The foreach loop/method was first introduced by Java 5. This method was used to iterate over arrays as in the case of loops it is a while loop and the do-while loop. Unfortunately, there is no such foreach keyword method in Python 3.0 but we can alternatively use this method in many ways. In this article, we will be learning about how the foreach function works and how is it used. So let’s get started.
Understanding the ‘Foreach’ Function in Python 3
In Python, there isn’t a specifically designated keyword as “foreach” loop the functioning of each loop is the same as the For-loop in Python. So we write the syntax of foreach loop as follows:
for items in iterable:
operate(items)
In the above syntax:
- The for loop iterates through every item in the iterable structure.
- On every iteration, the operator, that is defined in the next statement as “operate”, is operated on the items. This operator can be a print statement which means that the print statement will print the items in all the iterations.
Let’s see this using an example.
Example: Foreach loop using for loop in python
Let’s consider the below code as an example of using the foreach loop using the for loop in Python.
myArr = [15, 16, 17]
for i in range(len(myArr)):
print("value: %s" % (myArr[i]))
In the above code:
- We have declared an array first.
- We have iterated a for loop over the array length.
- We have printed all the values present in the array.
The output of the program is:
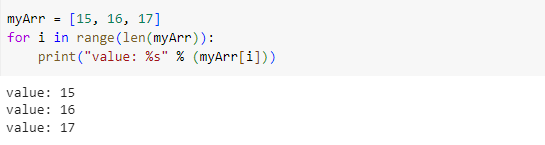
In this way, we can use the for loop doing the same function as foreach loop.
There is another way we can have our Foreach loop which is a user-defined loop.
User-Defined Foreach Loop
The user can define a foreach loop for itself. This foreach loop can look like this:
def foreach(function, iterable):
for eachItem in iterable:
function(eachItem)
In the above syntax:
- The custom/user-defined foreach loop contains 2 arguments.
- The first argument is a function that gives a call back to the “eachitem”.
- The second argument is the “iterable” sequence data on which the iteration and the callback are going to be performed.
Conclusion
In Python, there is no specific keyword as “foreach”. But Python offers some options that work the same as foreach loop. In this article, we have learned about the two ways in which we can use for each loop. that are using For loop as foreach loop and creating the user-defined foreach loop with the help of examples and syntax.