list.
Conclusion
To convert the PyTorch to a list, the framework offers multiple methods like tolist(), numpy(), and a for loop. The PyTorch framework allows the user to build the tensors called user-defined and the built-in templates as well. The user creates a tensor and uses one of the above-mentioned methods to convert it to the list. This guide has elaborated on all the methods of converting the tensors to the lists with their implementations using Python code.