Folders are required to save the information so that the location of files becomes easy when retrieving. The folders tend to organize the information on the device. If the folders are not created, the files seem to be unorganized and mismanaged. Not only this the retrieval of files becomes a difficult task. Every programming language requires a folder to save the files and locate these files when needed.
In this write-up, we will go through methods to create a folder in Python.
How to Create a Folder in Python?
To create a folder in Python, the first step involves deciding where the folder is to be created. When the location is decided the os module of the Python is imported to design a folder at the specified location. There are two basic functions to create a folder in Python that are os.mkdir() and os.mkdirs().
Method 1: Create a Folder Using os.mkdir() Function
The os.mkdir() function of Python creates a folder in the specified path. Below is the code implementation of the function.
# importing os module
import os
# Folder name
folder = "Python 1"
# The path where the folder is to be created
main = "C://"
# Path
path_specified = os.path.join(main, folder)
os.mkdir(path_specified)
# Check if the folder does not already exist
if not os.path.exists(folder):
os.mkdir(folder)
print("Folder '% s' is created" % folder)
else:
print(f"Folder '{folder}' already exists.")
In the above code:
- The os module of Python is imported.
- In the next step, a folder is created as “Python 1”.
- The location of the folder is specified in C:// drive.
- The join() method combines the location with the folder name.
- In the last steps, the for-else loops print whether the folder is created or it already exists in the specified location.
Output
In the output below, it is observed that the folder “Python 1” is created in local disk C://. Python shell also confirms the creation of the folder as “Folder ‘Python 1’ is created”.
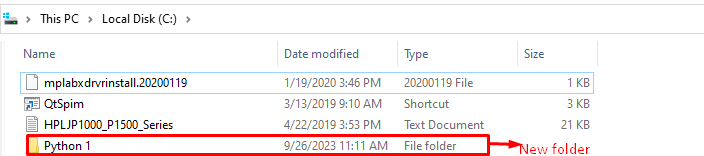

If the folder with the same name is created it throws an error that the folder of the file already exists.

Method 2: os. mkdirs() Method
The os.mkdirs() method creates the parent directory if required. The code below creates a folder directly, without separately specifying the location and the new folder.
#Import the os module
import os
#Give the path for the folder to be created
path_specified = "D://app//Python1"
if not os.path.exists(path_specified):
#makedirs() method
os.makedirs(path_specified)
print(f"The Created folder '{path_specified}'.")
else:
print(f"The Folder '{path_specified}' already exists.")
In the above block of code, The folder “Python1” is declared with the main or the parent folder that is “app”. The os.mkdirs() method takes the path specified and creates a new folder.
Output
In the output below it is observed that the new folder is created in the D drives folder “app”.


If the code is executed again or the folder with the same name is declared, the output shows that the “Python1” folder already exists.

This concludes the implementation of methods to create a new folder in Python.
Conclusion
To create a new folder in Python the path of the folder is to be specified using two different methods that are os.mkdir() and os.mkdirs(). In this article, we have implemented these methods to create a folder in Python.