Getting familiar with the data types can be really hectic in Python programming, the type of the variable is not defined in an explicit manner. Getting to know a variable’s type can be really helpful for beginner coders as they do not need to cram each datatype and can be helpful in catching the errors in a code. There are built-in methods in Python that can get the type of a variable.
This article discusses different methods to determine the Python Variable’s type and discusses its relevant examples.
How to Determine a Python Variable’s Type?
In Python, variables can hold different kinds of data and sometimes it is necessary to know the data type the variable holds. There are different data types like integer, tuple, set, and more. Python has different built-in variables that can get the variable’s type. A variable’s type can be determined using:
- Using the type() method
- Using the isinstance() method
- Using the __class__ attribute
Let us discuss each method to know the Python variable’s type in detail.
Method 1: Using the type() Method
The type() function in Python is a built-in method that returns the type of the function. The type of the element refers to the data structure. The type method returns the object’s type. It can take and return any data type. The syntax of the type() function with a single parameter is:
type(obj)
- type function gets the type of the Python’s object
- obj determines the Python’s object whose type is to be found
The type() function with three parameters is:
type(obj, bases, dict)
- obj defines the Python object whose type needs to be found.
- bases represent the __base__ attribute. It is a tuple from which the present class is derived.
- dict represents the __dict__ attribute. It saves the namespaces for the class and assists in creating the base class.
Let us get started with different examples that use the type() function.
Example 1: type() Method With a Single Parameter
The following example explains how to get a type of a variable when a single variable is provided:
def find_type(var):
return print('The type of the given input is:',type(var))
find_type([1,3,4])
find_type({2,3,4})
find_type('Tea')
find_type(0)
find_type(('rose', 'jasmine'))
find_type({'Apples':3,'Kiwis':10})
find_type(28.3)
In the above code,
- The function find_type gets the parameter var. The function prints the type of the variable provided.
- The function is checked for different data structures.
Output
The output displays the type of different data structures provided to the find_type() function that uses the type() method to print the type of input data:
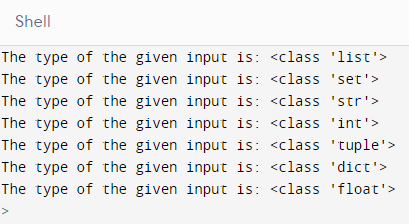
Example 2: type() Method With Three Parameters
The type() method with three parameters can be used to dynamically create classes. A class can be created with no base class or the class can be derived from a Parent class. The attributes of the class can be defined at the time of execution. The following code explains how to create a class without a base class or its attributes are derived from a base class:
new_class = type('New_Class', (object, ),
dict(var1='Coding is fun.', year=2009))
print(type(new_class),'\n')
print(vars(new_class),'\n')
class test:
str = "Coding is fun."
year = 2009
updated_class = type('Updated_Class', (test, ),
dict(x='Code', y=2018))
print(type(updated_class),'\n')
print(vars(updated_class))
In the above code,
- A class named new_class is created using the type() function. The name of the class is New_Class with no base class. The third parameter is a dictionary that contains class attributes var1= ‘Coding is fun.’ and year=2009.
- The type of the new_class is printed which will be type indicating that it is an instance of the class type.
- The vars(new_class) are printed that will return a dictionary containing the attributes of the new_class and their respective values.
- A base class test is defined with two attributes str=’Coding is fun.’ and year=2009.
- Another class updated_class is created using the type() method which is derived from its parent class test. The third parameter is a dictionary with the class attributes x=’Code’ and y=2018.
- The type, attributes, and their respective values are printed.
Output
The output displays the usage of the type() function with three parameters to dynamically create classes in Python:
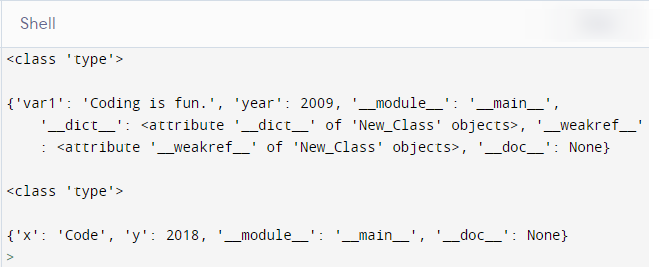
Example 3: Verifying the Type of a Python Object Using type()
The type of a Python variable can be verified using the type() method. The variable is checked against different conditions. In the following example, type(5) is not int will give the boolean value of False since it is an integer, and type(3.33) is float will give the bool value True since 3.33 is a float value with a decimal part. The following code explains the usage of the type() method to verify the Python variable’s type:
print(type(5) is not int)
print(type(22.2) is tuple)
print(type(3.33) is float)
print(type(3) is not list)
Output
The following output displays how a Python’s variable type can be verified using the type() method in Python:
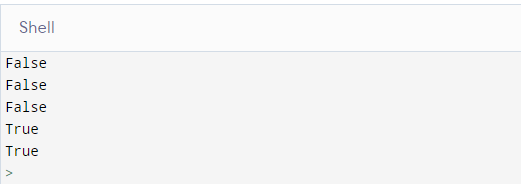
Method 2: Using the isinstance() Method
The Python function isinstance() can be used to check the type of a variable. Two parameters are passed to the isinstance method, the first one is the variable whose type is to be checked and the second is the data type the variable is checked against. The function returns True if the variable has the same data type as in the second parameter and returns False in the opposite case. The following code uses the isinstance() method to check the variable’s type against different data types:
print(isinstance([1,3,4],list))
print(isinstance({2,3,4}, set))
print(isinstance('Tea',str))
print(isinstance(0,int))
print(isinstance(('rose','jasmine'),tuple))
print(isinstance({'Apples':3,'Kiwis':10},dict))
print(isinstance(28.3,float))
Output
The following output displays the usage of the isinstance() method to check the variable’s type against a certain data type:
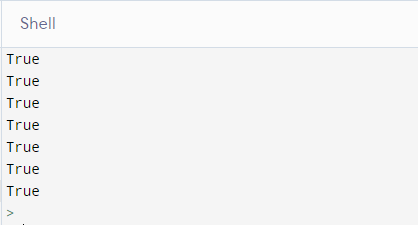
Method 3: Using the __class__ Method
__class__ is a unique attribute in Python that can provide information about the class’s type. The information about the class’s type can be fetched through the instance of the class. The following code explains how to get the variable’s type using the __class__attribute in Python:
def find_class(var):
return print('The input variable belongs to the class type:',var.__class__)
find_class([1,3,4])
find_class({2,3,4})
find_class('Tea')
find_class(0)
find_class(('rose', 'jasmine'))
find_class({'Apples':3,'Kiwis':10})
find_class(28.3)
In the above code,
- A function find_class is defined that gets the parameter var.
- The function prints the type of the variable by calling the __class__method on a variable.
- The function is checked on different inputs.
Output
The following output displays how the __class__ attribute can be used to determine the variable’s type in Python:
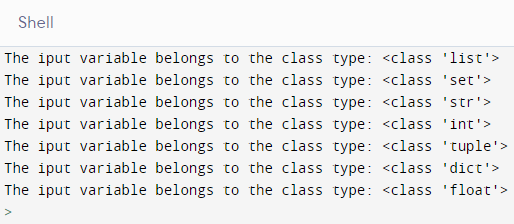
Comparison Between type() and isinstance()
Both functions are used to determine the variable’s type. The type function returns the exact information about the type of the variable’s type. The isinstance function checks if the object belongs to an instance of a particular class or any of its derived classes.
Why is it Important to Determine the Type of the Variable?
Getting to know the type of the variable is useful for the below-stated reasons:
- For debugging purposes
- Finding the argument’s type
- Creating classes and defining their attributes
- Assists in the convenient registering of databases in SQL
Conclusion
In Python programming, the type of the function can be determined by the function type(), isinstance(), and the __class__ attribute. The type of a variable is not directly defined in Python, however, using the built-in functions like type() and instance can get the variable’s type. Beginners can know the type conveniently without the need to remember each data type. The type function is handy to detect any errors in a program so it is helpful in debugging purposes. The article discusses different methods to find the variable’s type