The List is a collection of data that stores information in a specific order. The unique number is assigned to each List item known as the index number. The single index in the list can store a single object or list of objects. A list that has a nested structure (list in the list) is known as a 2D list or List of Lists.
Consider we have two separate Lists of animals and plants, by inserting these Lists into a new Single List named “Living things”. The structure of this List gets transformed and it is known as a “List of Lists”. This newly created List(List of Lists) doesn’t contain the source List element’s values instead, it copies their references.
Content Overview
How to Create a List of Lists in Java?
- Method 1: Creation of List of Lists Using Java 9 List.of() Method
- Method 2: Create a List of Lists Using Arrays.asList() Method
- Method 3: Create a List of Lists Using add() Method
- Method 4: Create a List of Lists Using Java 8 Stream API Method
How to Iterate Over a List of Lists in Java?
- Method 1: Use of Nested For-each Loop Or Enhanced For Loop
- Method 2: Use of ForEach() Method to Iterate Over the List of Lists
How to Create a List of Lists in Java?
The List of Lists is created by inserting or adding separate lists into a new List containing both List’s elements. This process is done using multiple methods like “List.of()”, “Arrays.asList()”, “add()”, and “collect()”. These methods are explained below with proper implementation:
Method 1: Creation of List of Lists Using Java 9 List.of() Method
The “List.of()” factory method is available in the Java 9 version. It allows users to create an immutable list of Lists having a fixed length. The immutability means that the user can not alter the elements of a List after the creation phase. Moreover, the List of Lists created using the “List.of()” method has a fixed length and does not contain “null” as its element:
import java.util.*;
class ListOfList {
public static void main(String args[]) {
//Declaring String, Integer, and Double Type ArrayLists
List<String> strList = List.of("Arsenal", "Liverpool", "Chelsea");
List<Integer> intList = List.of(68, 12, 23);
List<Double> doubleList = List.of(0.7, 29.3, 77.1);
//List of Lists Creation
List<List<? extends Object>> parentList = List.of(strList, intList, doubleList);
System.out.println("Creation of List of Lists Via Java 9 List.of() Method: \n" + parentList.toString());
}
}
The explanation of the above code is mentioned below:
- First, create three “String”, “Integer”, and “Double” type Lists named “strList”, “intList”, and “doubleList” using the “List.of()” method.
- Then, create a fourth List with a dynamic type named “parentList” and initialize it with the
- Initialize it with the “List.of()” method. Now, pass all three “strList, intList, and doubleList” created Lists as this method arguments to create a List of Lists.
- In the end, apply the “toString()” method over the “parentList” and display the returned result on the console.
The below output authorizes the creation of a List of Lists in Java using the List.of() method:
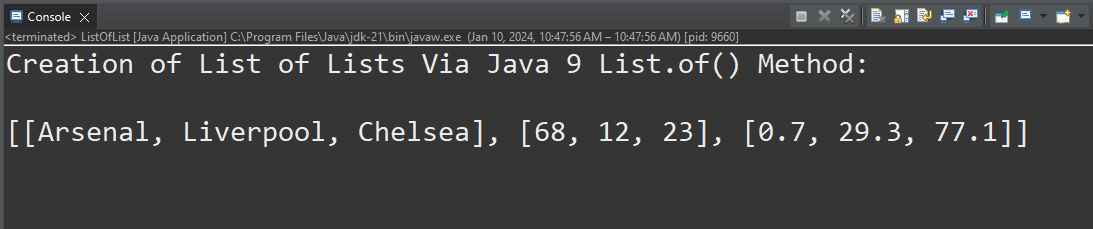
Method 2: Create a List of Lists Using Arrays.asList() Method
The “Arrays.asList()” method allows the users to create a “List of Lists” in Java similar to the “List.of()” method. This method generates a mutable List whose values can be altered at any phase of the program but the Structure of a List remains unchanged. Pass the elements to be inserted in the List as the “Arrays.asList()” method arguments. Also, use the comma “,” token to separate each element. Let’s proceed to the creation of a List of Lists in Java:
import java.util.*;
class ListOfList {
public static void main(String args[]) {//Defining String, Integer, and Double Type ArrayLists
List<String> strList = Arrays.asList("Arsenal", "Liverpool", "Chelsea");
List<Integer> intList = Arrays.asList(68, 12, 23);
List<Double> doubleList = Arrays.asList(0.7, 29.3, 77.1);
//List of Lists Creation List<List<? extends Object>> parentList = Arrays.asList(strList, intList, doubleList);
System.out.println("Creation of a List of Lists Via Arrays.asList() Method: \n\n" + parentList.toString());
}
}
The working of the above code is as follows:
- Initially, define three different types of Lists named “strList”, “intList”, and “doubleList” via the “Arrays.asList()” method.
- Next, pass these created Lists in the “Arrays.asList()” method to create a new List named “parentList”. This List is considered a List of Lists because it is created by combining Lists
- In the end, apply the “toString()” method over the “parentList” and display the returned result on the console.
The generated output confirms the creation of a List of Lists using the “Arrays.asList() method in Java:
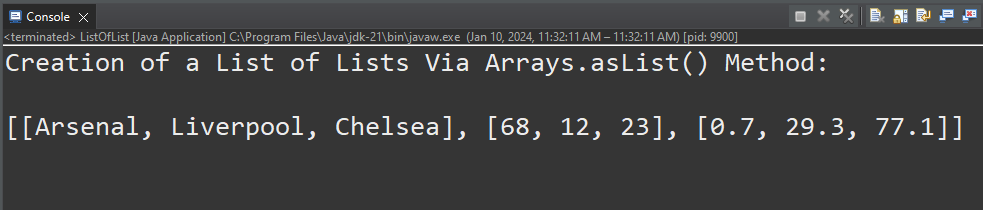
Method 3: Create a List of Lists Using add() Method
The “add()” allows users to insert a single element or a collection into the selected collection like List. By inserting the “List” as an element for another “List”, the List of List gets created. It consumes more lines of code because it can only insert one element or collection at a time. So, if you want to insert “50” Lists inside a single List element then you have to invoke the “add()” method “50” times:
import java.util.*;
class ListOfList {
public static void main(String args[]) {//Declaring String, Integer, Double, and Random Type ArrayLists
List<String> strList = new ArrayList<>();
List<Integer> intList = new ArrayList<>();
List<Double> doubleList = new ArrayList<>();
List<List<? extends Object>> parentList = new ArrayList<>();
strList.add("Hello");
strList.add("Liverpool");
strList.add("Chelsea");
intList.add(13);
intList.add(45);
intList.add(87);
doubleList.add(17.3); doubleList.add(45.8);
doubleList.add(8.73);
//List of Lists Creation
parentList.add(doubleList);
parentList.add(intList);
parentList.add(strList);
System.out.println("Creation of a List of Lists Via add() Method: \n\n" + parentList.toString());
}
}
The working of the mentioned code is as follows:
- First, declare four ArrayLists named “strList”, “intList”, “doubleList”, and “parentList”. The data types of these created Lists are String, integer, double, and unspecified/dynamic.
- Now, insert dummy values in the “strList”, “intList”, and “doubleList” Lists using the “add()” method.
- After that, insert the initialized three Lists into the “parentList” using the “add()” method separately to create a List of Lists.
- In the end, display the “parentList” List on the console after applying the “toString()” method.
The result confirms the creation of a List of Lists using the “add()” method in Java:
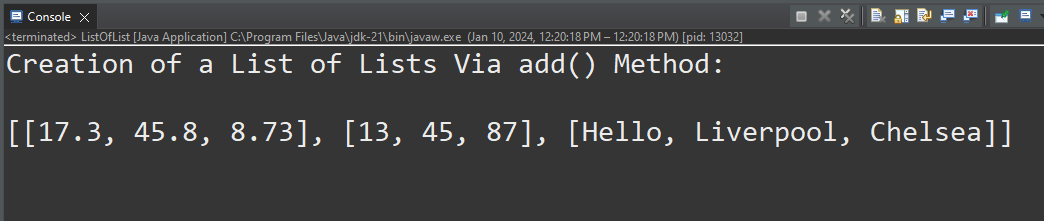
Method 4: Create a List of Lists Using Java 8 Stream API Method
The Java 8 Stream API uses the “Stream.of()” and “collect()” methods to create a List of Lists. The “Stream.of()” method selects all provided Lists and the “collect()” method transforms them into a single newly-created List:
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
class ListOfList {
public static void main(String args[]){//Declaring String, Integer, and Double Type ArrayLists
List<String> strList = new ArrayList<>();
List<Integer> intList = new ArrayList<>();
List<Double> doubleList = new ArrayList<>();
strList.add("Arsenal");
strList.add("Chelsea");
strList.add("Aston Villa");
intList.add(13);
intList.add(45);
intList.add(87);
doubleList.add(17.3);
doubleList.add(45.8);
doubleList.add(8.73);
//List of Lists Creation
List<List<? extends Object>> combineList = Stream.of(strList, intList, doubleList).collect(Collectors.toList());
System.out.println("Creation of a List of Lists Via Java 8 Stream API: \n\n" + combineList.toString());
}
}
Internal working of the above code is shown below:
- Initially, declare and initialize three targeted Lists named “strList”, “intList”, and “doubleList”.
- Then, pass these lists into the “Stream.of()” method and apply the “collect()” with the generated Stream. Also, pass the “Collectors.toList()” as an argument for the “collect()” method to transform the provided Lists into a single List.
- Store the generated result in a new dynamic type List named “combineList”.
- Finally, display this List on the console after passing through the “toString()” method.
The generated output shows the creation of a List of Lists in Java:
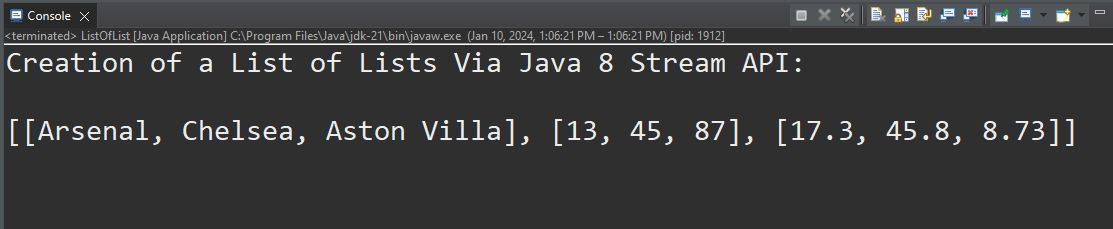
How to Iterate Over a List of Lists in Java?
After the creation of a List of Lists, the user may want to perform specific operations over the elements or want to display them on the console. To perform these and other tasks the user must iterate over the List of Lists elements. For iteration over the List of Lists, Java provides multiple approaches which are being demonstrated below.
To enhance the understanding, the List of Lists named “combineList” created in the above methods is going to be iterated.
Method 1: Use of Nested For-each Loop Or Enhanced For Loop
The user can iterate over the List of Lists elements using the “For-each” loop or “Enhanced for loop” in the nested structure. The outer loop deals with the parent List and the inner loop traverses the element of each child List of the List of Lists:
System.out.println("[");
for(List<? extends Object> parentItem : combineList) {
for(Object childItem: parentItem) {
System.out.println(" => " + childItem);
}
}
System.out.println("]");
In the above code snippet:
- First, apply the “enhanced for loop” over the “combineList” List of Lists and store each element in “parentItem”.
- Set the data type of “parentItem” to dynamic because our List of Lists contains the Lists of multiple data types.
- Then, utilize nested “enhanced for loop” to iterate over each child List. Finally, display the child values along with a dummy token or text as desired.
The output shows that data for the provided List of Lists is retrieved after iteration:
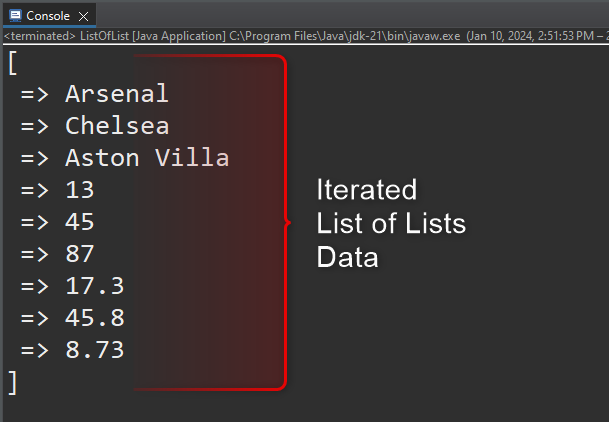
Method 2: Use of ForEach() Method to Iterate Over the List of Lists
The “forEach()” method iterated over all elements of the provided collection until the ending of elements or the occurrence of an exception:
System.out.println("[");
combineList.forEach(
(List<? extends Object> innerList) ->
innerList.forEach((Object item) -> System.out.println(" -> " + item))
);
System.out.println("]");
}
}
In the above code block, two “forEach()” methods are used to iterate over the parent and child List of Lists elements. Moreover, a dummy custom token is also utilized that prints before each List of Lists element.
The generated output shows the retrieved elements after iterating over the List of Lists:
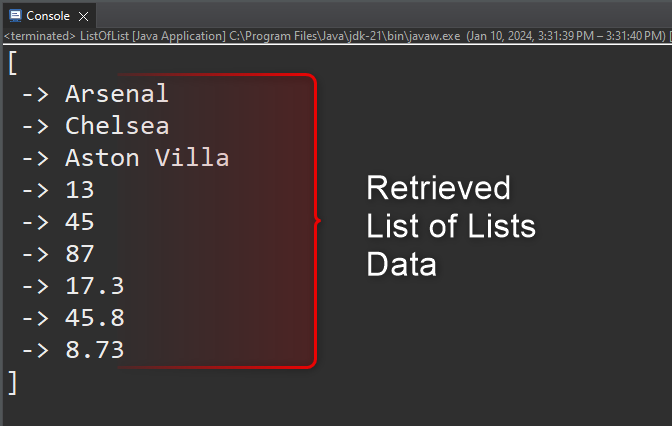
That’s all about the working of a List of Lists in Java.
Conclusion
A List of Lists stores the Lists as the element of another List in the natural order. The List of Lists is created using several methods like “List.of()”, “Array.asList()”, “add()”, and Stream API. The user can also iterate over the List of Lists to extract elements residing at specific indexes or to display all elements. This iteration is performed using the “enhanced for loop” or “forEach()” method. This guide has demonstrated the working of a List of Lists in Java.