In Python, lists are ordered data structure types that can be modified. As lists can be changed therefore we can apply operations to them. Getting the difference between two lists helps in a multitude of scenarios like carrying out data validation, co-relating and modifying the data. Various method exists that can get you the difference between two lists.
A few ways by which we can get the difference between the two lists are:
- Using set()
- Using in
- Using NumPy
- Using symmetric_differnce()
- Using Union() and Intersection()
- Using set and ^
How to get the difference between two lists with unique entries?
Let us go through each method to learn we can find the difference between two lists:
Method 1: Using set()
The Python function, set(), can be used to get the difference between two lists with unique entries. The lists are converted to a set so they only contain the unique elements. The following code demonstrates how we can get the difference between two lists in Python using set():
list_1 = [23,24,9,45,98,56,34,78,20]
list_2 = [7,9,20,87,98,20,34,78]
y = set(list_2)
diff = [x for x in list_1 if x not in y]
print(diff)
In the above code,
- Two lists list_1 and list_2 are defined and given a value
- y=set(list_2) converts the list_2 into a set stored in the variable y so that no element in the list_2 repeats and it contains only the unique elements
- x is defined to be an element in list_1. Each element in the list_1 is checked if it belongs to y or not. The difference between the two lists is stored in the list named diff in a list comprehension.
- print(diff) displays the result that will show the difference.
Output:
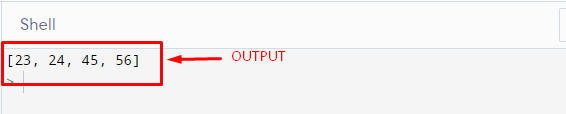
Output With Unique Entries:
Let us consider two lists with unique entries where list_1= [3,4,2,7,6] and list_2=[1,9,5].
The output is:

Method 2: Using in
In this method, in keyword will be used to get the difference between two lists. The following code illustrates how we can get the difference between two lists using in:
list_1 = [23,24,9,45,98,56,34,78,20]
list_2 = [7,9,20,87,98,20,34,78]
diff = []
for x in list_1:
if x not in list_2:
diff.append(x)
print(diff)
In the above code,
- Two lists list_1 and list_2 are defined and given a value
- An empty list diff is defined that will contain the difference of two lists later.
- A for loop is iterated that will for each element x in list_1
- It will return True if any element(x) of list_1 is not included in the list_2. In this case, the elements will be appended to the list diff
- The list diff will be printed using print(diff) representing the difference between the two lists.
Output:

Output With Unique Entries:
Let us consider two lists with unique entries where list_1= [3,4,2,7,6] and list_2=[1,9,5].
The output is:

Method 3: Using NumPy
The difference between the two lists can be found by using the functionalities from the NumPy library. The individual differences between the two lists are found using np.setdiff which is then integrated through np.concatenate. The following code illustrates how we can get the difference between two lists with unique entries:
import numpy as np
list_1 = np.array([23,24,9,45,98,56,34,78,20])
list_2 = np.array([9,20,98,20,34,78])
diff1 = np.setdiff1d(list_1, list_2)
diff2 = np.setdiff1d(list_2, list_1)
diff = np.concatenate((diff1, diff2))
print(list(diff))
In the above code,
- The module numpy is imported as np
- Two NumPy arrays list_1 and list_2 are defined and given a value
- diff1 = np.setdiff1d(list_1, list_2) extracts the unique elements in list_1 that are not in list_2 and store them in the variable diff1
- diff2 = np.setdiff1d(list_2, list_1) extracts the unique elements in list_2 that are not in list_1 and store them in the variable diff2.
- Both the individual differences diff1 and diff2 will be combined using np.concatenate and stored in the variable diff
- print(list(diff)) will print the final difference in the list format.
Output:

Output With Unique Entries:
Let us consider two lists with unique entries where list_1= [3,4,2,7,6] and list_2=[1,9,5].
The output is:

Method 4: Using symmetric_difference()
Another way to get the difference between two lists is by using the symmetric_difference(). Using this function, the elements of list_1 will be returned that are not there in the list_2 and vice versa. The result is calculated in lesser steps, unlike the other methods. Let us look at the following code to see how the symmetric_difference() works:
list_1 = [23,24,9,45,98,56,34,78,20]
list_2 = [9,20,98,20,34,78]
difference = set(list_1).symmetric_difference(set(list_2))
diff = list(difference)
print(diff)
In the above code,
- Two lists list_1 and list_2 are defined and given a value
- Both the lists are converted to sets using the set() function and the symmetric difference between them is stored in a set named difference
- The set “difference” is converted to a list by using the list() function and stored in the variable “diff”
- print(diff) prints diff that is a list having the result of the symmetric difference between the two lists.
Output:

Output With Unique Entries:
Let us consider two lists with unique entries where list_1= [3,4,2,7,6] and list_2=[1,9,5].
The output is:

Method 5: Using set() and XOR Operaror ^
A convenient way to get the difference between the two lists is by using the set function along with the XOR operator ^. Let us look at the following code that gives the difference between two lists using the XOR operator:
list_1 = [23,24,9,45,98,56,34,78,20]
list_2 = [9,20,98,20,34,78]
difference = set(list_1) ^ set(list_2)
diff = list(difference)
print(diff)
In the above code,
- Two lists list_1 and list_2 are defined and given a value
- list_1 and list_2 are converted to sets using the set() function and the symmetric function between them is found through the XOR operator. The resulting symmetric difference is stored in the variable named difference.
- The result is converted to a list using the list() function and stored in the variable diff.
- The result is printed using print(diff) that contains the symmetric difference between the two lists.
Output:

Output With Unique Entries:
Let us consider two lists with unique entries where list_1= [3,4,2,7,6] and list_2=[1,9,5].
The output is:

Method 6: Using Union() and Intersection()
Lastly, we have a rather long method to find the difference between the two lists. Firstly, the union and the intersection of two sets are taken. Then, the result is found though taking the difference between the union and the intersection. Look at the following code to demonstrate how the difference between two lists can be taken through union and intersection:
list_1 = [23,24,9,45,98,56,34,78,20]
list_2 = [9,20,98,20,34,78]
set1= set(list_1)
set2= set(list_2)
union = set1.union(set2)
print('Union:', union)
intersection = set1.intersection(set2)
print('Intersection:', intersection)
# get the differences
difference = union - intersection
print('The difference of two lists is:', difference)
In the above code,
- Two lists list_1 and list_2 are defined and given a value. Both the lists are converted to sets by the set() function to contain only the unique elements
- Union is taken between set1 and set2 and stored in a variable named union. The union of two sets is printed out.
- Similarly, the intersection of the two sets is taken and stored in the variable named intersection. The intersection of the two sets is printed.
- The result is obtained by getting the difference between union and intersection
- The result is printed using the print() function.
Output:
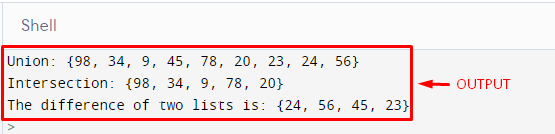
Output With Unique Entries
Let us consider two lists with unique entries where list_1= [3,4,2,7,6] and list_2=[1,9,5]. The output is:
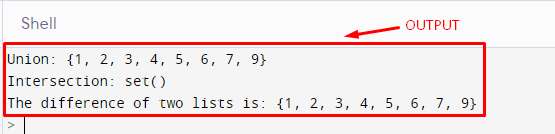
In this article, we have discussed the methods by which we can get the difference between two lists with unique elements.
Conclusion
The difference between two lists in Python can be found by different methods like set(), using the NumPy functionalities, symmetric_difference(), and more methods. Getting the difference between two lists plays an important role in handling the data like altering the data or identifying any list. All of the methods to get the difference between two lists in Python with unique entries are discussed in detail and illustrated with examples.