The user data is usually stored in “Collections” like “Set” and “List” at the backend to preserve data for a longer time without damaging the order. Both collections are represented by the “java.util” package in Java and the user must include the package to use these collections.
The “Set” is a Collection type that stores unique elements and it can be implemented using “HashSet”, “TreeSet”, or “LinkedHashSet”. On the other hand, the “List” can contain “null” or duplicated values and the selection of a single List element is straightforward and fast due to its “index-based” ordered nature of storing the data.
This guide covers the following sections:
- Differ Between Set and List
- How to Convert Set to List in Java?
Differ Between Set and List
Although both Set and List are collections there are some differences between them, which are shown below in the tabular format:
Set | List |
---|---|
Duplication of elements is not allowed. | Duplication of elements is allowed |
Data is stored in an unordered manner. | Elements are stored in insertion order. |
Elements cannot be accessed using Index. | Each element is stored at a unique index and they are easily accessed using their corresponding index. |
Generally faster in storing the data. | Best for searching the element or performing the operation on a specific element. |
It can be implemented using HashSet, LinkedHashSet, or TreeSet. | It can be implemented using ArrayList, LinkedList, and many more. |
How to Convert Set to List in Java?
The “Set” is converted into a “List” to store the data in an ordered manner and allow space for “null” and duplicated elements. The conversion is also performed for fast accessing of elements, as the time complexity of searching elements in “Set” is much greater than the “List”. The methods or approaches that can be used for conversion are mentioned and explained below:
- Method 1: Convert Set to List Using “List.addAll()” Method in Java
- Method 2: Convert a Set to a List by Passing the Set as a List Constructor Argument
- Method 3: Convert a Set to a List Using Java 8 Stream API
- Method 4: Convert Set to List Using “copyOf()” Method
- Method 5: Convert Set to List Using Brute Force or Naive Method
- Method 6: Convert Set to List Using Guava
Method 1: Convert Set to List Using “List.addAll()” Method in Java
The “addAll()” method of List adds multiple provided values into the List at once and is often used to duplicate or transfer the data between lists. This method also selects the values stored in a set and places that data into the targeted list without any lossage of data.
Syntax
The “addAll()” method syntax is shown below:
collect.addAll(items)
In the above syntax:
- The “collect” can be any collection type like “ArrayList”, “LinkedList”, or simple “List” in which the data needs to be inserted.
- The “items” are the collection elements that need to be inserted.
Take a look at the practical implementation of converting a Set to a List via the “addAll()” method:
import java.util.*;
class setToList {
public static void main(String args[])
{
Set<String> selectedSet = new HashSet<String>();
selectedSet.add("Article");
selectedSet.add("by");
selectedSet.add("Javabeat");
System.out.println("\nOrignal Set Elements: " + selectedSet);
//Convert Set to List
List<String> targetedList = new ArrayList<String>();
targetedList.addAll(selectedSet);
System.out.println("\nThe Converted Set Elements Into List: ");
for (int a = 0; a <targetedList.size(); a++)
{
System.out.println("Element in List Residing at index no: " + a + " is: " + targetedList.get(a));
}
//Convert Set to LinkedList
LinkedList<String> targetLinkedList = new LinkedList<String>();
targetLinkedList.addAll(selectedSet);
System.out.println("\nThe Converted Set Elements Into LinkedList: ");
for (int a = 0; a <targetLinkedList.size(); a++)
{
System.out.println("Element in Linked List Residing at index no: " + a + " is: " + targetLinkedList.get(a));
}
}
}
In the above code block:
- First, import the “java.util” package and create a “main()” method inside the “setToList” class.
- Next, create a “String” type Set named “selectedSet” using the “HashSet” class. Insert random elements in this Set via the “add()” method and print the Set on a console.
- Then, create a String type “List” named “targetedList” and assign it the elements of “selectedSet” using the “addAll()” method. After that, use the “for” loop to print all elements of the “targetedList”.
- Also, create a “LinkedList” named “targetLinkedList” and use the same “addAll()” method to convert Set into “LinkedList”.
- Finally, iterate over the “targetLinkedList” using a “for” loop to print the residing elements on the console.
The output of the mentioned code is shown below:
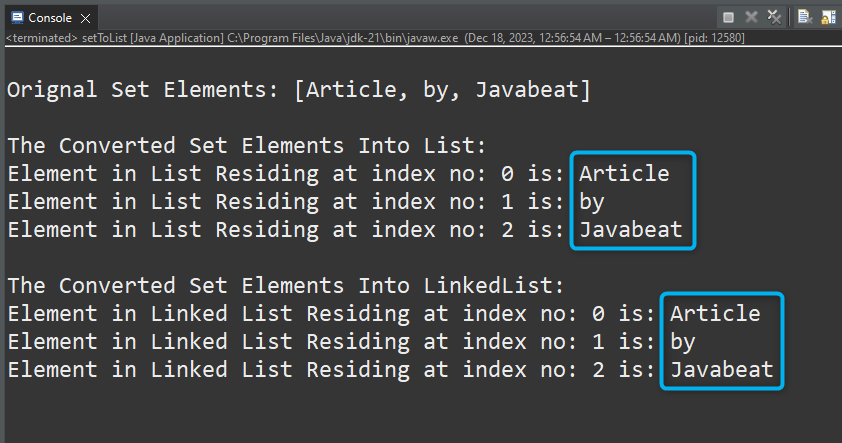
Method 2: Convert a Set to a List by Passing the Set as a List Constructor Argument
The simplest and least consuming line of code method to convert the set to a list is by setting the “Set” as an argument for the “List” constructor. The “List” constructor then by default selects and inserts the “Set” elements into the “List” during the initialization process:
import java.util.*;
public class setToList {
public static void main(String args[])
{
Set<String> selectedSet = new HashSet<String>();
selectedSet.add("Article");
selectedSet.add("by");
selectedSet.add("Javabeat");
System.out.println("\nOrignal Set Elements: " + selectedSet);
//Convert Set to List
List<String> targetedList = new ArrayList<String>(selectedSet);
System.out.println("\nThe Converted Set Elements Into List: ");
for (int a = 0; a <targetedList.size(); a++)
{
System.out.println("Element in List Residing at index no: " + a + " is: " + targetedList.get(a));
}
}
}
The explanation of the above code is written below:
- First, import the “java.util” library and create a set named “selectedSet” containing random items, as described in the above section.
- Next, create a List named “targetedList” and pass the “selectedSet” as an argument for the constructor. To store or convert the Set data into a List.
- After that, use the “for” loop that iterates till the “targetedList” size which is retrieved using the “size()” method.
- Finally, apply the “get()” method over the List to select each residing element of the List and display them on the console.
The output is shown as:
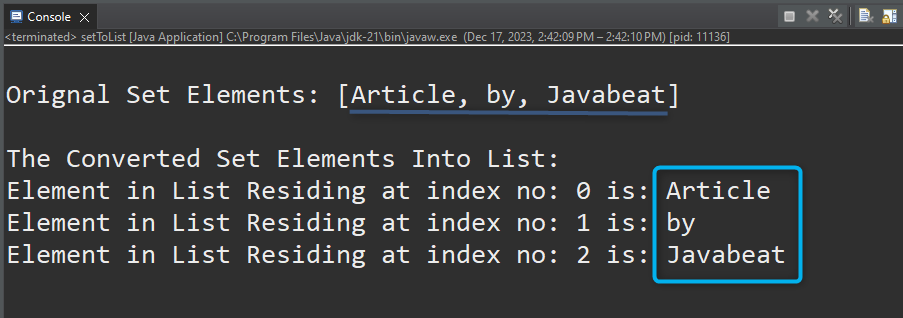
Method 3: Convert a Set to a List Using Java 8 Stream API
The Stream API works only at Java version 8 or more, it offers a method “Stream.collect()” to perform various operations like conversion over the Sets or Lists. According to our requirements, the user can either use the “toList()” or “toCollection(ArrayList::new)” methods. The second method provides more control over the returned List while guaranteeing the type, mutability, or thread-safety of the returned List:
import java.util.*;
import java.util.stream.Collectors;
public class setToList {
public static void main(String args[])
{
Set<String> selectedSet = new HashSet<String>();
selectedSet.add("Article");
selectedSet.add("by");
selectedSet.add("Javabeat");
System.out.println("\nOrignal Set Elements: " + selectedSet);
//Convert Set to List
List<String> targetedList = selectedSet.stream().collect(Collectors.toList());
// 2nd Approach //List<String> targetedList = selectedSet.stream().collect(Collectors.toCollection(ArrayList::new));
System.out.println("\nConverted Set Elements Into List Using Stream API: ");
for (int a = 0; a <targetedList.size(); a++)
System.out.println("Element in List Residing at index no: " + a + " is: " + targetedList.get(a));
}
}
The description of the above code is as follows:
- Initially, import the “stream.Collectors” package and create a Set named “selectedSet” just like done in the above sections.
- Next, apply the “collect()” method of a “stream()” API over the “selectedSet” and pass the “Collectors.toList()” method as an argument.
- As an alternative, you can apply the “toCollection()” method over the “Collectors” and pass the “ArrayList::new” as a method parameter. To get a better mutability or thread-safety of the returned List.
- It will convert the provided Set over the Stream into a “List” collector and store the result in another List named ”targetedList”.
- The elements residing in the generated List are then displayed on the console using the “for” loop.
Output for the provided code confirms the conversion of Set into List using Java 8 Stream API:
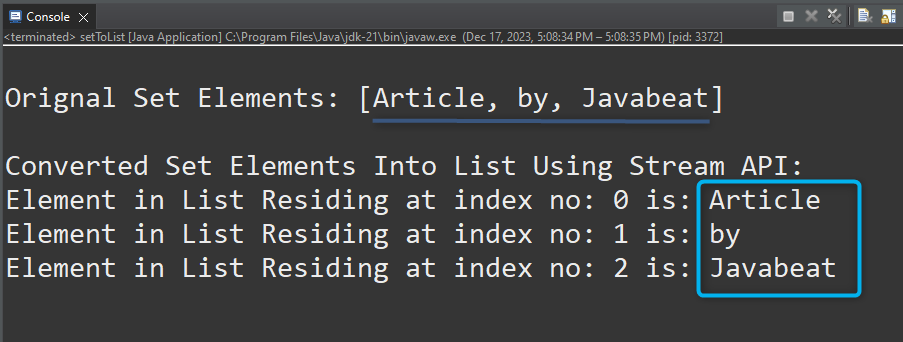
Method 4: Convert Set to List Using “copyOf()” Method
The “copyOf()” method of List allows users to copy the element of other collections like List or Set into the selected List:
import java.util.*;
public class setToList {
public static void main(String args[])
{
Set<String> selectedSet = new HashSet<String>();
selectedSet.add("Article");
selectedSet.add("by");
selectedSet.add("Javabeat");
System.out.println("\nOrignal Set Elements: " + selectedSet);
//Convert Set to List
List<String> targetedList = List.copyOf(selectedSet);
System.out.println("\n\nConverted Set Elements Into List Using copyOf() Method: ");
for (int a = 0; a <targetedList.size(); a++)
System.out.println("Element in List Residing at index no: " + a + " is: " + targetedList.get(a));
}
}
Explanation for the above code is as follows:
- First, define a Set named “selectedSet” and print its residing elements on the console.
- Next, copy the elements of “selectedSet” into “targetedList” by invoking the “List.copyOf()”.
- In the end, display the elements of “targetedList” on the console window similar to the mentioned sections.
The output is shown below:
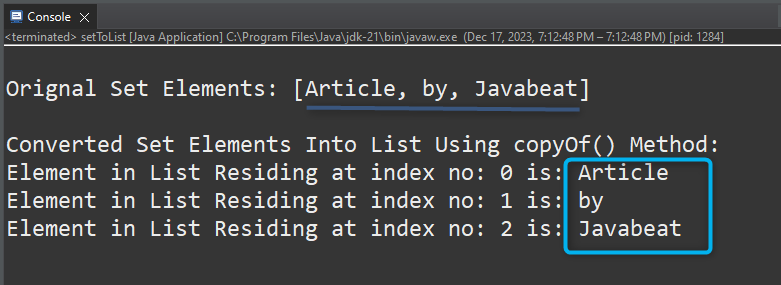
Method 5: Convert Set to List Using Brute Force or Naive Method
The “Brute Force” method to convert a Set into a List is by iterating over each list element and then copying them into the List one by one. The addition of an element in the List is performed by the usage of an “add()” method:
import java.util.*;
public class setToList {
public static void main(String args[])
{
Set<String> selectedSet = new HashSet<String>();
selectedSet.add("Article");
selectedSet.add("by");
selectedSet.add("Javabeat");
System.out.println("\nOrignal Set Elements: " + selectedSet);
List<String> targetedList = new ArrayList<>();
System.out.println("\nConverted Set Elements Into List: ");
for (String listItem : selectedSet) {
targetedList.add(listItem);
}
System.out.println(targetedList);
}
}
In the above code block:
- First, create a Set named “selectedSet” and insert random elements in it.
- Then, declare a String type List named “targetedList” using the “ArrayList” class.
- After that, utilize the enhanced “for” loop that iterates over the “selectedSet”.
- Also, use the “add()” method to iterate each element into “targetedList” and print the List on a console.
The output for the above code is shown as:
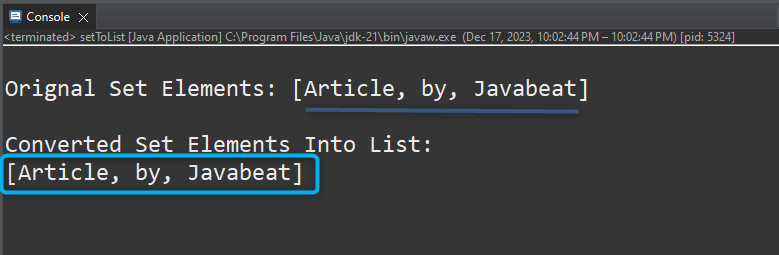
Method 6: Convert Set to List Using Guava
The “Guava” is an open-source library developed and handled by Google. It enhances the Java standard libraries or methods by providing quality utilities and more specific methods to deal with most tasks, like converting Set to List. The “Guava” library can be downloaded from the “Guava” repository, once downloaded you have to set its “Built Path” for your project. Once, completed you can insert the below code to perform the desired operation of converting Set to List:
import java.util.*;
import com.google.common.collect.Lists;
public class setToList {
public static void main(String args[])
{
Set<String> selectedSet = new HashSet<String>();
selectedSet.add("Article");
selectedSet.add("by");
selectedSet.add("Javabeat");
System.out.println("\nOrignal Set Elements: " + selectedSet);
List<String> targetedList = Lists.newArrayList(selectedSet);
System.out.println("\nConverted Set Elements Into List Using add() Method: ");
System.out.println(targetedList);
}
}
The output is shown as follows:
- First, import the “common.collect.Lists” package and create a class named “setToList”.
- In addition, a Set having the type of String named “selectedSet” is declared and initialized with random values.
- Then, pass this Set as an argument in the “newArrayList()” method invoked by the Guava “Lists” class.
- Store the result in “targetedList” which is then displayed on the console.
The output shows the conversion of Set into List using Guava Library:
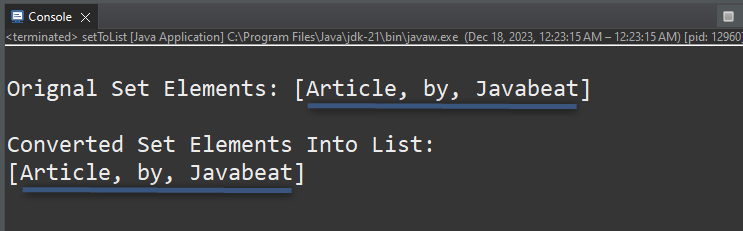
That’s all about the conversion of Set to List in Java.
Conclusion
The conversion of Set to List is performed by the usage of “List.addAll()”, “copyOf()”, Java 8 Stream API “Collectors.toList()” methods. In addition, the user can pass the Set as a List constructor argument or iterate over the set element and add each element into the List using an “add()” method. Moreover, the “Lists.newArrayList()” method provided by an external library named “Guava” is also used to perform the mentioned task. This guide has successfully explained the conversion of Set to List in Java.