Prime Numbers are those numbers that are divisible by 1 and itself. In Java, these prime numbers can easily be calculated by using loops, public variables, and custom logic. Implementing the logic for calculating prime numbers in Java is ideal for new developers learning the basics of Java. This article discusses and implements various methods for printing the Prime numbers between 1 to N in Java.
Printing the Prime Number from 1 to N Using a Pre-defined Range (N)
In this code, the prime numbers will be printed from 1 to N using a pre-defined range. For this, an integer variable “range” is declared to calculate the prime numbers between 1 to N:
int range = 100; // defining the range ( 1 to n)
System.out.println("Prime numbers between 1 to "+range+" are ");
The for loop begins iteration from i=2 as “1” and “0” are not prime numbers. For instance, the prime numbers are to be calculated from 2 to 100 (range). In the first iteration, i(2) is less than the range (100). Inside this loop, the int variable “count” is assigned 0. The inner for loop initializes “j” with 1 value. The condition is checked if j(1) is less than i (2). Upon true, the if-statement divides the value of “i” by “j”. In this iteration, the 2 (i) is divided by 1(j) which gives 0 remainder. The count value is incremented by 1:
for(int i=2;i<=range;i++)
{
int count=0;
for(int j=1;j<=i;j++)
{
if(i%j==0)
{
count++;
}
The else-if statement is executed if “i” is not divisible by “j” and the current iteration is skipped. In the second iteration, the value of “j” is 2 which is less than or equal to 2 (i). The condition is true and the i (2) is divided by j (2) which gives 0 as the remainder. The value of the “count” becomes 2. In the third iteration, the value of j (3) exceeds i (2) and the inner for loop terminates. In the outer for loop, the condition in if-statement (count == 2) is checked. The count is only assigned to 2 because prime numbers are only divisible by itself and 1. So the value of “i” is printed (2) which is the prime number. The value of “i” is increment i.e., 3 and the loop continues until i is less than or equal to 100:
else if ( i % j != 0)
{
continue; // if the remainder is non-zero, skip that iteration.
}
}
if(count==2)
System.out.print(i+" ");
}
Output
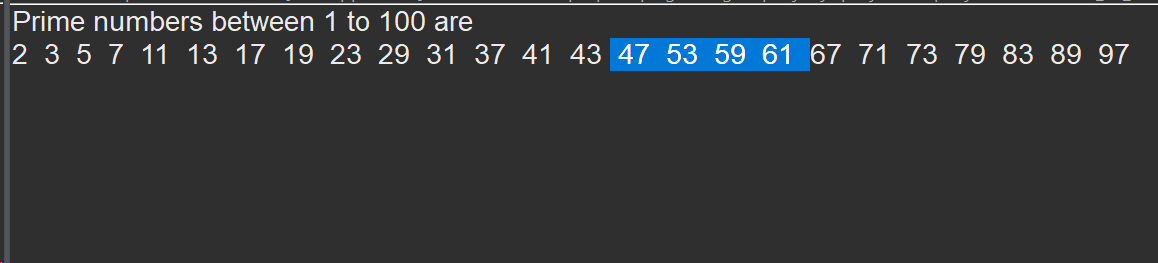
Printing the Prime Number from 1 to N Using the User Input
To print the prime numbers via the user input, include the Scanner class from the java.util package using the following line of code:
import java.util.Scanner; //importing the Scanner class
In the following code, an instance of the Scanner class “input” is created that accepts the standard input via keyboard from the user. The print statement prompts the user to enter the range for calculating the prime numbers. The Scanner object “input” invokes the nextInt() method to read the user input which is then stored in the “range” variable:
Scanner input = new Scanner(System.in); //creating an object of Scanner class
System.out.println("Enter the number till which you want to determine the prime numbers "); // defining the range ( 1 to n)
int range = input.nextInt();
To calculate the prime numbers, the “range” given by the user must be greater than 2 as 0 and 1 are not prime numbers. The if-statement checks if the “range” value is less than 2 and prints the following message using the println() method on the console:
if ( range < 2 )
{
System.out.println("No prime numbers to calclate ");
}
In the else block, the following message is printed on the console. The integer variable “i” is assigned value “2”:
else
{
System.out.println("Prime numbers between 1 to "+range+" are ");
int i =2;
The while loop checks if “i” is less than the range. Upon true, the count and “j” variable of the integer data type are initialized with the following values. The inner while loop will execute unless j exceeds i. The i is the number that is to be checked for prime number and j divides i with every value to determine that. The count value is increment if the condition i % j is satisfied. When the value of count ==2, the value of i is printed which shows the prime numbers. The value of i is increment and the outer loop continues until i is less than or equal to the range:
while ( i <=range)
{
int count=0 , j=1;
while ( j<=i)
{
if( i % j ==0)
{
count= count+1;
}
j++;
}
if( count == 2)
{
System.out.print( i + " ");
}
i= i+1;
}
}
Output
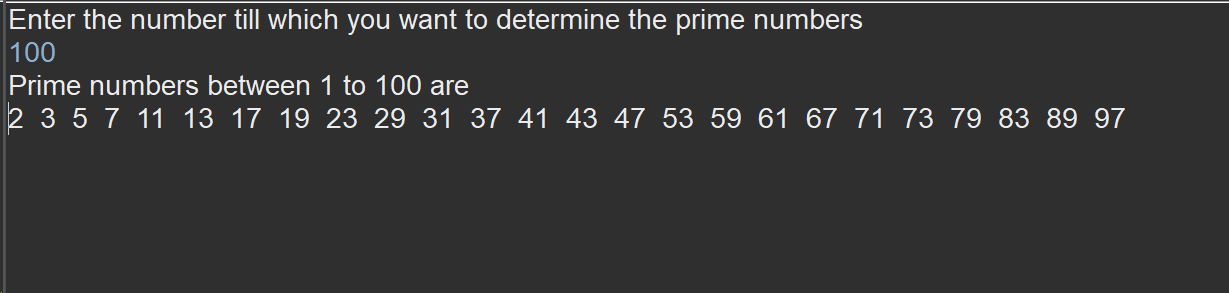
Printing the Prime Numbers Using the Recursion
Recursion is the process of a function calling itself multiple times until the specific condition is met. Developers widely practice recursion that optimizes the code performance. In order to calculate the prime numbers ranging from 1 to N, recursion can be used to simplify the approach as shown in the code below.
Inside the main() method, an integer variable “range” is declared. The print statement displays the message and the for loop begins iterations from “i=2” as 0 and 1 are not prime numbers. Furthermore, this loop determines if the value of “i” lies within the “range”. In the if-statement, recursive calls are made to the isPrime() functions that take the value of “i” as argument. The if-statement prints the prime number if the result returned by the isPrime() is true and vice versa:
public static void main(String[] args) // main method to execute code
{
int range = 100;
System.out.println("Prime numbers from 1 to " + range + " using recursion:");
for (int i = 2; i <= range; i++) {
if (isPrime(i))
{
System.out.print(i + " ");
}
} }
The isPrime() method is a private recursive function that returns true or false as output. Inside this method, the for loop is used to calculate the prime numbers by initializing the value of “i” with “2”. The loop terminates when “i” exceeds the square root of the “num”. This clever approach checks the value of “i” for the factors that make the code optimized by preventing unnecessary iterations. The if-statement within the loop calculates the modulus of “num” and “i”. If the “num” is divisible by the “i” and the remainder is 0, then the output returned is false because the prime number is not divisible by every number. The output returned is true if the num % i is not equal to 0 which means that the number is prime:
private static boolean isPrime(int num) {
for (int i = 2; i <= Math.sqrt(num); i++) {
if (num % i == 0) {
return false;
}
}
return true;
}
Output
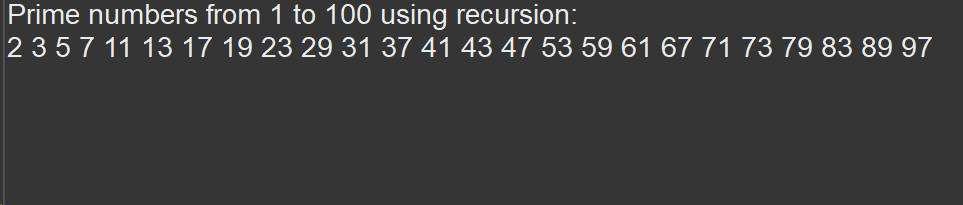
That is all from this article.
Conclusion
Prime numbers play a vital role in programming such as algorithms for random number generation, performance optimization or coding challenges, etc. In Java, printing prime numbers from 1 to N can be achieved by pre-defining the range (N), via the user input or through recursion. For this, public variables, loops such as for loop, while loop, etc are used. This article is a practical walkthrough for printing prime numbers from 1 to N in Java.