In Java, two arrays are compared to check whether both are equal or not. Both arrays are equal if they belong to the same data type, contain an equal number of items, and the values of both array items are the same. In addition, the order of each containing item should be the same or if both items are empty or null. This comparison is beneficial before merging multiple arrays or to identify the redundancy of data in multiple arrays.
This guide explains the concept of comparing two arrays in Java.
How to Compare Two Arrays in Java?
There are a couple of built-in methods namely “equals()” and “deepEquals()” which return true if both arrays are equal or else return false. Along with it, the “==” operator is also useful for comparison purposes as it compares the references of both arrays and their items without considering the content. Let’s proceed to the implementation of these methods.
Method 1: Compare two Arrays Using the “deepEquals()” Method in Java
The “deepEquals()” method of the “Arrays” class allows the user to perform deep comparisons of provided arrays. It provides accurate results even for the nested or multi-dimensional arrays. This method recursively compares the value/content of both array elements to provide the most accurate result:
import java.util.Arrays;
public class CompareArrays {
public static void main(String[] args)
{
String[] array1 = { "I", "J", "K" };
String[] array2 = { "I", "J", "K" };
System.out.println("Comparing 1-D Array Using equals() Method => " + Arrays.deepEquals(array1, array2));
String[][] array3 =
{
{"I", "J", "K"},
{"A", "S", "D"}
};
String[][] array4 =
{
{"I", "J", "K"},
{"A", "S", "D"}
};
System.out.println("Comparing Multi-D Array Using equals() Method => " + Arrays.deepEquals(array3, array4));
}
}
The working of the above code is as follows:
- First, create two one-dimensional arrays with the same random values and pass them into the “deepEquals()” method. This method then compares the values and order of both arrays for the generation of comparison results.
- In the same manner, create and pass two multi-dimensional arrays to check the working of the “deepEquals()” method.
The output confirms that the “deepEquals()” method performs correct comparison for both single and multi-dimensional arrays:
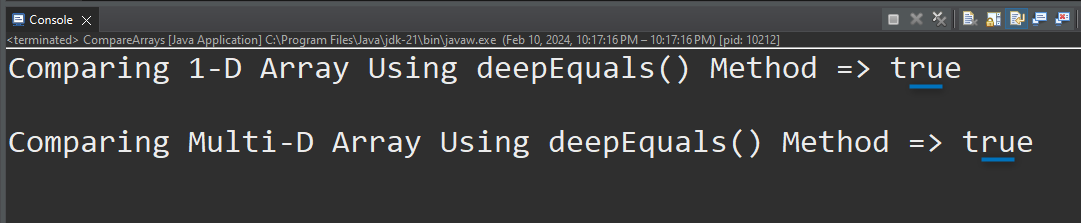
Method 2: Compare two Arrays Using the “equals()” Method in Java
The “equals()” method compares the contents of elements residing at the same index position of both arrays and generates a boolean result. If the content of both array elements are equal then the value of “true” is returned as a result.
It is not recommended to compare nested arrays using the “equals()” method because it does not perform a deep comparison of array elements. However, for a single-level or 1-dimensional array, it returns the correct result, as shown below:
import java.util.Arrays;
public class CompareArrays {
public static void main(String[] args)
{
String[] array1 = { "I", "J", "K" };
String[] array2 = { "I", "J", "K" };
String[][] array3 =
{
{"I", "J", "K"},
{"A", "S", "D"}
};
String[][] array4 =
{
{"I", "J", "K"},
{"A", "S", "D"}
};
System.out.println("Comparing 1-D Array Using equals() Method => " + Arrays.equals(array1, array2));
System.out.println("Comparing Multi-D Array Using equals() Method => " + Arrays.equals(array3, array4));
}
}
In the above code block:
- First, create two “One Dimensional” and two “Two Dimensional” arrays having random data items.
- Next, compare the items of both One-dimensional and Two-dimensional arrays separately using the “equals()” method.
Output confirms that the equals() method performs correct comparison for one-dimensional array only and false for the multi-dimensional array:
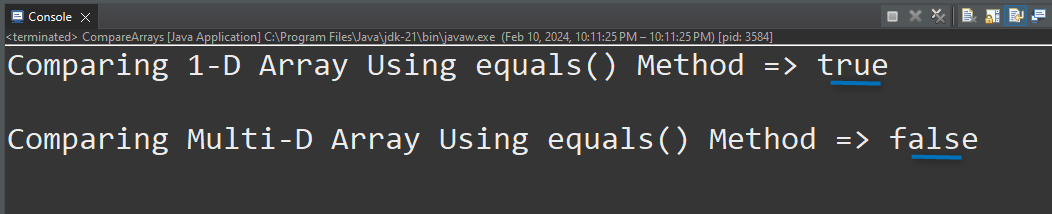
Method 3: Compare two Arrays Using the “==” Method in Java
The “==” operator is another option to perform the comparison of provided arrays. It performs the referential equality and returns true if both operands belong to the same parent class or type and contain the same value. It is utilized along the “for” loops to compare each residing element of an Array and nested “for” loop to compare multi-dimensional arrays. For instance, have a look at the code block below:
public static boolean compareOneDimArray(String[] Array1, String[] Array2)
{
if (Array1 == Array2) {
return true;
}
if (Array1 == null || Array2 == null) {
return false;
}
if (Array1.length != Array2.length) {
return false;
}
for (int i = 0; i < Array1.length; i++) {
if (!Array1[i].equals(Array2[i])) {
return false;
}
}
return true;
}
The above code block compares one-dimensional arrays and its code explanation is shown below:
- First, utilize the “if” statement along with the “==” operator that checks the reference of both provided arrays. In the case of the same reference the value of “true” is returned.
- Next, use the “==” operator to check the nullability of both arrays and check if the length of both is not equal to each other.
- After that, utilize the “for” loop that iterates the length of an array. Inside it, compare the values of both array elements according to their indexes. Also, return the value of “true” if the elements are not equal.
public static boolean compareMultiDimArray(String[][] Array1, String[][] Array2)
{
if (Array1 == Array2) {
return true;
}
if (Array1 == null || Array2 == null) {
return false;
}
if (Array1.length != Array2.length) {
return false;
}
for (int i = 0; i < Array1.length; i++)
{
if (Array1[i].length != Array2[i].length) {
return false;
}
for (int j = 0; j < Array1[i].length; j++)
{
if (!Array1[i][j].equals(Array2[i][j])) {
return false;
}
}
}
return true;
}
The above function compares multi-dimensional arrays and its working is stated below:
- First, compare the reference, nullability, and length of both arrays using the “==” operator.
- Then, utilize the “for” that traverses over the main array and inside it check the length of nested array elements.
- In addition, compare each nested array element using the “equals()” method just like in the above code block.
public static void main(String[] args)
{
String[] Array1 = { "A", "B", "C" };
String[] Array2 = { "A", "B", "C" };
if (compareOneDimArray(Array1, Array2)) {
System.out.println("Both One Dimensional Arrays are Equal");
}
else {
System.out.println("Both One Dimensional Arrays are Not Equal");
}
String[][] Array3 = { {"A", "B", "C"}, {"X", "Y", "Z"} };
String[][] Array4 = { {"A", "B", "C"}, {"X", "Y", "Z"} };
if (compareMultiDimArray(Array3, Array4)) {
System.out.println("\nBoth Multi-Dimensional Arrays are Equal");
}
else {
System.out.println("\nBoth Multi-Dimensional Arrays are Not Equal");
}
- Now, define a main() method, and inside it declare and initialize a couple of one and multi-dimensional arrays.
- Finally, pass these arrays into corresponding “compareOneDimArray(), and “compareMultiDimArray()” methods for comparison.
Output
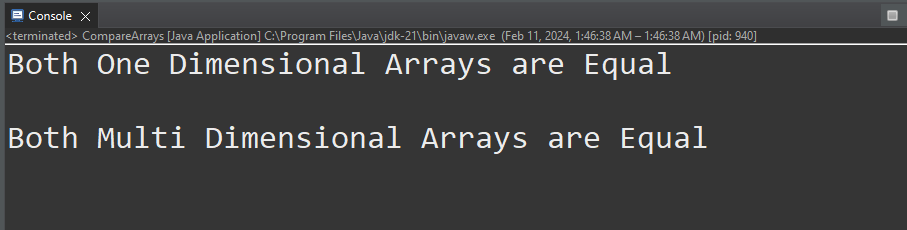
Method 4: Compare two Arrays Using the Java Stream API
To compare arrays residing over the Stream use the methods like “allMatch()” or “anyMatch()”. These methods return true if both arrays contain all elements or some elements respectively. The user can utilize these methods as per their requirements, as shown below:
import java.util.stream.IntStream;
import java.util.Arrays;
public class CompareArrays {
public static boolean compare1DArrays(String[] array1, String[] array2) {
if (array1 == null || array2 == null) {
return false;
}
if (array1.length != array2.length) {
return false;
}
return IntStream.range(0, array1.length).allMatch(i -> array1[i].equals(array2[i]));
}
public static void main(String[] args) {
String[] Array1 = { "A", "B", "C" };
String[] Array2 = { "A", "B", "C", "C"};
String[] Array3 = { "A", "C", "B" };
String[] Array4 = { "A", "B", "C"};
System.out.println("Array Comparison Results:");
System.out.println("\n " + compare1DArrays(Array1, Array2));
System.out.println("\n " + compare1DArrays(Array1, Array3));
System.out.println("\n " + compare1DArrays(Array1, Array4));
}
}
In the above code snippet:
- First, create a function accepting two arrays and utilize the “if” statements to check the nullability of both Arrays. Along with it, compare the length of both arrays.
- Now, compare each element of corresponding positions in both arrays (array1 and array2) for equality using the “equals()” method.
- Moreover, check if all elements are equal in both arrays using the “allMatch()” method and return a boolean value accordingly.
- Lastly, define multiple arrays inside the “main()” method and pass them into the created function “compare1DArrays()” to perform the comparison.
The output displays the result obtained by comparing different arrays with each other:
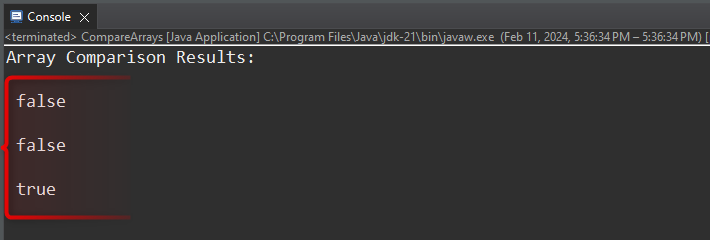
That’s all about comparing two arrays in Java.
Conclusion
In Java, to compare two arrays along with their element’s order use the “equals()” and “deepEquals()” methods, the “==” comparison operator, or the “allMatch()” method of Stream API. The “equals()” method compares each element of both arrays and returns “true” in case of successful matching. It can only compare single-dimensional arrays; to compare multidimensional arrays, you can use the “deepEquals()” method. The “==” operator performs referential comparison and the “equals()” method performs content comparison. Lastly, the “allMatch()” method selects all array elements available over the Stream and then matches them with the elements of a second array. If all elements are available in both arrays at the same index, then the value of “true” is returned.