In the Python programming language, the logical operators are essential operators that enable users to make some decisions based on specific conditions. These operators are used for testing various conditions such as checking if a particular statement is true or false, if a user input is valid or not, etc. Among them, the most popular logical operators is the “or” operator. With the help of the “or” operator, users can check for multiple conditions in Python.
Quick Outline
This blog will explain the working of the “or” operator in Python using the following outline.
- What is “or” Operator in Python?
- How to Use “or” Operator in Python?
- What is Bitwise OR “|” Operator in Python?
- Comparison Between Logical “or” Operator and Bitwise OR “|” Operator in Python
What is “or” Operator in Python?
In Python, the “or” operator is one of the logical operators that is used to test or check whether any of two conditions is True. The “or” operator returns “True” when either or both conditions are True. If both conditions are False, it returns “False”.
Flowchart of “or” Operator
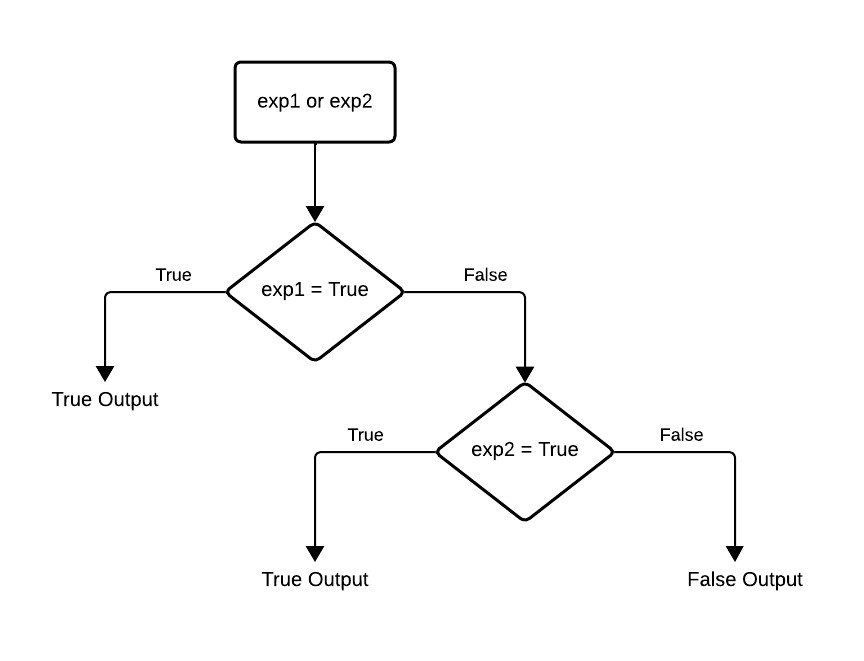
This flowchart explains the “or” operation on “exp1” and “exp2”. This will first check for “exp1”. If the “exp1” is “True”, it will return the “True” output, else it will check for “exp2”. If the “exp2” is “True” it will return a “True” output, otherwise, the “False” output will be returned.
Truth Table for “or” Operator
Input | Output | |
Expression 1 | Expression 2 | Expression1 or Expression 2 |
True | True | True |
True | False | True |
False | True | True |
False | False | False |
The above truth table shows that if either of the expressions is true, the output will be “True”. The output will be “False” only when both expressions are false.
Practical Implementation of “or” Operator in Python | Short Circuit
The “or” operator evaluates the given expression until it finds the “True” value and does not evaluate the rest of the expression.
Here, we have defined two functions i.e “true_fun()” and “false_fun()”. We are evaluating these functions using the “or” operator to see the results:
def true_fun():
print("True Function Running")
return True
def false_fun():
print("False Function Running")
return False
case1 = true_fun() or true_fun()
print("Case 1", case1)
print()
case2 = true_fun() or false_fun()
print("Case 2", case2)
print()
case3 = false_fun() or true_fun()
print("Case 3", case3)
print()
case4 = false_fun() or false_fun()
print("Case 4", case4)
print()
Here:
- “case1” evaluates only the first “true_fun()” expression because it is true so it returns “True”.
- “case2” also evaluates only the first “true_fun()” expression as it is true. The second operand “false_fun()” will not be evaluated and the final output will be “True”.
- “case3” evaluates both expressions because the first “false_fun()” expression is false. So the second “true_func()” expression is evaluated and returns “True”.
- “case4” also evaluates both expressions because both of them are false. The final output will be “False”.
The running functions and outputs can be seen in the below screenshot:
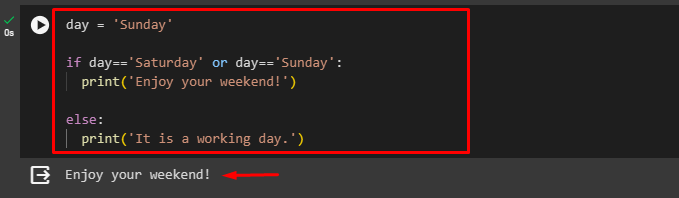
How to Use “or” Operator in Python?
To use the “or” operator in Python, simply write the expression with the desired operands and the “or”
operator. This operator can be used with boolean expressions, non-boolean values, and if-else statements. Users can also use multiple “or” operators and even use the “or” operator with the “and” operator.
Check out the following use cases for a better understanding.
Case 1: Using “or” Operator with Boolean Expression
Boolean expressions are the expressions that check a condition and result in either “True” or “False”. The “or” operator is commonly used with the boolean expression to evaluate them.
Example 1
For example, we have two expressions i.e. “exp1” and “exp2”. First, both of these expressions will be evaluated. The “exp1” will check if 3 is greater than 5 and returns the result “False”. The “exp2” will check if 3 is less than 5 and return “True”. Then, the “or” operator will be applied to the resultant value of these expressions. The final output will be “True” since the “exp1” was “False” and the “exp2” was “True”:
exp1 = 3>5
exp2 = 3<5
result = exp1 or exp2
print(result)
The below output shows the “True” result:
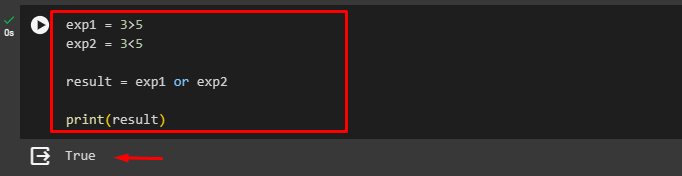
Example 2
In this example, we have set the value of “x” to “2”. We have applied the “or” operator on the different conditions of “x”. The conditions indicate that the value of “x” should be either less than “0” or greater than “20”. Since both conditions are false, the output will be “False”.
x = 2
result = (x < 0) or (x > 20)
print(result)
The “or” operator has returned the “False” output:
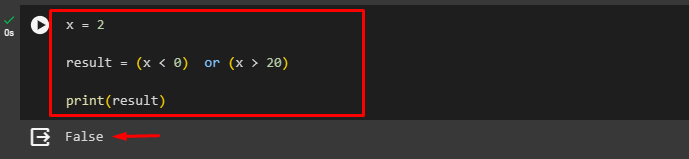
Case 2: Using “or” Operator with Non-boolean Values
Users can also use the “or” operator with non-Boolean values, such as numbers, strings, lists, etc. In such a scenario, the “or” operator retrieves the first operand if it assesses to a truthy value. Otherwise, it returns the second operand. Objects like “None”, “False”, “0”, “()”, “[]”, etc are considered to be False.
Example 1
For example, we have non-boolean values “0” and “1” and we have used the “or” operator with them. This will return the second operand as the first operand is falsy value i.e. “0”:
res = 0 or 1
print(res)
The “or” operator has returned the following output:

Example 2
In this example, we have two strings i.e. “str1” and “str2” and we have applied the “or” operation on them, as a result, the value of the first operand i.e. “Python” will be retrieved because it is a truthy value:
str1 = 'Python'
str2 = 'Programming'
res = str1 or str2
print(res)
The output can be seen in the screenshot below:
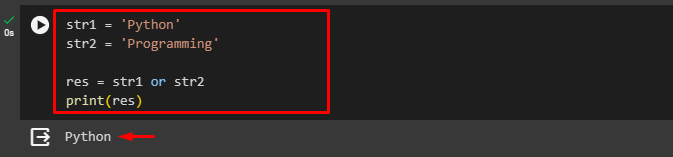
Case 3: Using “or” Operator with if-else Statement
If-else statement is a conditional statement that evaluates the conditions. The “or” operator can be utilized with the if-else statement to compare conditions.
For instance, we have used the “if” statement with the “or” operator to compare the following two conditions. According to the conditions, if the value of “day” is either “Saturday” or “Sunday”, the “if” statement/block will be executed. Otherwise, the “else” statement will be executed:
day = 'Sunday'
if day=='Saturday' or day=='Sunday':
print('Enjoy your weekend!')
else:
print('It is a working day.')
As the value of “day” was “Sunday”, one condition was true. So, the “if” statement has been executed and displayed the following output:
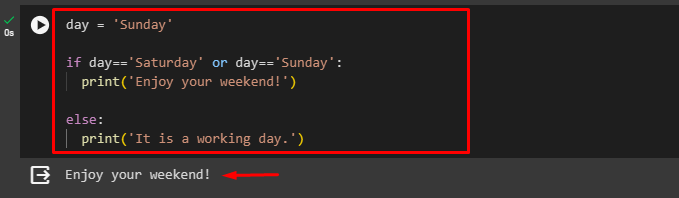
Case 4: Using Multiple “or” Operator with if-else Statement
Users can also use more than one (multiple) “or” operators with if-else statements to evaluate multiple conditions.
Here, we have specified some conditions with the “or” operator inside the “if”. According to the conditions, either the “qualification” is “MS”, “experience” is greater than “2”, or the “category” is “CS”, the block of the “if” statement will be executed. When all the situations are false, the “else” statement will be implemented. As we have set the “qualification” to “MS”, “experience” to “5”, and “category” to “Linux”, two conditions are true. So, the output of the “if” statement will be returned:
qualification = 'MS'
experience = 5
category = 'Linux'
if (qualification == 'MS') or (experience > 2) or (category == 'CS'):
print("You are Qualified")
else:
print("Sorry, Not Qualified")
Subsequently, the following output has been displayed:
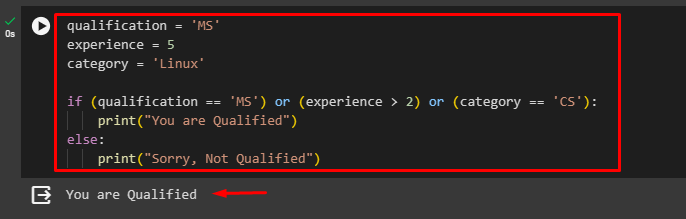
Case 5: Using “or” Operator with “and” Operator
Python also enables users to use two logical operators together. We can use the “or” $ “and” operators together in a single statement. “or” has a lower precedence than the “and” so it is assessed after the “and” operator in an expression. However, users can use brackets to change the order of evaluation.
In the below code, we have initialized some variables i.e. “day”, “weather” and “time” and assigned them some values. Then, we are using the “or” and “and” operator with different conditions in the “if” statement. We have also used brackets to specify the order for evaluating conditions. The condition indicates either the “day” is “Sunday” or “weather” is “good” and the “time” is “morning”, it will return a “True” output, and the “if” block will be executed. Otherwise, it will execute the “else” block:
day = 'Sunday'
weather = 'bad'
time = 'evening'
if (day=='Sunday' or weather=='good') and time=='morning':
print('Enjoy your weekend!')
else:
print('Go to work')
Since the conditions in the if statement are not satisfied because the “day” is “Sunday”, but “weather” is not “good”, and “time” is not “morning”, it goes to the else block and prints “Go to work”:
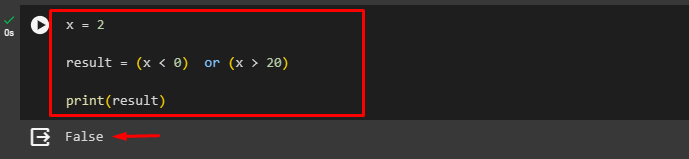
Note: Must use the brackets to specify the operation’s order while using the “or” and “and” operators together to avoid confusion.
Bitwise OR “|” Operator in Python
The bitwise OR “|” is a binary operator that accomplishes a bitwise OR operation/calculation on each bit of two integers. It performs comparison on each first operand’s bit to the corresponding second operand’s bit and retrieves “1” if at least one of the bits is “1” otherwise it retrieves “0”.
The bitwise OR “|” operator first converts the value of specified operands into binary bits and then performs the bit-by-bit operation. After that, it converts the binary number into decimal and returns it as the output.
Truth Table for Bitwise OR “|” Operator
Input | Output | |
Bit 1 | Bit 2 | Bit 1 | Bit 2 |
1 | 1 | 1 |
1 | 0 | 1 |
0 | 1 | 1 |
0 | 0 | 0 |
Example
In this example, we have specified the values of “a” and “b” and used the bitwise OR “|” operator with them. This will first convert the value of “a” and “b” into binary bits and then perform the bit-by-bit operation. After that, it will convert the binary number into decimal and return it as the output:
a = 6
b = 3
res = a | b
print(res)
The output using the bitwise OR “|” operator can be seen below:
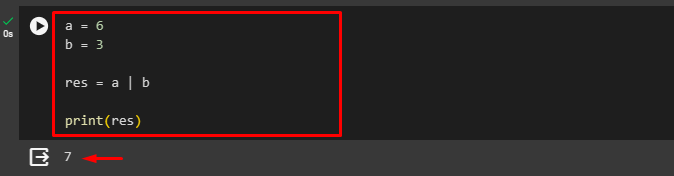
The binary values of the above numbers are:
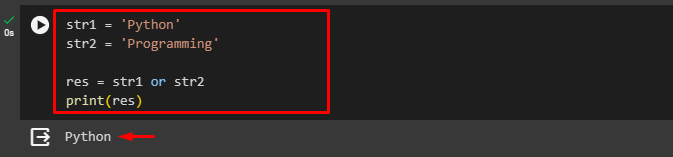
Performing bitwise OR “|”:
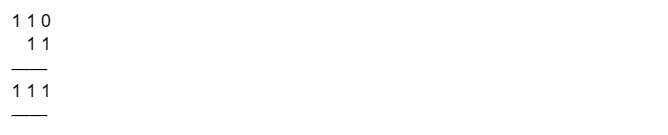
The decimal value of 111:

Comparison Between Logical “or” Operator and Bitwise OR “|” Operator in Python
The logical “or” operator is used to combine and test conditions. It returns “True” when either or both conditions are True and otherwise it returns “False”. In contrast, the bitwise OR “|” operator performs a binary operation on the operands’ bits. It compares the bits of two integers and sets the result bit to “1” if at least one of the equivalent/corresponding bits is 1, otherwise, it retrieves “0”.
Example
Here, we have initialized a couple of variables with some values. Then, we applied the logical “or” and bitwise OR “|” operations on them and displayed the results:
num1 = 5num2 = 9
res1 = num1 or num2res2 = num1 | num2
print(res1)print(res2)
The logical “or” has returned the first value i.e. “5” while the bitwise OR “|” operator has returned the value “13”:
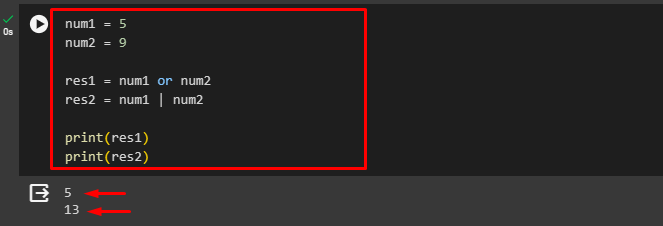
Note: Click on the provided link to access our Google Colab Notebook.
Conclusion
In a nutshell, the “or” operator is one of the logical operators that test conditions and check whether any of two conditions is True. It yields “True” when either or both conditions are True, else, it retrieves “False”. This blog has illustrated everything about the “or” operator in Python.