The array is an essential data structure that stores data of the same type in multiple dimensions. It can efficiently manage a large dataset. Sometimes, users may need to compute the length of a specific array for various reasons, such as to iterate through its elements, for indexing, etc. In Python, there are different methods including built-in functions that can be used to calculate the desired array’s length.
This guide will explain different methods to find the length of an array and multidimensional array in Python.
How to Get/Find the Length of an Array in Python?
Finding the length of an array means calculating the total number of elements in it. In Python, different approaches can be used to compute the length of Python arrays, such as
- Approach 1: Get/Find Array’s Length Via “len()” Method
- Approach 2: Get/Find Array’s Length Via “.size” Attribute of NumPy Library
- Approach 3: Get/Find Array’s Length Via “.shape” Attribute of NumPy Library
- Approach 4: Get/Find Array’s Length Via “for” Loop
- Approach 5: Get/Find Array’s Length Via “length_hint()” Method
- Approach 6: Get/Find Array’s Length Via “__len__()” Method
Approach 1: Get/Find Array’s Length Via “len()” Method
The “len()” method is Python’s built-in function that calculates/determines the length of the specific array in Python. It returns the total number of elements present in a Python array. This method can be used to calculate the length of any array whether it is created through square brackets, array module, or numpy module.
Example 1: Use “len()” Method to Find Length of Array Created Through Square Brackets
In this example, we have created a simple “my_array” array through square brackets. We will pass this array as an argument to the “len()” method to calculate its length and print it:
my_array = [1, 7, 3, 8, 4]
print("Array Elements: ", my_array)
arr_length = len(my_array)
print("\nLength of the Array: ", arr_length)
The “len()” function has calculated and returned the length of “my_array” i.e. “5”:
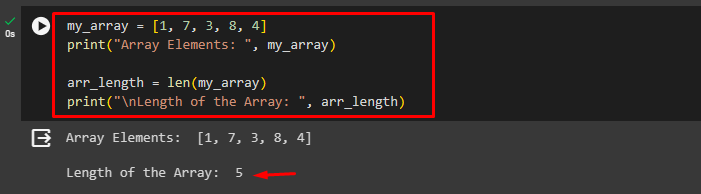
Example 2: Use “len()” Method to Find length of Array Created Through Array Module
In this example, we have imported the “array” module as “Arr” to create an array. We have created the “my_arr” array of the integer type using the “i” type code. We passed this array to the “len()” method to determine and display its length:
import array as Arr
my_arr = Arr.array('i',[9, 7, 5, 4, 3, 1])
print("Array Elements: ", my_arr)
length_arr = len(my_arr)
print("\nLength of the Array: ", length_arr)
The below output displays the length of the “my_arr” array:
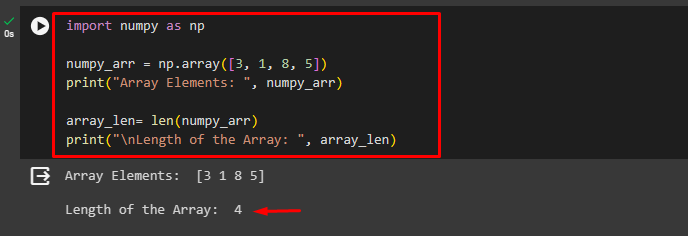
Example 3: Use “len()” Method to Find Length of Array Created Through NumPy Module
Here, we have imported the “numpy” module as “np” to create a NumPy array. We have created the “numpy_arr” array using the numpy module and used it in the “len()” method to find out its length:
import numpy as np
numpy_arr = np.array([3, 1, 8, 5])
print("Array Elements: ", numpy_arr)
array_len= len(numpy_arr)
print("\nLength of the Array: ", array_len)
The below output indicates that the length of the numpy array is “4”:
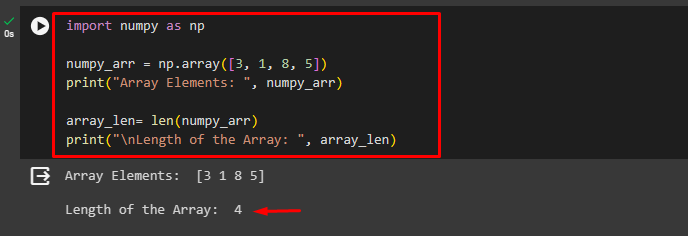
Approach 2: Get/Find Array’s Length Via “.size” Attribute of NumPy Library
Python’s NumPy library has a “.size” attribute that is utilized to compute and return the total number of elements present in the numpy array. To do so, we have imported the “numpy” module as “np”. We have defined the “numpy_arr” NumPy array using the numpy module. Then, we used the “.size” attribute with the “num_arr” array variable to calculate the array’s length:
import numpy as np
numpy_arr = np.array([5, 9, 13, 27, 2])
print("Array Elements: ", numpy_arr)
array_len= numpy_arr.size
print("\nLength of the Array: ", array_len)
The “numpy_arrr.size” has returned the length of the “numpy_arr” array i.e. “5”:
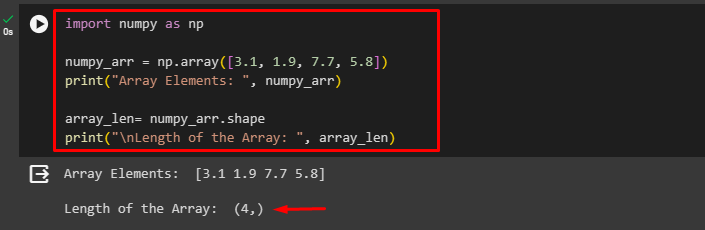
Approach 3: Get/Find Array’s Length Via “.shape” Attribute of NumPy Library
The “.shape” attribute is a NumPy library’s attribute that determines the dimensions (number of rows and columns) of the NumPy array. It can be used to calculate the NumPy array’s length. For instance, we are using the “.shap” attribute with the “numpy_arr” array to find out its length:
import numpy as np
numpy_arr = np.array([3.1, 1.9, 7.7, 5.8])
print("Array Elements: ", numpy_arr)
array_len= numpy_arr.shape
print("\nLength of the Array: ", array_len)
In the below output, the length of the “numpy_arr” array can be seen i.e. “4”:
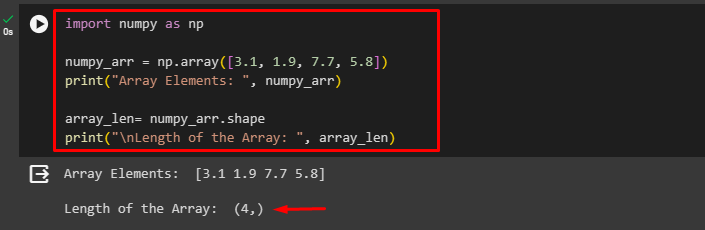
Approach 4: Get/Find Array’s Length Via “for” Loop
The “for” loop is utilized to iterate over all the elements of iterables including arrays. Users can use the “for” loop to find the length of a simple array, NumPy array, or the array that is created through the “array” module.
Example 1: Use “for” Loop to Find Length of Array Created Through Square Brackets
In this example, we have defined the simple “my_array” array using square brackets. We have defined the counter i.e. “count” and set its initial value to “0”. Then, we have used the “for” loop to iterate over the “my_array” elements. The “count+1” will increment the “count” by “1” in each iteration and store the total number of array elements in the “count” variable. The “print()” function will display the total count (array’s length):
my_array = [4, 7, 1, 9]
print("Array Elements: ", my_array)
count = 0
for i in my_array:
count = count+1
print("\nLength of the Array: ", count)
By doing so, the length of the “my_array” array has been calculated as seen below:
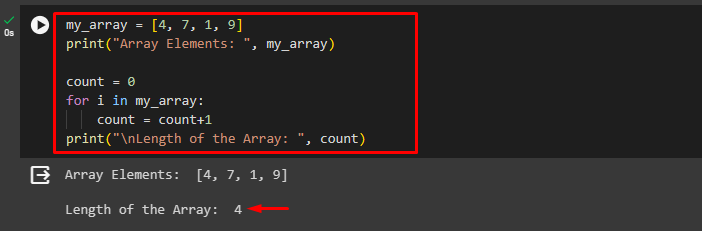
Example 2: Use “for” Loop to Find length of Array Created Through Array Module
Here, we have created a double data type array named as “my_arr” array through the “d” type code. We are using it with the “for” loop to iterate through its elements and compute the total number of elements in it:
import array as Arr
my_arr = Arr.array('d',[9.2, 7.1, 5.8, 4.0, 3.2])
print("Array Elements: ", my_arr)
count = 0
for i in my_arr:
count = count+1
print("\nLength of the Array: ", count)
The below output shows the array’s length:
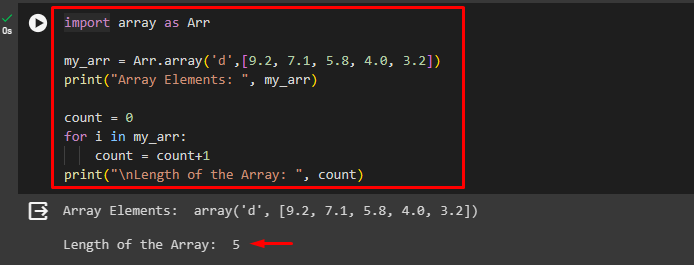
Example 3: Use “for” Loop to Find Length of Array Created Through NumPy Module
In this example, we are using the “numpy_arr” array in the “for” loop to find out the total number of its elements:
import numpy as np
numpy_arr = np.array([5, 2, 9, 34, 1, 26])
print("Array Elements: ", numpy_arr)
count = 0
for i in numpy_arr:
count = count+1
print("\nLength of the Array: ", count)
According to the below output, the length of the NumPy array is “6”:
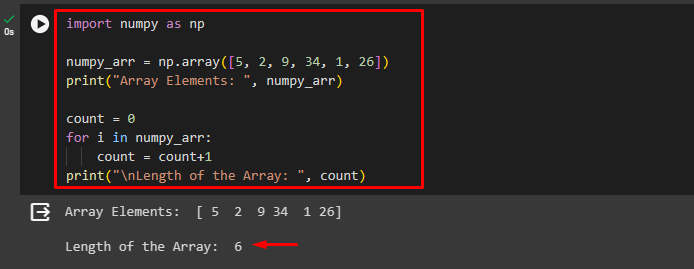
Approach 5: Get/Find Array’s Length Via “length_hint()” Method
Python’s “operator” module provides the “length_hint()” method to calculate the desired iterable’s length. Users can use this method to get the length of Python arrays.
Example 1: Use “length_hint()” Method to Find the Length of Array Created Through Square Brackets
In this example, we have imported the “operator” module. Then, we initialized the simple “my_array” array and passed it to the “length_hint” method to calculate its length:
import operator
my_array = [3, 8, 1, 7, 4]
print("Array Elements: ", my_array)
arr_length = operator.length_hint(my_array)
print('\nLength of Array: ', arr_length)
Subsequently, the array’s length has been calculated:
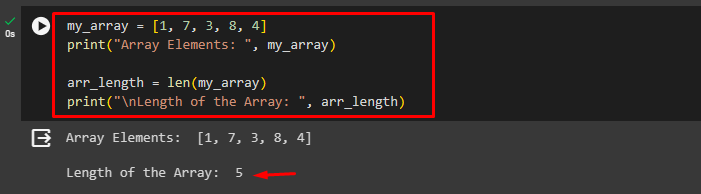
Example 2: Use “length_hint()” Method to Find length of Array Created Through Array Module
Here, we have created the “my_arr” array using the “array” module. Then, we passed it to the “length_hint” method to find the total number of elements in the array:
import array as Arr
import operator
my_arr = Arr.array('i',[9, 7, 5, 4, 3, 1])
print("Array Elements: ", my_arr)
arr_length = operator.length_hint(my_arr)
print('\nLength of Array: ', arr_length)
In the below output, the total number of array elements (array’s length) can be seen:
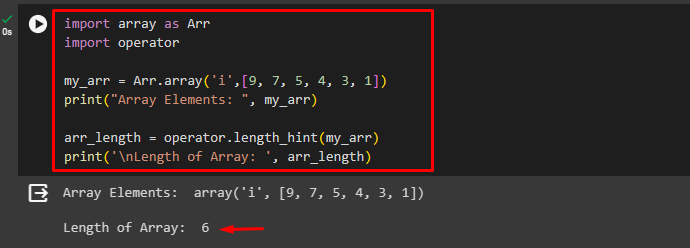
Example 3: Use “length_hint()” Method to Find Length of Array Created Through NumPy Module
In this example, we are calculating the length of the “numpy_arr” NumPy array with the help of the “length_hint” method:
import numpy as np
import operator
numpy_arr = np.array([5, 10, 0, 3, 1, 8, 2])
print("Array Elements: ", numpy_arr)
arr_length = operator.length_hint(numpy_arr)
print('\nLength of Array: ', arr_length)
The below output displays the NumPy array’s length:
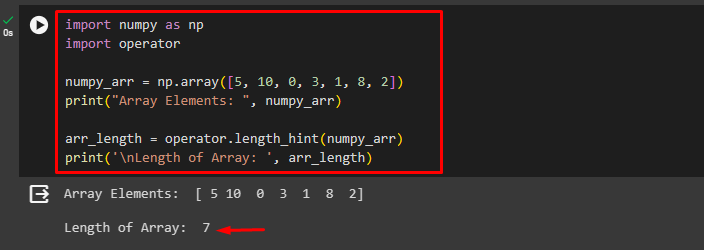
Approach 6: Get/Find Array’s Length Via “__len__()” Method
The “__len__()” method works the same as the “len()” method. When we use the “len()” method, it actually calls the “__len__()” method and returns the length of the desired Python array.
For instance, we have the “my_array” array and we are calculating its length by using it with the “__len__()” method:
my_array = [1, 7, 3, 8, 4]
print("Array Elements: ", my_array)
arr_length = my_array.__len__()
print("\nLength of the Array: ", arr_length)
Subsequently, the length of the specified array has been computed as seen below:
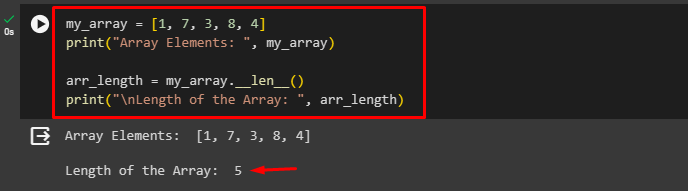
How to Get/Find the Length of Multidimensional Array in Python?
Multidimensional arrays are arrays that have numerous dimensions. Users can have multiple rows and columns in the array. To find the length of the multidimensional array in Python, the following methods are used:
- Method 1: Get/Find the Length of the Multidimensional Array Via “.size” Attribute
- Method 2: Get/Find the Length of the Multidimensional Array Via “.shape” Attribute
Method 1: Get/Find the Length of the Multidimensional Array Via “.size” Attribute
To get the length of a multidimensional array, use the “.size” attribute with the desired multidimensional array variable. For instance, we have the “arr1” multidimensional array and we are using it with the “.size” attribute to compute its length:
import numpy as np
arr1 = np.array([[4, 7, 1], [2, 4, 8], [1, 3, 7]])
print("Array Elements: \n", arr1)
arr_length = arr1.size
print("\nLength of the Array: ", arr_length)
The “.size” attribute calculated the multidimensional array’s length i.e. “9”:
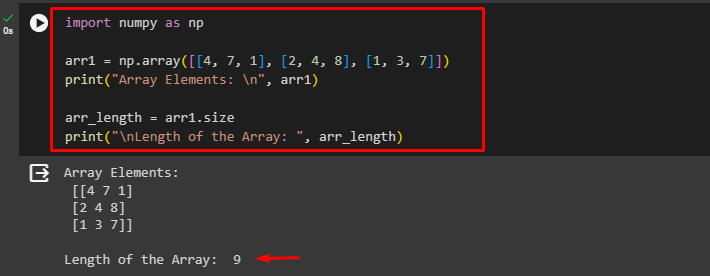
Method 2: Get/Find the Length of the Multidimensional Array Via “.shape” Attribute
The “.shape” method can also be used to get the multidimensional array’s length. It returns the number of rows and columns in the array. Here, we have the “arr1” multidimensional array and we are calculating its length using the “.shape” attribute:
import numpy as np
arr1 = np.array([[4, 7, 1], [2, 4, 8], [1, 3, 7]])
print("Array Elements: \n", arr1)
arr_length = arr1.shape
print("\nLength of the Array: ", arr_length)
According to the below output, the “arr1” array contains “3” rows and “3” columns and the total number of elements is 9:
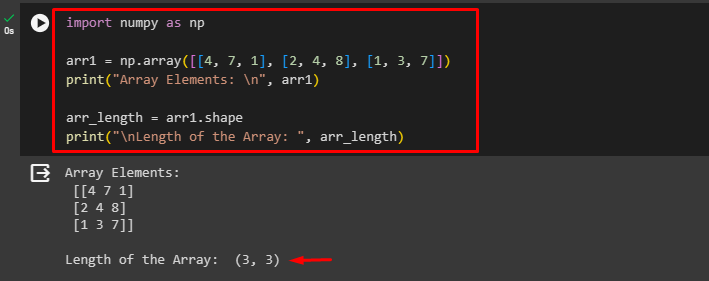
Note: To access our Google Colab Notebook, visit the provided link.
Conclusion
In the Python programming language, various approaches can be utilized to compute the length of Python arrays. To get the specific array’s length, users can use the “len()” method, “length_hint()” method, “__len__()” method, “.size” or “.shape” attribute of NumPy library or “for” loop. However, the “len()” method is the easiest and most efficient method to calculate the length of the desired Python array. This guide has explained different methods to find the length of an array and multidimensional array in Python.